Receive JSON POST with PHP
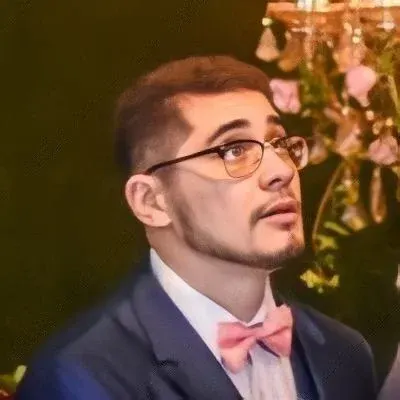
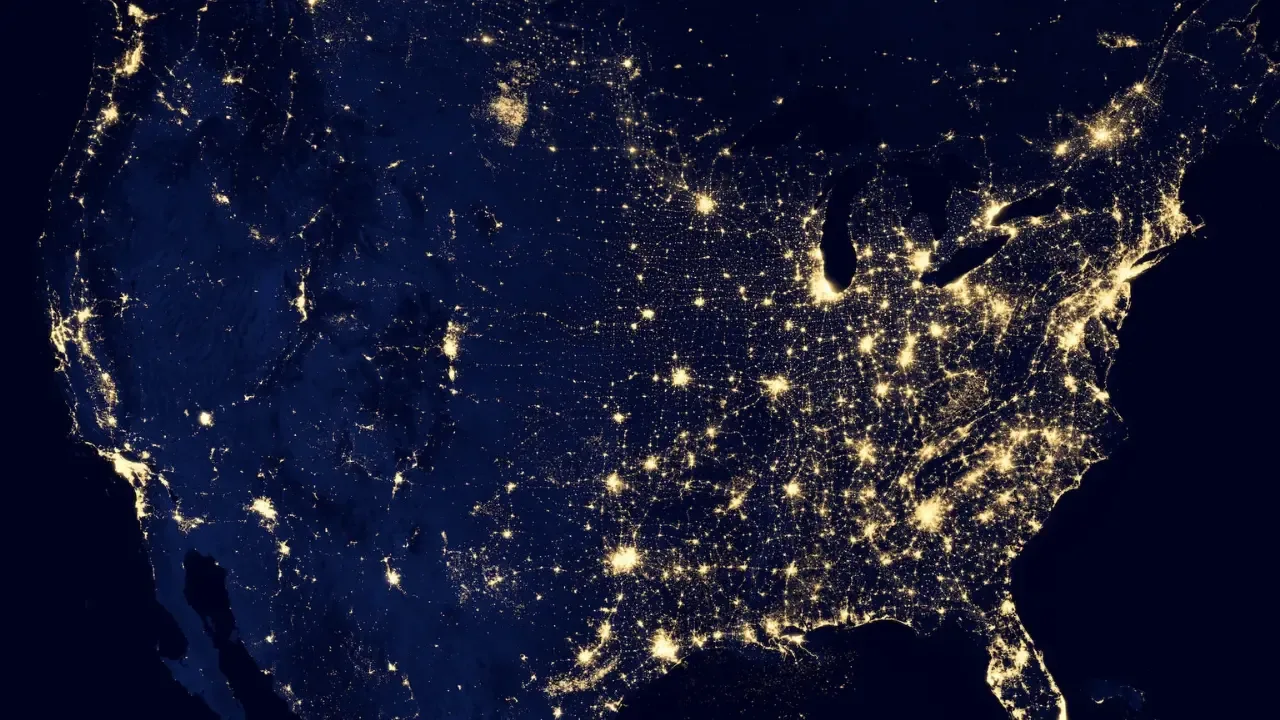
How to Receive JSON POST with PHP: Decoding Issues ⚙️
So, you're trying to receive a JSON POST on your payment interface website, but you're having trouble decoding it. 😩 Don't worry, we're here to help! 🤗 In this blog post, we'll address common issues and provide easy solutions to help you decode that JSON like a pro. 💪
The $_POST Array Dilemma 🤔
You mentioned that when you tried printing $_POST
, you got an empty array. That's definitely a sign that something's not quite right. Let's investigate further. 👀
Solution 1: Loop Over $_POST ➰
To access the values in $_POST
, you can loop over the array using a foreach loop. Simply modify your code as follows:
if ($_POST) {
foreach ($_POST as $key => $value) {
echo "Key: " . $key . " - Value: " . $value . "<br />";
}
}
Now try sending a JSON POST request to your payment interface and see if the values are properly displayed. 📝
Solution 2: JSON Decoding Using $_POST Values 📝
You mentioned attempting to decode the JSON using the operation
key from $_POST
. However, it returned NULL
. To fix this, you can try the following code snippet:
$string = $_POST['operation'];
$var = json_decode($string);
echo $var;
Make sure to check if the operation
key is present in the JSON payload you're receiving. If it's not, you might need to update your code accordingly. 🔄
Solution 3: Utilizing file_get_contents() and json_decode() 📥
If the above solutions didn't work, you can try using file_get_contents('php://input')
to retrieve the raw JSON data from the request body. Here's an example snippet to help you out:
$data = json_decode(file_get_contents('php://input'));
