PHPDoc type hinting for array of objects?
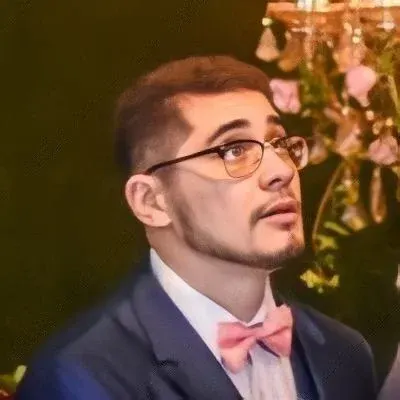
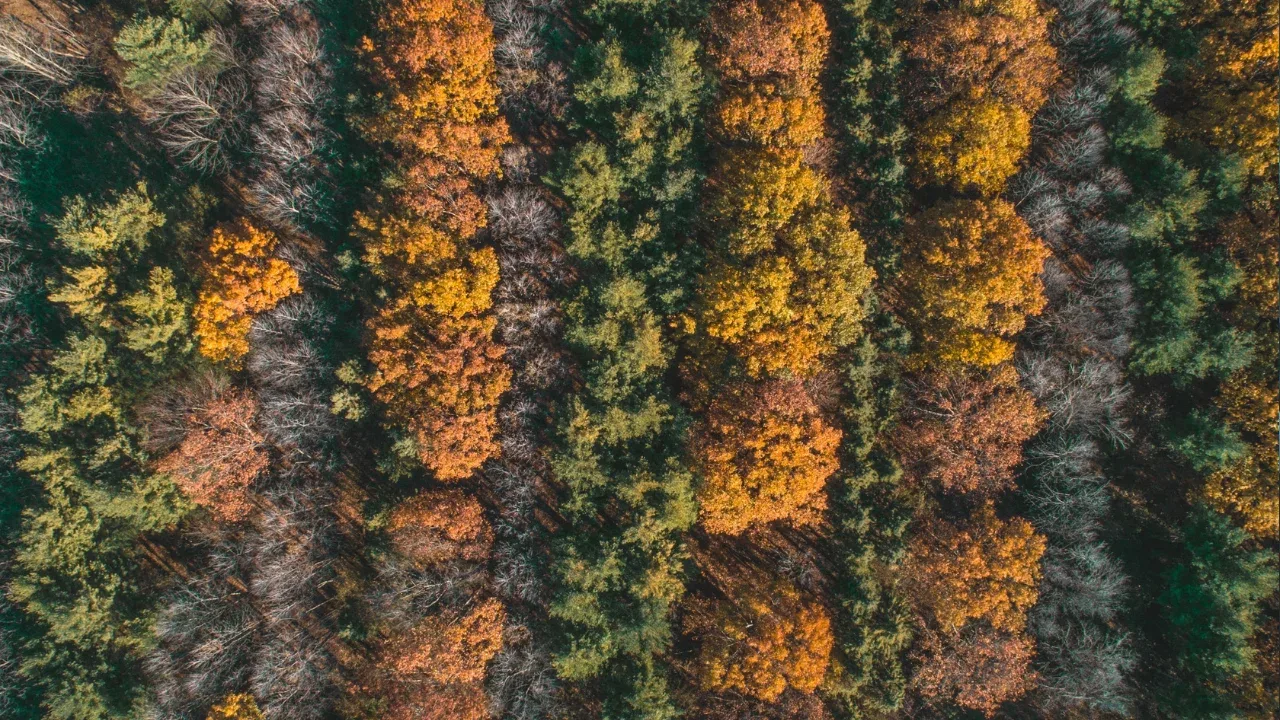
📝 PHPDoc Type Hinting for Array of Objects
Ever wondered how to use PHPDoc to specify the type of an array of objects? 🤔 It's a common issue that many developers face when trying to provide proper type hints in their code. But fear not, because we've got you covered with some easy solutions! Let's dive right in. 💪
The Problem 💥
When it comes to PHPDoc, you can use the @var
tag to specify the type of a member variable. This is super helpful because it allows IDEs like PHPEd to provide intelligent code insights for that variable. But what if you have an array of objects and need to specify the type for that? 🤷♂️
Consider the following code snippet:
class Test
{
/** @var SomeObj */
private $someObjInstance;
}
This works perfectly fine for a single object of type SomeObj
. But what about an array of SomeObj
objects? How can we hint the type for that?
