PHP mail function doesn"t complete sending of e-mail
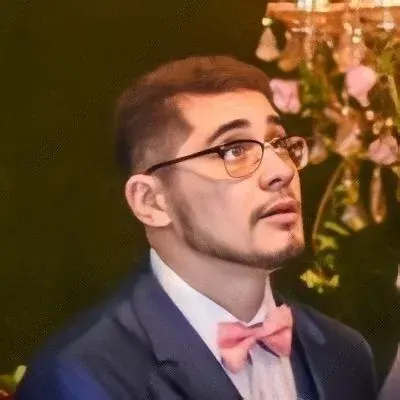
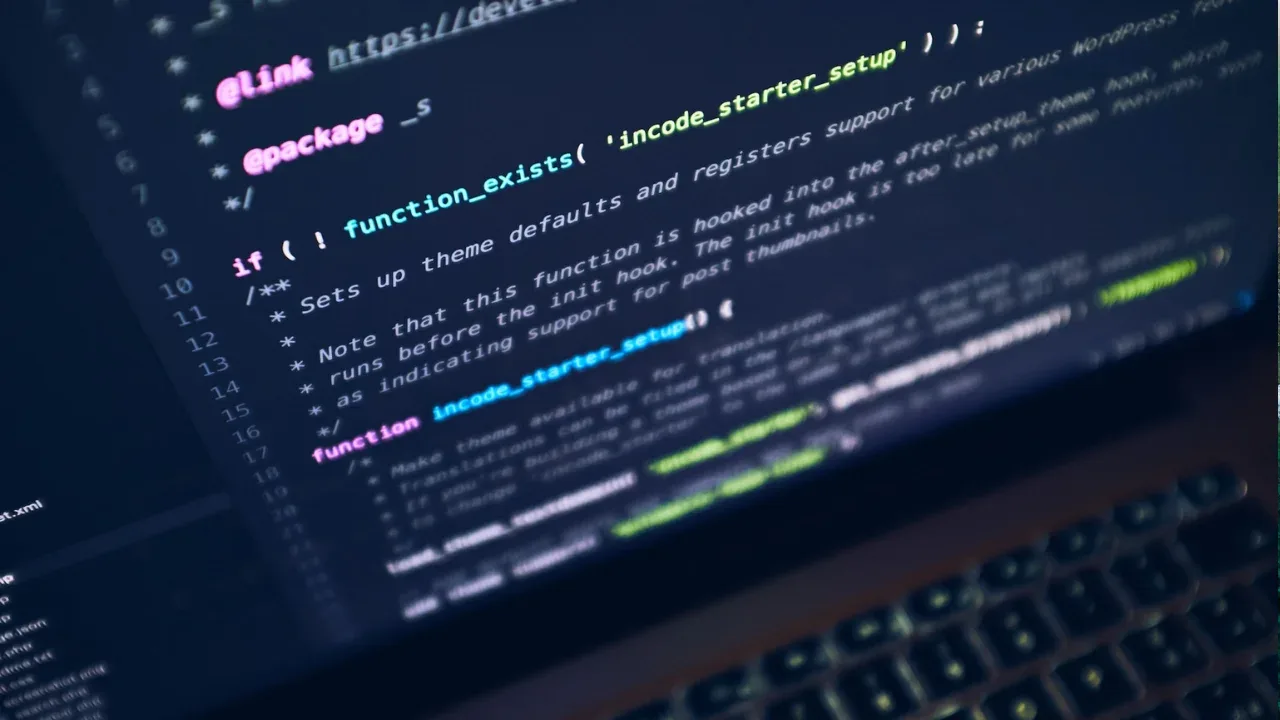
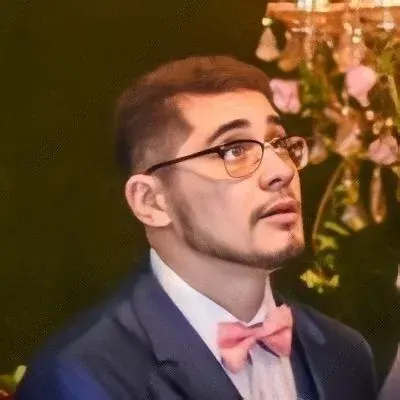
📧📨🚫 PHP Mail Function Not Sending Emails? Here's the Solution! 🚀💡
So, you've created a website with a beautiful contact form, but when a user submits it, the PHP mail function fails to send the email. 😩 Don't fret! This common issue has a simple fix.
🔍 Understanding the Problem
Let's take a closer look at the code snippet you shared:
$name = $_POST['name'];
$email = $_POST['email'];
$message = $_POST['message'];
$from = 'From: yoursite.com';
$to = 'contact@yoursite.com';
$subject = 'Customer Inquiry';
$body = "From: $name\n E-Mail: $email\n Message:\n $message";
if ($_POST['submit']) {
if (mail($to, $subject, $body, $from)) {
echo '<p>Your message has been sent!</p>';
} else {
echo '<p>Something went wrong, go back and try again!</p>';
}
}
The code looks fine at first glance, but it's missing an essential header. 📨💨
🔧 Solution: Adding the Right Headers
To ensure that your emails go through successfully, you need to include proper headers in the mail()
function. Here's the updated code:
$name = $_POST['name'];
$email = $_POST['email'];
$message = $_POST['message'];
$to = 'contact@yoursite.com';
$subject = 'Customer Inquiry';
$headers = "From: yoursite.com\r\n";
$headers .= "Reply-To: $email\r\n";
$headers .= "MIME-Version: 1.0\r\n";
$headers .= "Content-Type: text/html; charset=UTF-8\r\n";
$body = "From: $name\nEmail: $email\nMessage:\n$message";
if ($_POST['submit']) {
if (mail($to, $subject, $body, $headers)) {
echo '<p>Your message has been sent!</p>';
} else {
echo '<p>Something went wrong, go back and try again!</p>';
}
}
✨ Explanation: What Does It Do?
1️⃣ We added a header called "Reply-To"
to ensure that when the recipient hits "reply," the email goes back to the original sender.
2️⃣ Included "MIME-Version"
and "Content-Type"
headers to support HTML formatting within the email's body.
3️⃣ Adjusted the order of the headers and the content structure to make it more readable and maintainable.
🏁 Final Thoughts
With these simple changes, your PHP mail function should now successfully send emails. 💌 Give it a try and see the magic happen!
If you encounter any other issues or have more questions, feel free to leave a comment below or reach out to me on Twitter. I'm here to help!
Now, it's time for you to implement these changes and make those emails fly! Don't forget to share your success story and spread the love! ❤️
Happy coding! ✨👩💻🚀