Property [title] does not exist on this collection instance
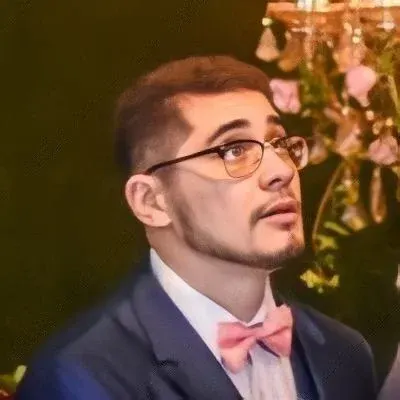
![Cover Image for Property [title] does not exist on this collection instance](https://images.ctfassets.net/4jrcdh2kutbq/3tAoFyOtU79sm9R904NmQv/9f3cdc236da23a8c4cb583429de2b2bc/Untitled_design__11_.webp?w=3840&q=75&fm=webp)
Fixing "Property does not exist on this collection instance" Error in Laravel
Are you facing the "Property does not exist on this collection instance" error while working on a Laravel project? Don't worry, you're not alone. This is a common issue that can occur when trying to access a property on a collection instance in Laravel. In this blog post, we will explore why this error occurs and provide easy solutions to fix it.
Understanding the Error
The error message "Property does not exist on this collection instance" typically occurs when you try to access a property on a collection instead of an individual model instance. In Laravel, the get()
method returns a collection of model instances, while the find()
method returns a single model instance.
In the context of the provided code snippet, the where('page', 'about-me')->get()
method returns a collection of Page
models. When you access the title
property using $about->title
in your view, the error is thrown because $about
is a collection, not an individual model instance.
Solution 1: Accessing Collection Items
To fix the error, you need to access the items within the collection. One way to achieve this is by iterating over the collection using a loop. Modify your view code as follows:
@section('title')
@foreach($about as $page)
{{$page->title}}
@endforeach
@stop
@section('content')
@foreach($about as $page)
{!! $page->content !!}
@endforeach
@stop
By iterating over the collection with a loop, you can access the properties of each individual model instance without triggering the "Property does not exist" error.
Solution 2: Retrieving a Single Model Instance
Alternatively, if you only expect one result from the database query, you can use the first()
method instead of get()
. This will return a single model instance instead of a collection.
Modify your controller code as follows:
public function index()
{
$about = Page::where('page', 'about-me')->first(); // Get a single model instance
return view('about', compact('about'));
}
With this change, you can continue using $about->title
and $about->content
in your view without encountering the error.
Conclusion
The "Property does not exist on this collection instance" error is typically caused by trying to access a property on a collection instead of an individual model instance in Laravel. By following the solutions provided in this blog post, you should be able to fix the error and access the desired properties without any issues.
Remember to choose the solution that best fits your specific use case. If you expect multiple results from the database query, use Solution 1 and iterate over the collection. If you only expect one result, use Solution 2 and retrieve a single model instance.
Now that you know how to fix this error, go ahead and implement the recommended solution in your code. Happy coding! 😊
Have you encountered the "Property does not exist on this collection instance" error in Laravel? How did you fix it? Share your thoughts and experiences in the comments below!
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
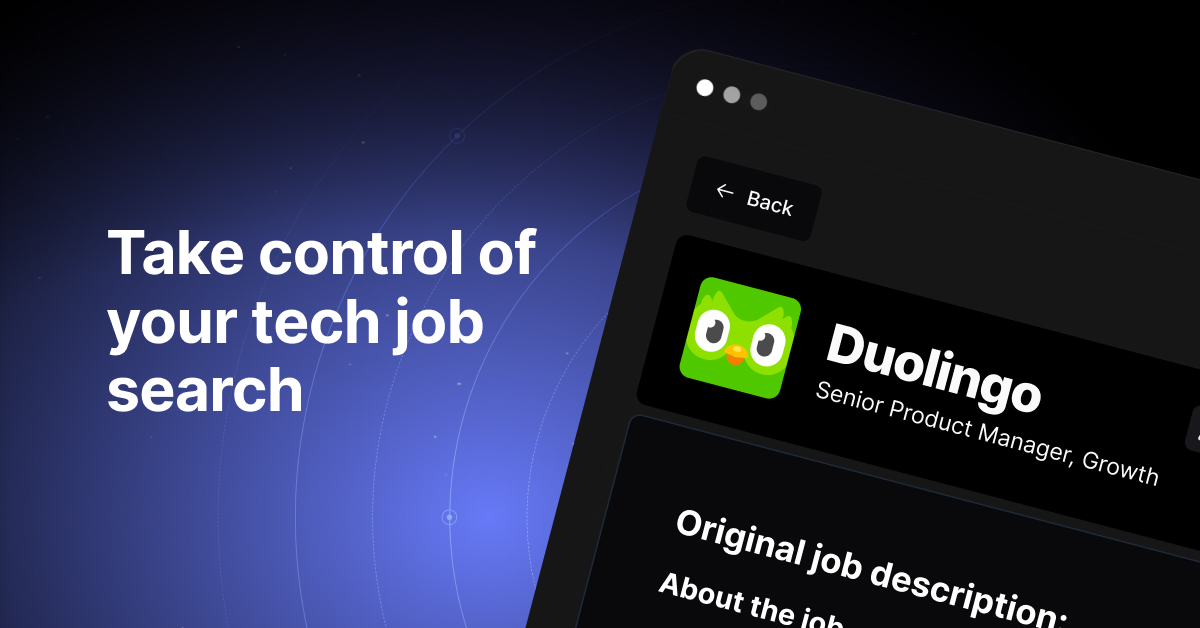