Truncate string in Laravel blade templates
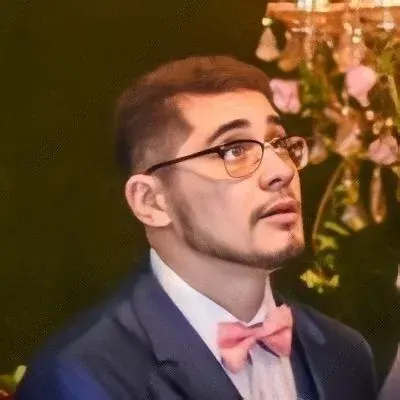
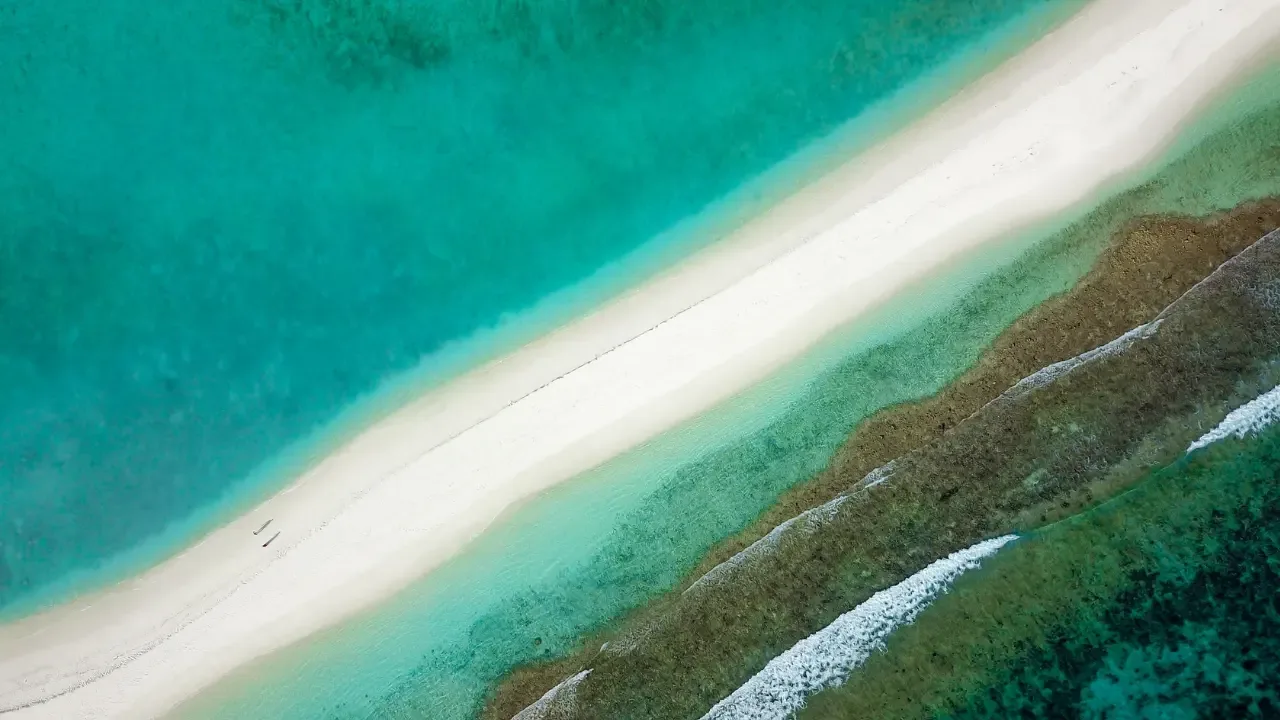
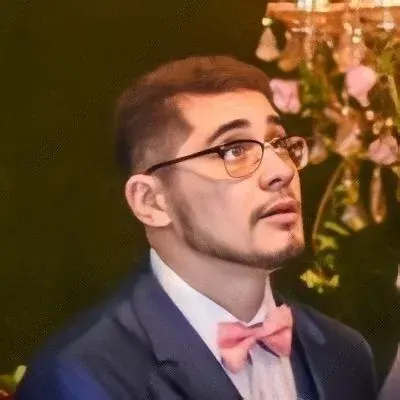
Truncate Strings in Laravel Blade Templates: A Handy Guide π―
Are you tired of dealing with long strings in your Laravel Blade templates? Do you wish there was a simpler way to truncate them without getting tangled in PHP code? π€ Well, you're in luck! In this guide, we will explore how to truncate strings in Laravel Blade templates effectively, without breaking a sweat. πͺ
The Problem π©
So, you want to truncate a string in Laravel Blade templates, like Smarty does, without diving into the PHP debate. π€ The example below is exactly what you're looking for:
{{ $myVariable|truncate:"10":"..." }}
You're hopeful that Laravel has a built-in function to achieve this. π But if not, you're curious if you can create your own reusable modifiers like Smarty provides. You appreciate Blade's simplicity, but you feel that truncating strings would be a real game-changer. π₯ Plus, you're specifically using Laravel 4. π
Easy Solutions π‘
Solution 1: Laravel Str::limit
Laravel has a fantastic Str class with a limit
function built right into it. This function can be used to truncate strings effortlessly. π
Here's how you can use it in your Blade templates:
{{ \Illuminate\Support\Str::limit($myVariable, 10, '...') }}
The limit
function takes three arguments: the string to be truncated, the maximum length, and the suffix to append (e.g., '...'). You'll get the truncated string displayed neatly in your view. βοΈ
Solution 2: Blade Custom Macros
If you find yourself frequently truncating strings and want a reusable solution, Blade macros are here to save the day! π¦ΈββοΈ You can create your own custom macros to extend the Blade templating engine functionality.
First, open your AppServiceProvider.php
file, which is located in the app/Providers
directory. Then, add the following code within the boot
method:
use Illuminate\Support\Str;
public function boot()
{
Str::macro('truncate', function ($value, $length = 100, $suffix = '...') {
return Str::limit($value, $length, $suffix);
});
}
Next, open your Blade template and use your newly created truncate
macro:
{{ Str::truncate($myVariable, 10, '...') }}
VoilΓ ! You have just created your own reusable macro. π Feel free to use it wherever and whenever you need to truncate strings in your views.
Call-to-Action π£
Now that you have learned two easy ways to truncate strings in your Laravel Blade templates, it's time to put that knowledge into action! πͺ Experiment with both solutions and determine which one suits your needs best.
If you found this guide helpful, or if you have any other questions, feel free to leave a comment below. We would love to hear from you and continue helping you in your Laravel journey! π
Don't forget to share this post with your fellow developers who might also be struggling with string truncation in Laravel Blade templates. Sharing is caring, after all! β€οΈ
Happy coding! π