Passing data to a closure in Laravel 4
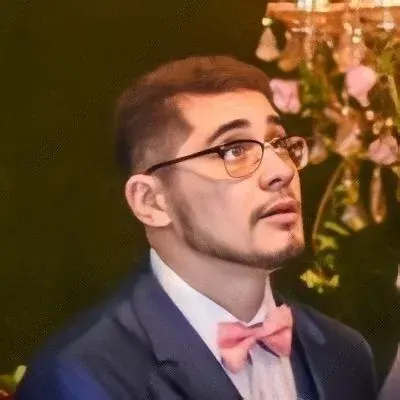
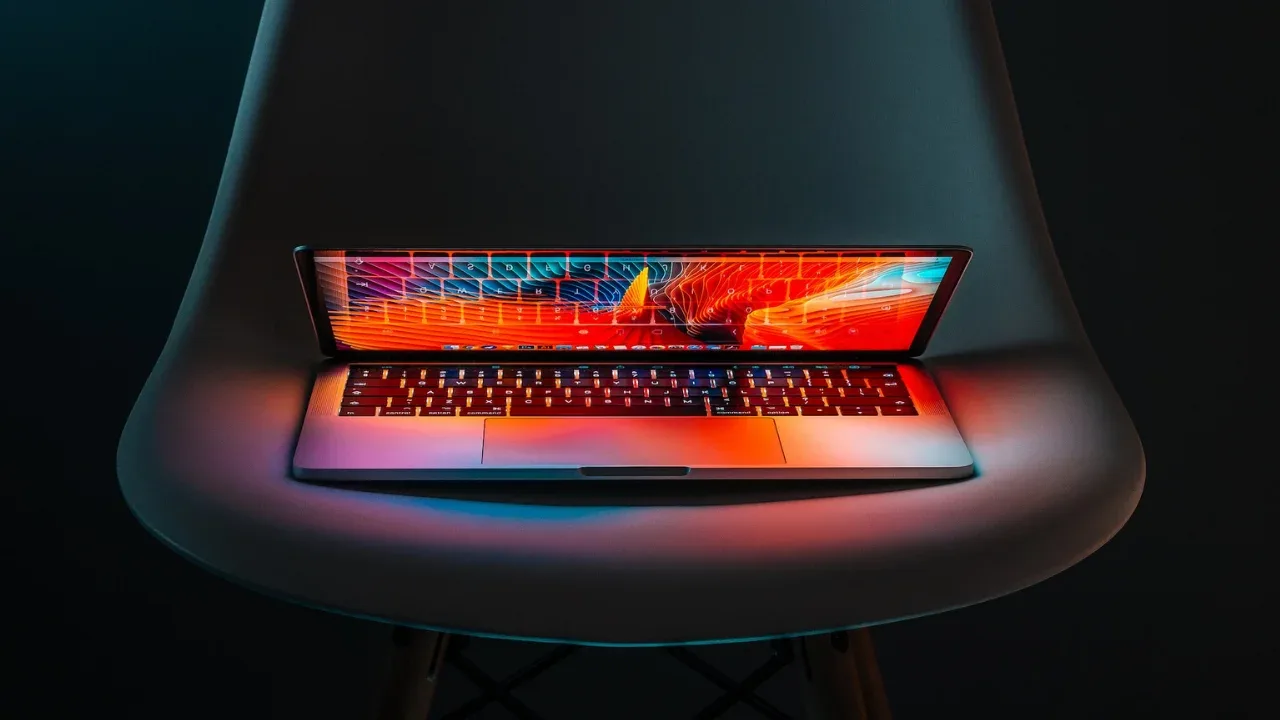
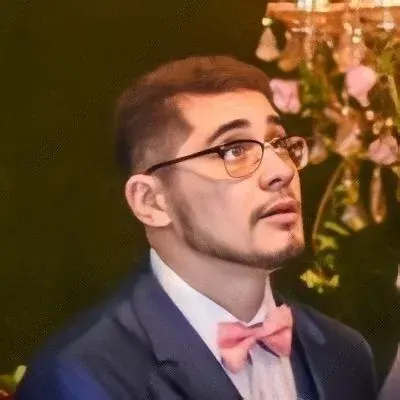
Passing Data to a Closure in Laravel 4: A Simple Solution 👨💻
So you're working with Laravel 4 and trying to pass data to a closure in the Mail class, but you're running into issues. Don't worry, you're not alone! Many developers have faced this problem before. In this blog post, we'll learn how to overcome this challenge and get your code working smoothly. Let's dive in! 🚀
The Problem: Undefined Scope Issues 🤔
The code snippet you shared seems perfectly fine at first glance. However, the error you're encountering suggests that the $team
object is not available within the closure. This error occurs because of variable scope limitations within closures. Closures don't automatically inherit the scope of the parent function or class.
The Solution: Use the use
Keyword 🙌
To pass data to a closure in Laravel 4, you can use the use
keyword to explicitly import variables from the parent scope. By doing this, you'll make the $team
object accessible within the closure. Here's what your updated code should look like:
Mail::send('emails.report', $data, function($m) use ($team) {
$m->to($team->senior->email, $team->senior->first_name . ' ' . $team->senior->last_name);
$m->cc($team->junior->email, $team->junior->first_name . ' ' . $team->junior->last_name);
$m->subject('Monthly Report');
$m->from('info@website.example', 'Sender');
});
By including use ($team)
after the closure's parameter list, you're telling Laravel to import the $team
object from the parent scope.
Real World Example 🌍
Let's say you're using the Mail
class to send weekly reports to different teams in your organization. You need to pass the team data to the closure to personalize each email sent. With the use
keyword, you can achieve this easily. Here's an example:
$teams = Team::all();
foreach ($teams as $team) {
Mail::send('emails.report', $data, function($m) use ($team) {
$m->to($team->senior->email, $team->senior->first_name . ' ' . $team->senior->last_name);
$m->cc($team->junior->email, $team->junior->first_name . ' ' . $team->junior->last_name);
$m->subject('Weekly Report for ' . $team->name);
$m->from('info@website.example', 'Sender');
});
}
By leveraging the power of the use
keyword, you can pass the $team
object for each iteration, ensuring that the closure has access to the correct team data.
Your Turn: Share your Success Story! 💪
Now that you know how to pass data to a closure in Laravel 4, it's time for you to give it a try! Implement the suggested solution in your code and see if it resolves the issue. If you face any other challenges or have additional questions, feel free to drop a comment below. We'd love to hear from you and help you on your Laravel journey! 👇
Happy coding! 🎉