var_dump($data->operation);
Keep in mind that file_get_contents()
returns the entire contents of the file or URL as a string, so you don't need to use json_decode()
with an extra parameter. 🙌
Solution 4: json_decode() with an Additional Flag 🤝
In case the previous solutions still didn't solve your problem, you can try adding an additional flag to json_decode()
to force the decoding of associative arrays. Modify your code like this:
$data = json_decode(file_get_contents('php://input'), true);
var_dump($data);
Now, check if $data
contains the JSON values you're expecting. Hopefully, this should do the trick! 🌟
The JSON Format and Payment Site Documentation 📋
You provided the JSON format according to the payment site documentation, which is incredibly helpful. 🙏 However, it seems like your decoding attempts didn't produce the desired results.
The problem might lie in a discrepancy between the actual JSON received and the JSON format mentioned in the documentation. Double-check that the JSON payload you're receiving matches the provided format exactly, including proper capitalization and key names.
Next Steps: Engage With Us! 📣
If you're still facing issues after trying out the solutions mentioned above, don't hesitate to reach out to us in the comments section below. We'd be more than happy to assist you further. 💬
Additionally, if you've found this blog post helpful, don't forget to share it with your fellow developers who might be grappling with JSON decoding issues. Let's spread the knowledge and make decoding JSON a breeze for everyone! 🌐💡
Happy coding! 🚀✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
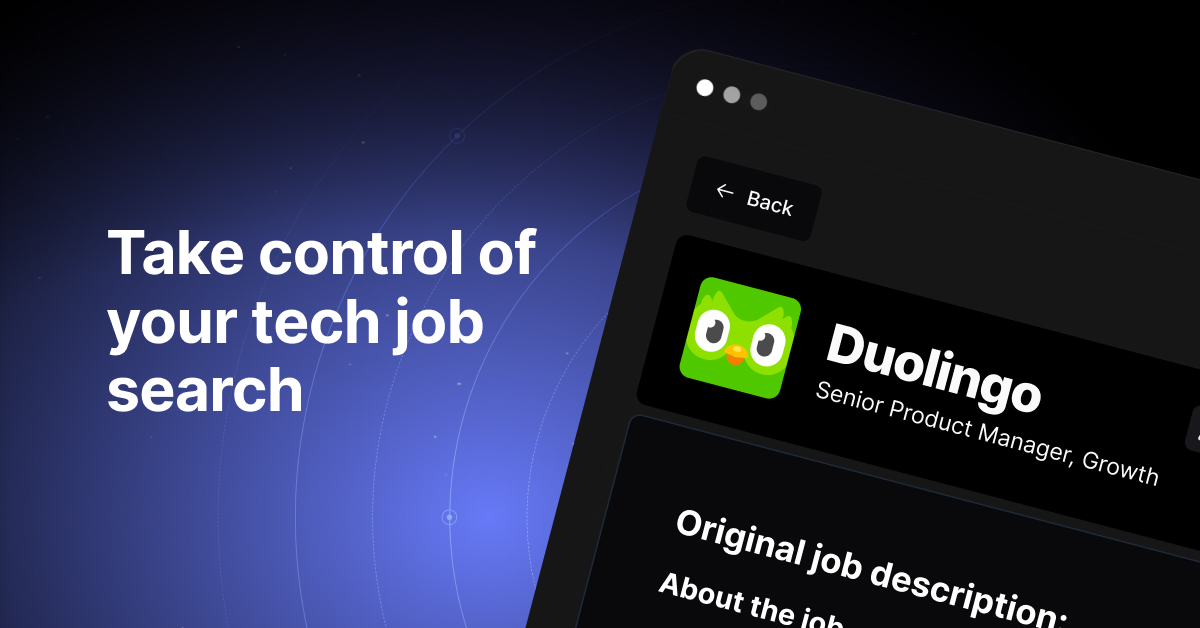