Programmatically creating new order in Woocommerce
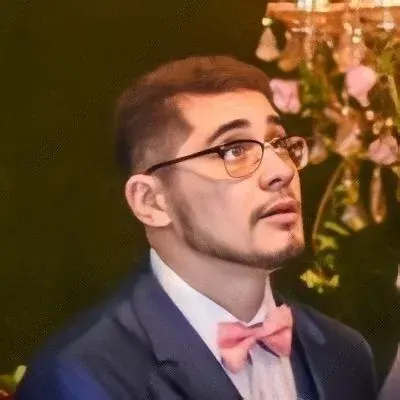
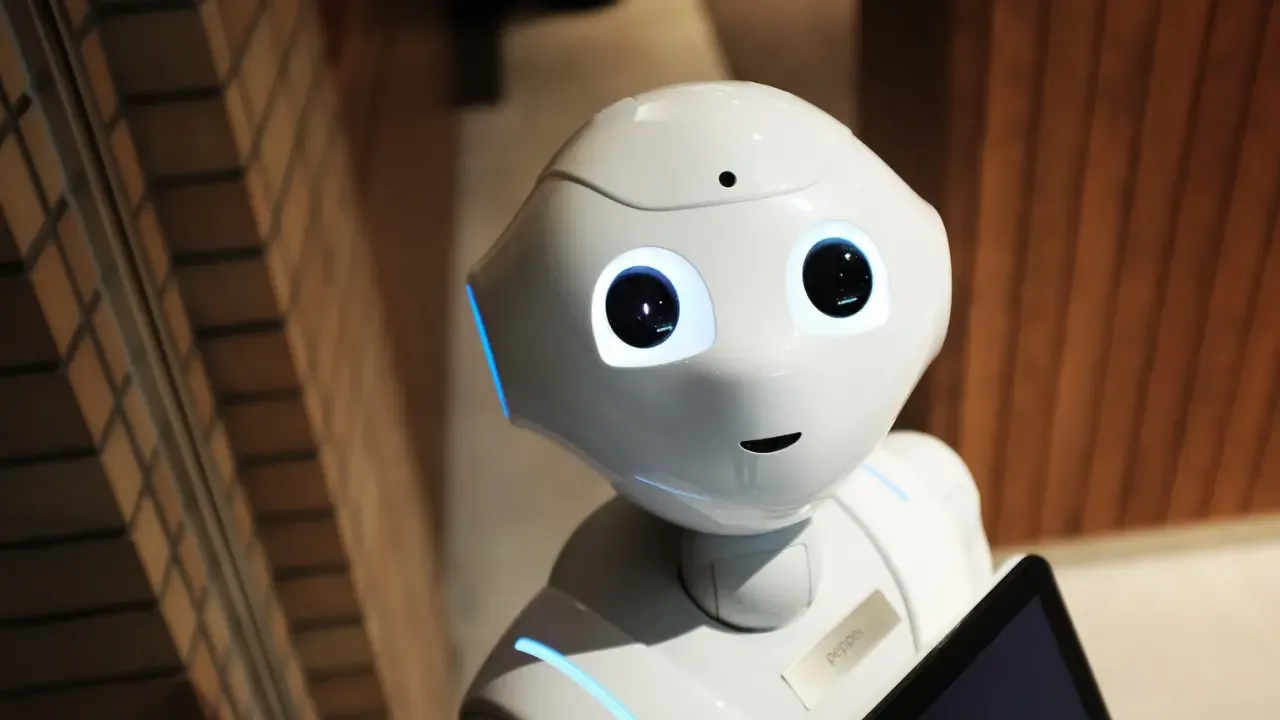
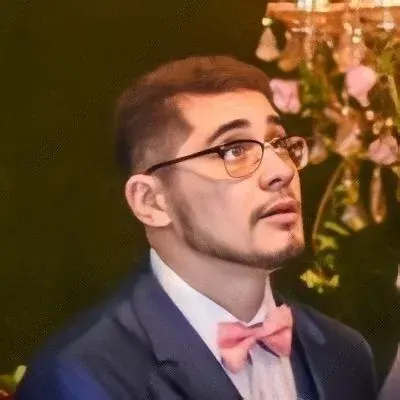
🛒 Creating a New Order in WooCommerce Programmatically
Having trouble programmatically creating an order in WooCommerce? Don't worry, we're here to help you out! 🤓💪
🔍 The Problem
You've tried using the code below to create an order, but you're encountering some issues. The order is created successfully, but there's no customer information or product line items added to it. The order seems to be created as a guest without any details.
