Populating a database in a Laravel migration file
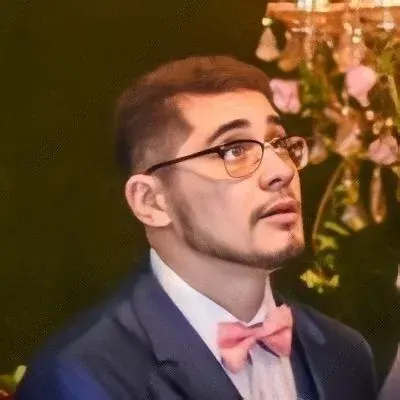
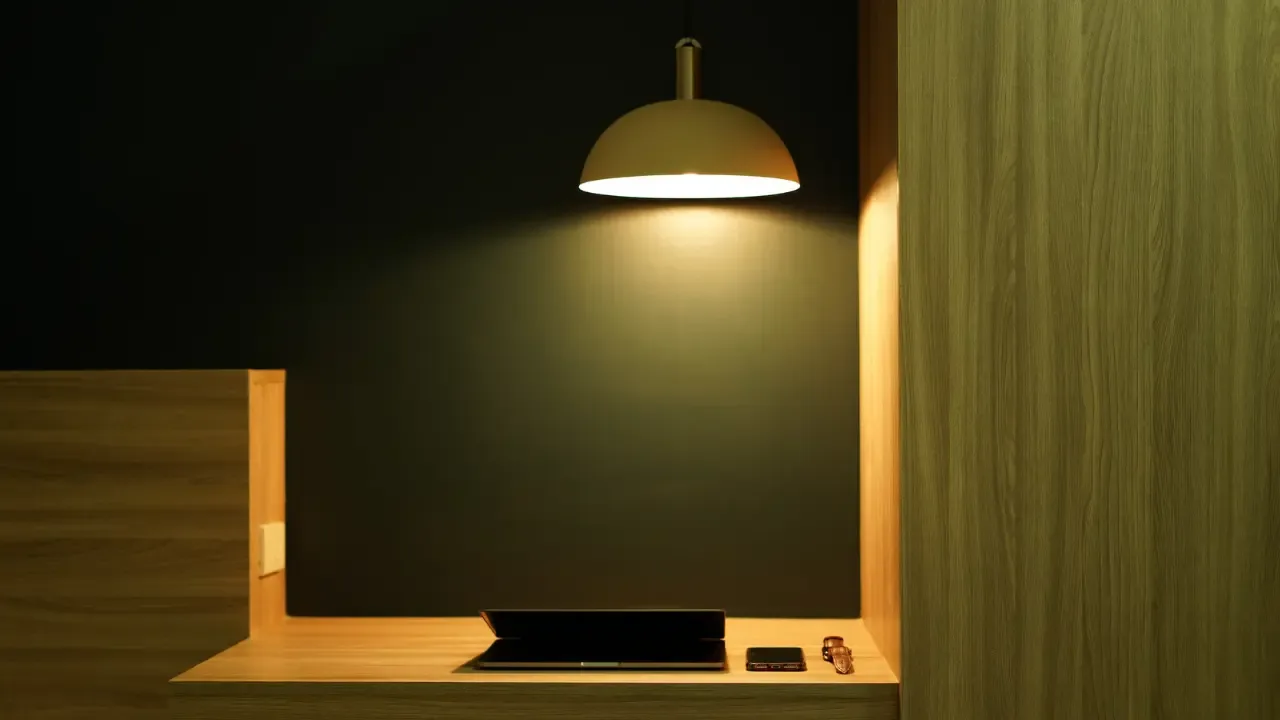
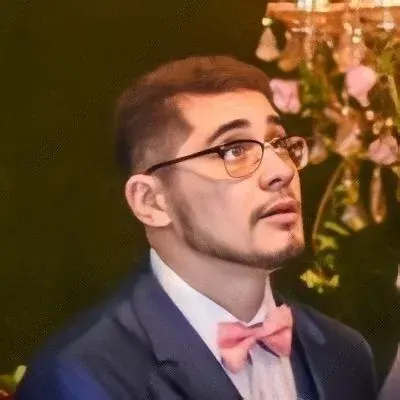
🚀 Populating a Database in a Laravel Migration File
So you're starting your journey with Laravel and have successfully created a migration file for your users
table. But now, you want to populate a user record as part of the migration. No worries! I've got you covered. In this blog post, I'll walk you through the process of populating a database in a Laravel migration file, addressing common issues and providing easy solutions.
The Problem: 🤔
Here's the scenario: you've written your migration code, included the data to be inserted, and excitedly run php artisan migrate
to see your magic happen. But instead of witnessing a successful migration, you're faced with the following error message:
SQLSTATE[42S02]: Base table or view not found: 1146 Table 'vantage.users' doesn't exist
Clearly, Artisan hasn't created the table yet, and this is causing the issue. But fear not, there is indeed a way to use Fluent Query to populate data as part of a migration.
The Solution: 💡
To overcome this issue, you can make use of Laravel's Schema Builder, which allows you to create and modify database tables using a fluent syntax. Here's how you can modify your migration file to successfully populate the users
table:
public function up()
{
Schema::create('users', function($table){
$table->increments('id');
$table->string('email', 255);
$table->string('password', 64);
$table->boolean('verified');
$table->string('token', 255);
$table->timestamps();
});
DB::table('users')->insert(
array(
'email' => 'name@domain.example',
'verified' => true
)
);
}
By moving the data insertion code inside the closure of the create
method, you ensure that the table is created before attempting to populate it. Now, when you run php artisan migrate
, you should successfully create the table and populate it with the specified data.
Conclusion: 🎉
Congrats! You've successfully learned how to populate a database in a Laravel migration file. Remember to always keep the order of operations in mind when performing migrations. By utilizing Laravel's Schema Builder and moving your data insertion code after the table creation, you can avoid the "table not found" issue and insert data seamlessly.
If you have any other questions or run into any other Laravel-related hurdles, feel free to reach out. Happy coding! 💻🚀
[ARTICLE BONUS:] Did this article help you? Have any additional tips or tricks to share? Let's start a conversation in the comments section below! 👇