Retrieve Laravel Model results based on multiple ID"s
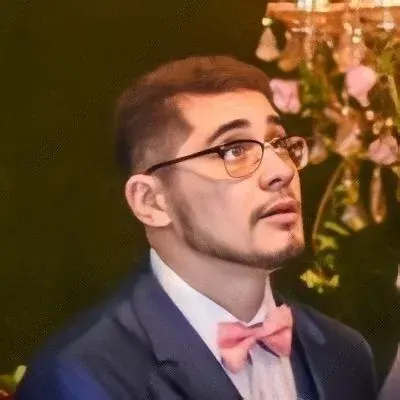
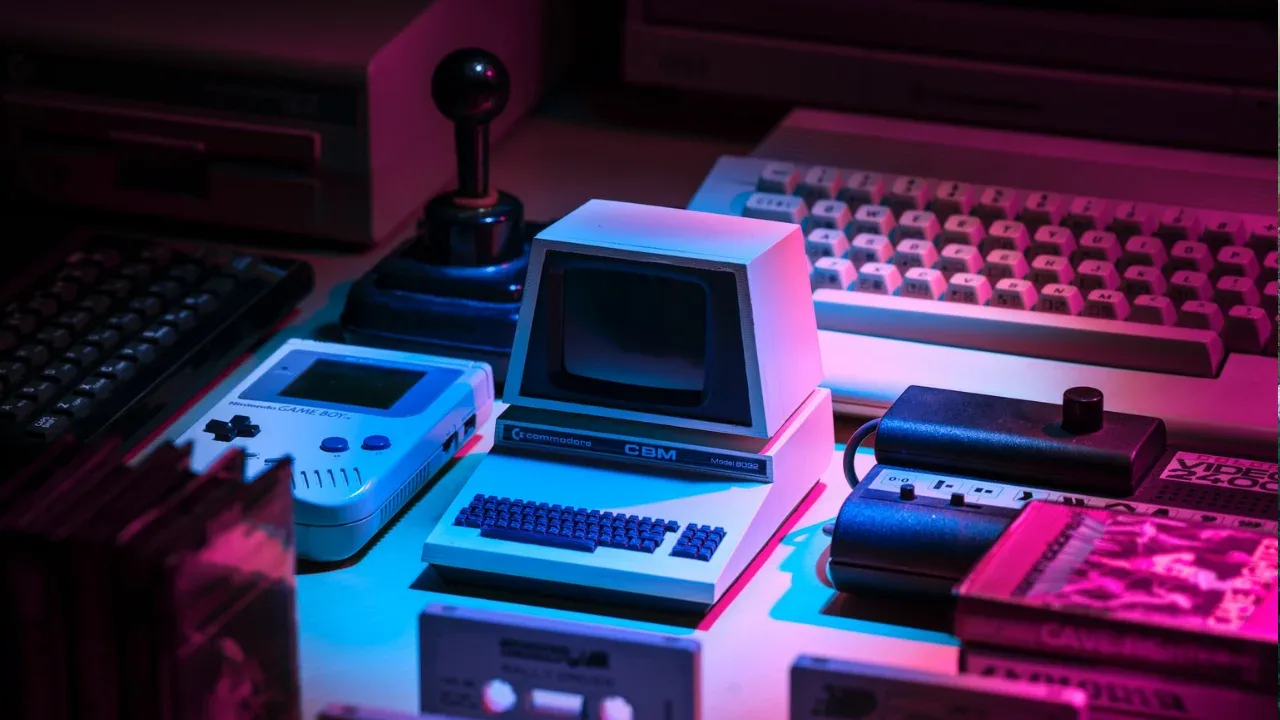
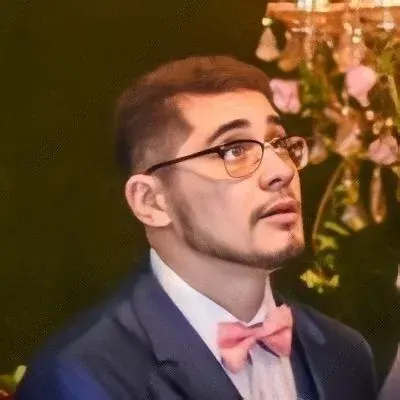
Retrieving Laravel Model Results Based on Multiple IDs
So you have implemented ZendSearch into your Laravel application as a search engine, and it is returning an array of record IDs ordered by relevance. 🧐 However, you want to retrieve the actual record information from your Model based on these ID results.
📚 Don't worry, I've got you covered! In this blog post, I will guide you through the correct way to query your Model and retrieve the results based on the ZendSearch array of IDs. Let's dive in! 💪
The Problem: Retrieving Multiple Model Results from an Array of IDs
When working with Laravel Models, the find()
method allows us to retrieve a single record based on its ID. But how do we retrieve multiple records using an array of IDs and maintain the order specified by ZendSearch? 🤔
The Solution: Using the whereIn()
Method
To retrieve multiple Model results based on an array of IDs, in the specified order, Laravel provides us with the whereIn()
method. This method accepts two arguments: the column to search within and the array of IDs. 🎉
Here's an example of how you can use the whereIn()
method in your case:
$ids = [1, 3, 2, 5]; // Your array of IDs returned by ZendSearch
$results = Model::whereIn('id', $ids)->get();
In the above example, Model
is the name of your Eloquent Model class. Replace it with the actual name of your Model.
The whereIn()
method searches for records where the specified column (id
in this case) matches any of the values within the array of IDs. It returns a collection of Model instances that match the criteria. 😊
The get()
method is then used to retrieve the results as a collection.
Maintaining the Order with orderByRaw()
Now that you have retrieved the Model results using the whereIn()
method, you might want to maintain the order specified by ZendSearch. To achieve this, Laravel provides the orderByRaw()
method. 🚀
You can chain the orderByRaw()
method to the previous query and pass it an SQL raw expression to order the results accordingly. Here's an example:
$results = Model::whereIn('id', $ids)->orderByRaw("FIELD(id, " . implode(',', $ids) . ")")->get();
foreach ($results as $result) {
// Access the record information here
}
In the above example, we use the implode()
function to convert the array of IDs into a comma-separated string. The SQL raw expression FIELD(id, your_comma_separated_ids)
ensures that the results are ordered as per your specified IDs.
Conclusion and Call-to-Action
Congratulations! 🎉 You have successfully retrieved Laravel Model results based on multiple IDs in the specified order. You can now access the desired record information and use it as needed in your application.
If you found this blog post helpful, please consider sharing it with your fellow Laravel developers who might be facing similar challenges. Let's spread the knowledge and make everyone's coding journey smoother! ❤️
If you have any questions or need further assistance, feel free to leave a comment below. I'd be more than happy to help you out.
Keep coding and creating amazing things with Laravel! Happy searching! 🚀✨