function create_vip_order() {
global $woocommerce;
$address = array(
'first_name' => '111Joe',
'last_name' => 'Conlin',
'company' => 'Speed Society',
'email' => 'joe@testing.com',
'phone' => '760-555-1212',
'address_1' => '123 Main st.',
'address_2' => '104',
'city' => 'San Diego',
'state' => 'Ca',
'postcode' => '92121',
'country' => 'US'
);
// Now we create the order
$order = wc_create_order();
// The add_product() function below is located in /plugins/woocommerce/includes/abstracts/abstract_wc_order.php
$order->add_product( get_product( '275962' ), 1 ); // This is an existing SIMPLE product
$order->set_address( $address, 'billing' );
$order->calculate_totals();
$order->update_status("Completed", 'Imported order', TRUE);
}
add_action( 'woocommerce_init', 'create_vip_order' );
🐞 The Error
Here's the error you're getting in your logs:
[19-Apr-2016 21:16:38 UTC] PHP Fatal error: Uncaught Error: Call to a member function add_product() on boolean in /Users/joe/Sites/speedsociety-2/wp-content/themes/ss/lib/contests/order.php:107
Stack trace:
#0 /Users/joe/Sites/speedsociety-2/wp-includes/plugin.php(525): create_vip_order('')
#1 /Users/joe/Sites/speedsociety-2/wp-content/plugins/woocommerce/woocommerce.php(330): do_action('woocommerce_ini...')
#2 /Users/joe/Sites/speedsociety-2/wp-includes/plugin.php(525): WooCommerce->init('')
#3 /Users/joe/Sites/speedsociety-2/wp-settings.php(392): do_action('init')
#4 /Users/joe/Sites/speedsociety-2/wp-config.php(67): require_once('/Users/joe/Site...')
#5 /Users/joe/Sites/speedsociety-2/wp-load.php(37): require_once('/Users/joe/Site...')
#6 /Users/joe/Sites/speedsociety-2/wp-admin/admin.php(31): require_once('/Users/joe/Site...')
#7 /Users/joe/Sites/speedsociety-2/wp-admin/edit.php(10): require_once('/Users/joe/Site...')
#8 {main}
thrown in /Users/joe/Sites/speedsociety-2/wp-content/themes/ss/lib/contests/order.php on line 107
💡 The Solution
To fix this issue, you need to make sure that you're using the correct hook and the right place to create the order. Instead of using the woocommerce_init
hook, you should use the woocommerce_new_order
hook, which is fired after a new order is created.
function create_vip_order( $order_id ) {
$order = wc_get_order( $order_id );
$address = array(
'first_name' => '111Joe',
'last_name' => 'Conlin',
'company' => 'Speed Society',
'email' => 'joe@testing.com',
'phone' => '760-555-1212',
'address_1' => '123 Main st.',
'address_2' => '104',
'city' => 'San Diego',
'state' => 'Ca',
'postcode' => '92121',
'country' => 'US'
);
$order->add_product( get_product( '275962' ), 1 ); // This is an existing SIMPLE product
$order->set_address( $address, 'billing' );
$order->calculate_totals();
$order->update_status( 'completed', 'Imported order', true );
}
add_action( 'woocommerce_new_order', 'create_vip_order', 10, 1 );
By using this hook, you will have access to the $order_id
parameter, which allows you to retrieve the order object using the wc_get_order()
function.
🎉 Now you should be able to create new orders programmatically and have customer information and product line items properly added to the order!
📣 Get Involved!
We hope this guide helped you solve your issue and get your WooCommerce orders flowing smoothly. If you have any other questions or need further assistance, feel free to leave a comment below. We'd love to hear from you! 😊
Don't forget to share this guide with your fellow developers or WooCommerce users who might find it helpful. Let's spread the knowledge and help everyone build awesome digital stores! 🚀🙌