Proper Repository Pattern Design in PHP?
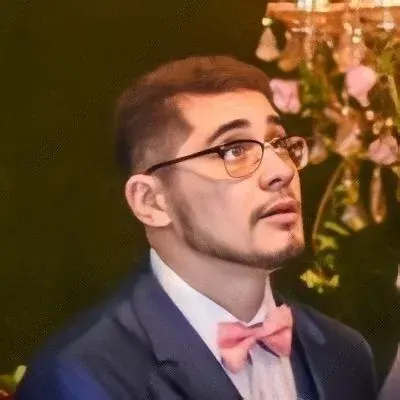
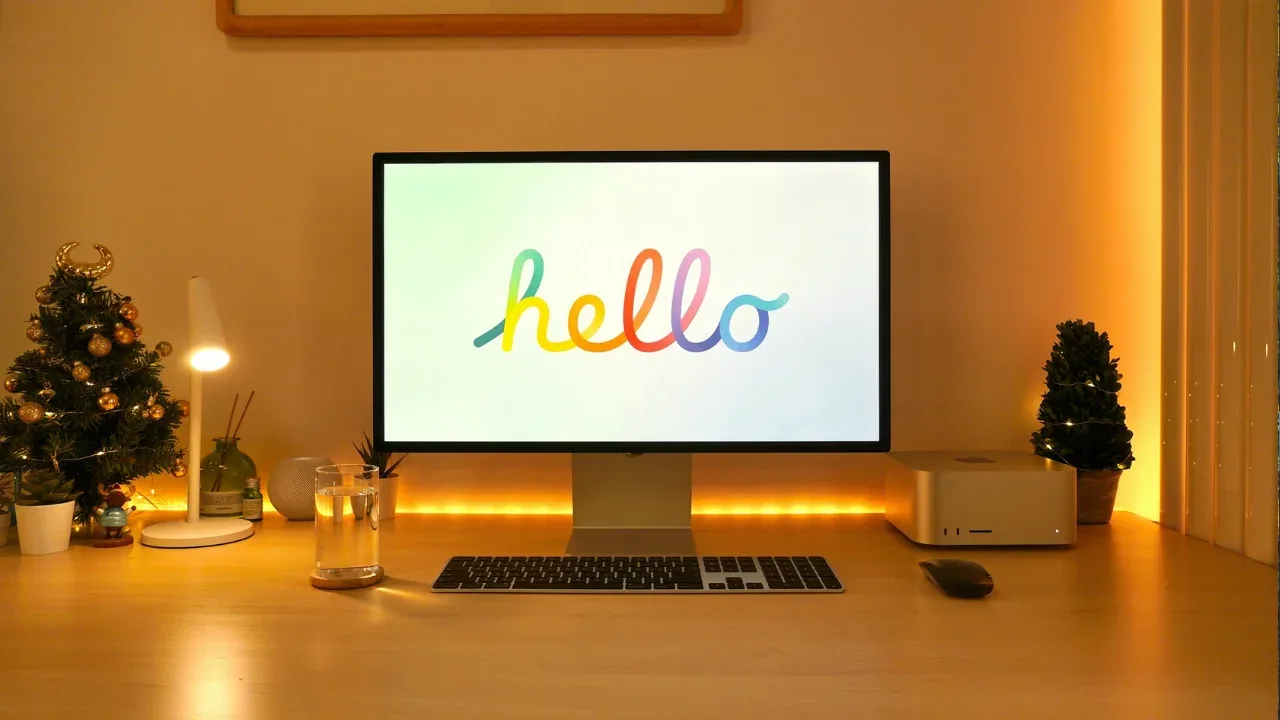
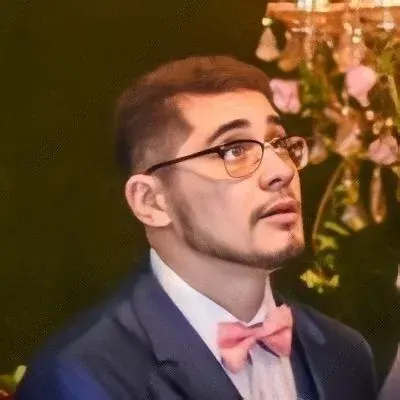
📦 Proper Repository Pattern Design in PHP? - A Practical Guide 🐘💻
<p>So, you've heard about the repository pattern and its potential to decouple your database from the rest of your application in PHP. But now you have some practical questions about repository design? Don't worry, we've got your back! Let's dive into some common issues and provide easy solutions to ensure proper repository pattern design in PHP.</p>
Issue #1: Too many fields 👥📋
The provided DbUserRepository
class uses a select all fields (SELECT *
) approach. But, in a real-world application, loading all fields can add unnecessary overhead and slow things down. So, how can we deal with this issue?
One method to solve this problem is to create value objects that represent the specific data needed. For example, instead of SELECTing all fields, you can create a UserBasicInfo
value object that only includes the name
and email
fields. This way, you can fetch only the required fields, reducing overhead and improving performance.
class UserBasicInfo {
public $name;
public $email;
public function __construct($name, $email) {
$this->name = $name;
$this->email = $email;
}
}
class DbUserRepository implements UserRepositoryInterface
{
protected $db;
public function __construct($db)
{
$this->db = $db;
}
public function findAllBasicInfo()
{
// Fetch only required fields (name, email) and return UserBasicInfo objects
}
}
Issue #2: Too many methods 🚀🚧
As your application grows, you might find yourself in a situation where you need to add more specific methods to your repository. The provided example already addresses this issue by including the basic CRUD methods. But what about more complex queries?
To tackle this, you can leverage the power of query builders or database abstraction libraries, like Eloquent in Laravel. These libraries provide expressive methods to build complex queries without cluttering your repository with countless specific methods.
class MyController
{
public function users()
{
$users = User::select('name', 'email', 'status')
->where('country', 'Canada')
->orderBy('name')
->get();
return View::make('users', ['users' => $users]);
}
}
By utilizing query builders, you can build dynamic queries based on conditions and sort orders, reducing the need for individual repository methods.
Issue #3: Impossible to match an interface 🔄🔀
Interfaces are essential for implementing the repository pattern. They define a contract that an implementation must adhere to. However, adding additional methods to your repositories can create a mismatch between the interface and the implementations.
To solve this, consider using the Specification Pattern. This pattern allows you to encapsulate complex query conditions and reuse them across different repositories. By employing this pattern, you can keep the repositories' interfaces clean while still running specific queries.
Here's an example of how you can use the Specification Pattern:
interface UserRepositoryInterface
{
public function findAllBySpecification(Specification $specification);
}
class UserInCountrySpecification implements Specification
{
protected $country;
public function __construct($country)
{
$this->country = $country;
}
public function isSatisfiedBy(User $user)
{
return $user->country === $this->country;
}
}
class DbUserRepository implements UserRepositoryInterface
{
protected $db;
public function __construct($db)
{
$this->db = $db;
}
public function findAllBySpecification(Specification $specification)
{
// Fetch all users and filter them based on the specification
// Example: return users that match UserInCountrySpecification
}
}
By implementing the Specification Pattern, you can define reusable specifications to query specific subsets of data without bloating your repository interfaces.
📢 Call-To-Action: Share your thoughts! 💌💬
Have you encountered any issues with the repository pattern design in PHP? Do you have any other tips or tricks to share? Leave a comment below and let's spark a discussion! Together, we can learn and improve our PHP repository patterns. Happy coding! 😄🚀