PHP random string generator
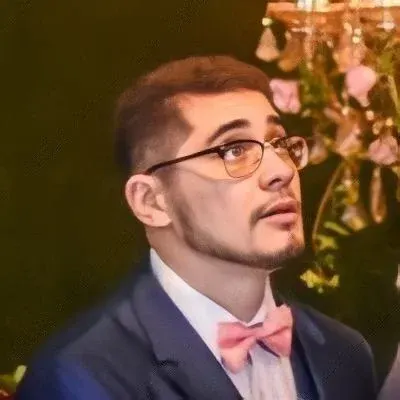

🎲 The PHP Random String Generator Guide 🎲
Are you trying to create a random string in PHP, but you're getting no output? Don't fret, I've got you covered! In this guide, I'll address common issues, provide easy solutions, and help you generate that random string in no time. Let's dive in! 💪
🤔 Understanding the Problem
The code you provided has a minor mistake. In the RandomString()
function, you correctly define the $randstring
variable, but inside the for
loop, you're assigning a new character to it on each iteration instead of appending to it. As a result, only the last character gets assigned to $randstring
. To fix this, we need to use the concatenation operator (.
) instead of the assignment operator (=
) in the loop. 🔄
💡 The Solution
Here's the corrected code:
<?php
function RandomString()
{
$characters = '0123456789abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ';
$randstring = '';
for ($i = 0; $i < 10; $i++) {
$randstring .= $characters[rand(0, strlen($characters))];
}
return $randstring;
}
$randomString = RandomString();
echo $randomString;
?>
In this updated code, the .=
operator will append each randomly chosen character to the $randstring
variable, resulting in a complete 10-character random string.
🎉 The Result
Once you've made the necessary changes, you should see a randomly generated string when executing the PHP script. 🚀
📣 Share Your Thoughts
Have you ever struggled with generating random strings in PHP? Did this guide help you? Share your experience in the comments below! Let's engage and learn together. 🤝
Remember, don't hesitate to reach out if you have any further questions. Happy coding! 💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
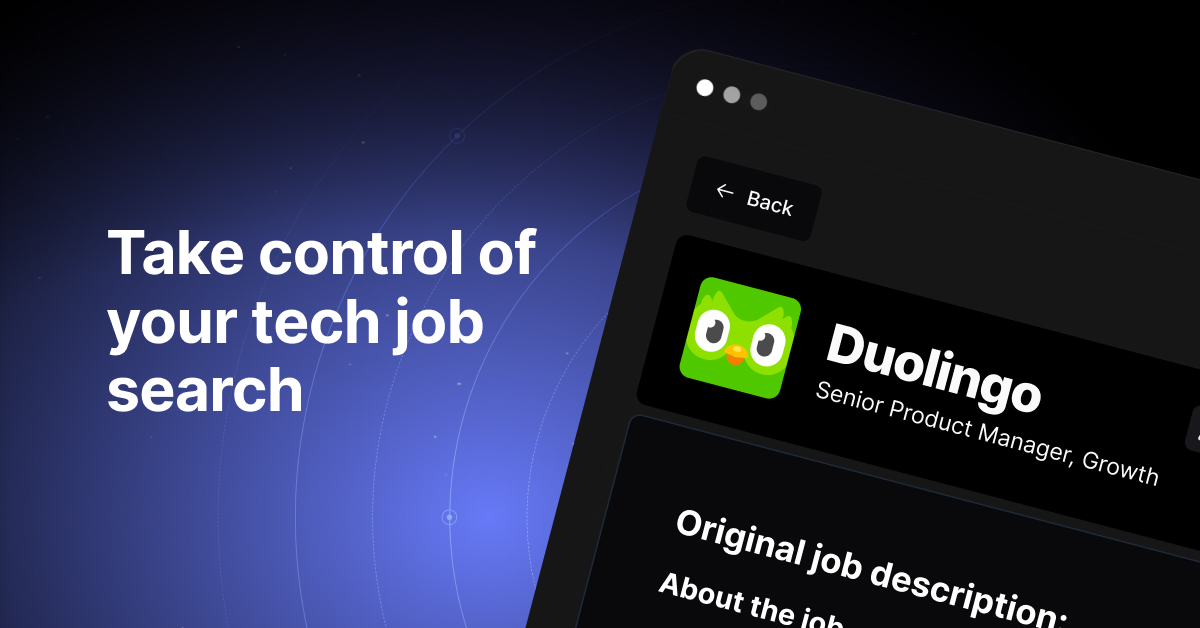