The Solution 🌟
Unfortunately, simply using @var array
is not enough. And @var array(SomeObj)
doesn't seem to be a valid option either. But fret not, there are a few alternative solutions you can try!
Option 1: Using Inline PHPDoc 📝
One option is to use inline PHPDoc within the code. This allows you to specify the type for the array directly. Here's how:
class Test
{
/** @var SomeObj[] */
private $someObjArray;
}
By appending []
after the object type, we can indicate that someObjArray
is an array of SomeObj
objects. This should provide the desired code hinting when working with the array later on.
Option 2: Utilizing PHPDoc Outside Class ✨
Another option is to move the PHPDoc outside the class declaration and use the @property
tag instead. This approach is particularly useful when you need to specify the type of static properties or properties with a different visibility than private. Here's an example:
/**
* @property SomeObj[] $someObjArray
*/
class Test
{
private $someObjArray;
}
By placing the type hint for the array outside the class, we can provide clear instructions to the IDE about the expected type.
The Call-to-Action 📣
Now that you're equipped with these handy solutions, give them a try the next time you encounter the need to type hint an array of objects in your PHP code! 🚀
Do you have any other tips or tricks that you'd like to share? Let us know in the comments below! We'd love to hear from you and learn from your experiences. Happy coding! 💻✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
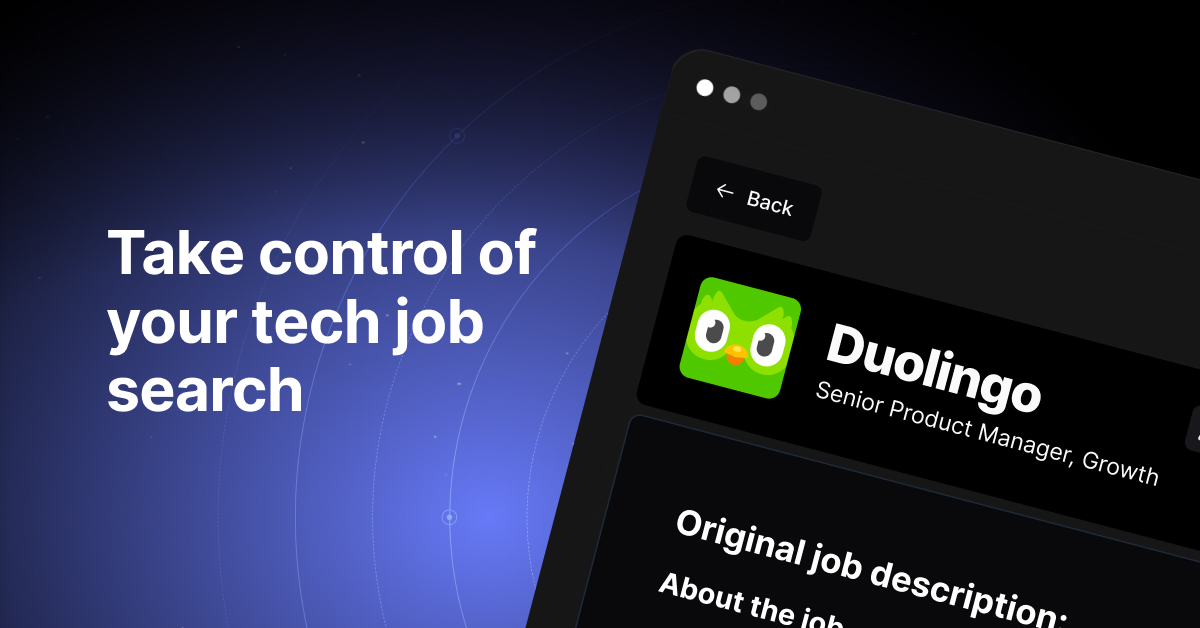