Preventing Laravel adding multiple records to a pivot table
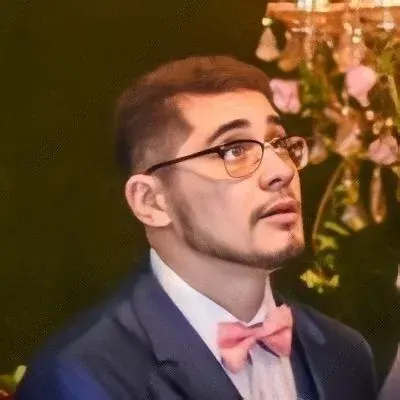
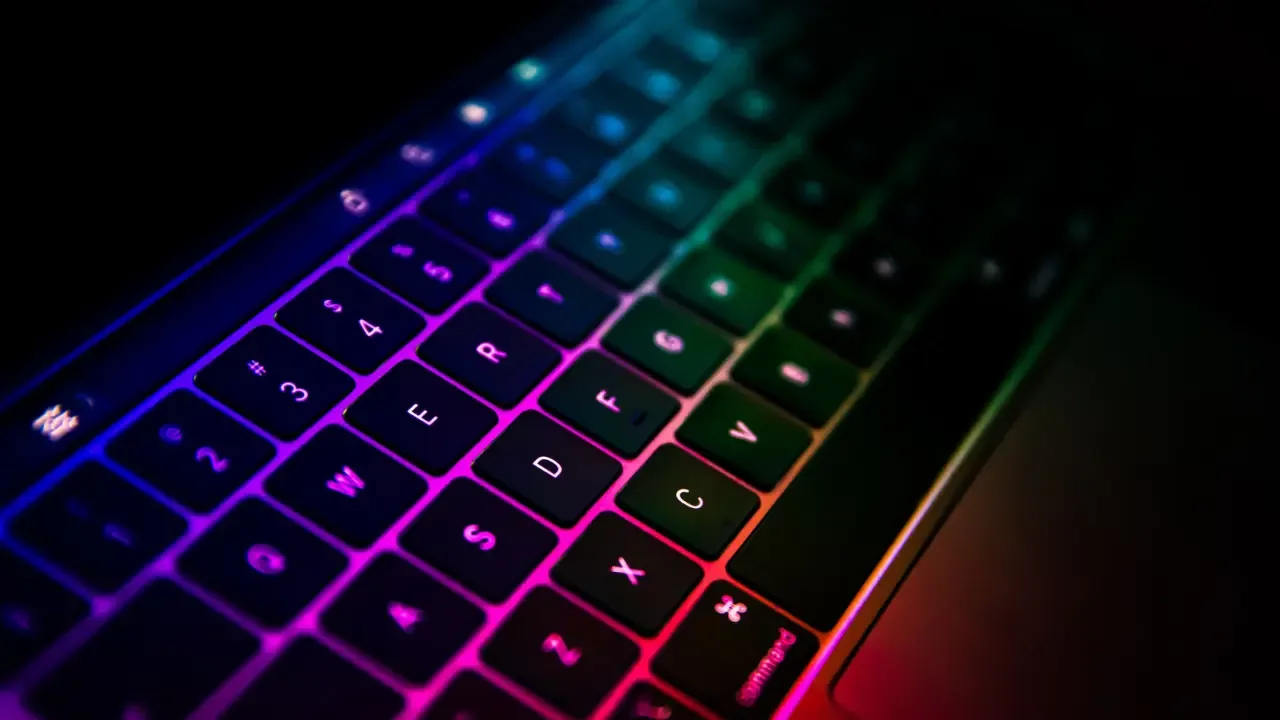
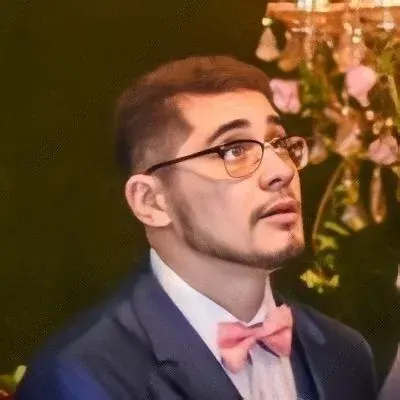
🛡️ Preventing Laravel Adding Multiple Records to a Pivot Table 🛡️
So, you've encountered a common issue when dealing with many-to-many relationships in Laravel. It's frustrating when the user accidentally adds duplicate entries to the pivot table 😫. But fear not, my friend! In this blog post, we'll explore easy solutions to prevent Laravel from adding multiple records to a pivot table. Let's dive in!
The Problem 🤔
When using the attach()
method to add an item to the cart, Laravel correctly adds a record to the pivot table. However, if the user clicks on the same link again, Laravel adds another duplicate entry to the pivot table. This behavior is not what we desire. We want to add a record to the pivot table only if it doesn't already exist.
Solution 1: Using syncWithoutDetaching()
🔄
Laravel provides us with a handy method called syncWithoutDetaching()
to handle this situation effortlessly. Instead of using attach()
, we can use syncWithoutDetaching()
to add a record to the pivot table. Here's how you can use it:
$cart->items()->syncWithoutDetaching($item);
Ta-da! 🎉 With syncWithoutDetaching()
, Laravel will add a record to the pivot table only if it doesn't already exist. No more duplicates!
Solution 2: Checking Pivot Table Manually 🕵️♂️
If you prefer a more hands-on approach, you can manually check the pivot table to determine if a matching record already exists before adding a new one. Here's how you can achieve that:
if (!$cart->items->contains($item)) {
$cart->items()->attach($item);
}
By using the contains()
method, we check if the $item
already exists in the related items. If it doesn't exist, we then proceed to attach it. Simple and effective! 🙌
Conclusion 📝
Preventing Laravel from adding multiple records to a pivot table is no longer a mystery! You have two fantastic solutions at your disposal: syncWithoutDetaching()
and checking the pivot table manually using contains()
. Choose the approach that suits your needs and keep those pivot table duplicates at bay! 🚫🔄
Now it's your turn! Have you ever encountered this issue? How did you solve it? Share your experience in the comments below and let's engage in a tech discussion 🚀.