MySQL query to get column names?
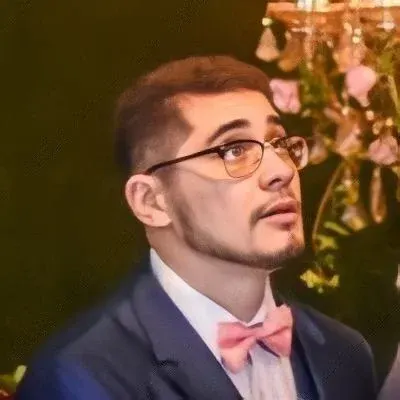
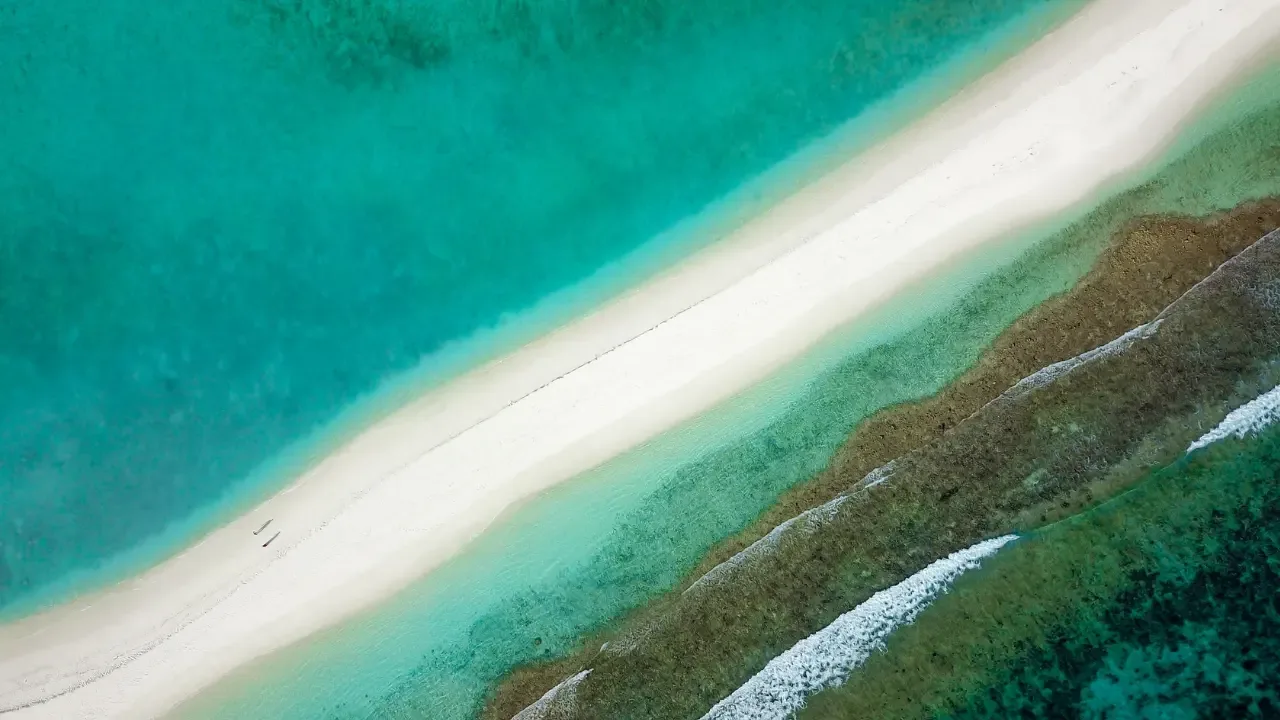
MySQL Query to Get Column Names
Are you stuck trying to retrieve the column names of a MySQL table using PHP? Don't worry, we've got you covered! In this blog post, we'll address this common issue and provide you with easy solutions to get the job done. 🎯
The Problem
Let's start by understanding the problem at hand. You want to retrieve all the column names of a MySQL table and store them in an array using PHP. 📋
The Solution
Thankfully, MySQL provides us with an easy solution. We can utilize the SHOW COLUMNS
command to fetch the column names. Let's take a look at how you can achieve this.
$tableName = "your_table_name";
$query = "SHOW COLUMNS FROM $tableName";
$result = mysqli_query($connection, $query);
$columnNames = array();
while ($row = mysqli_fetch_assoc($result)) {
$columnNames[] = $row['Field'];
}
Let's break down the code snippet:
Replace
"your_table_name"
with the actual name of the table you want to retrieve column names from.Execute the query using
mysqli_query()
with your database connection and query as parameters.Create an empty array
$columnNames
to store the column names.Iterate over the result using
mysqli_fetch_assoc()
to fetch each row.Retrieve the value of the 'Field' column from each row and add it to the
$columnNames
array.
After executing this code, you can access the column names in the $columnNames
array.
Example
Let's consider an example to make things clearer. Suppose we have a table named products
with three columns: id
, name
, price
.
Using the solution we provided earlier, here's how you can retrieve the column names of the products
table:
$tablename = "products";
$query = "SHOW COLUMNS FROM $tablename";
$result = mysqli_query($connection, $query);
$columnNames = array();
while ($row = mysqli_fetch_assoc($result)) {
$columnNames[] = $row['Field'];
}
// Accessing the column names
foreach ($columnNames as $columnName) {
echo $columnName . "<br>";
}
Running this code will output:
id
name
price
Awesome, right? Now you have the column names stored in the $columnNames
array and can use them as needed in your PHP code.
Time to Try It!
Now that you understand how to retrieve column names from a MySQL table using PHP, give it a try yourself! Implement this solution in your project, and let us know how it works for you. 🤩
If you have any questions or facing any issues, feel free to drop them in the comments below. Our expert team and the vibrant community will be there to assist you!
Happy coding! 💻💪
Cover image source: Freepik
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
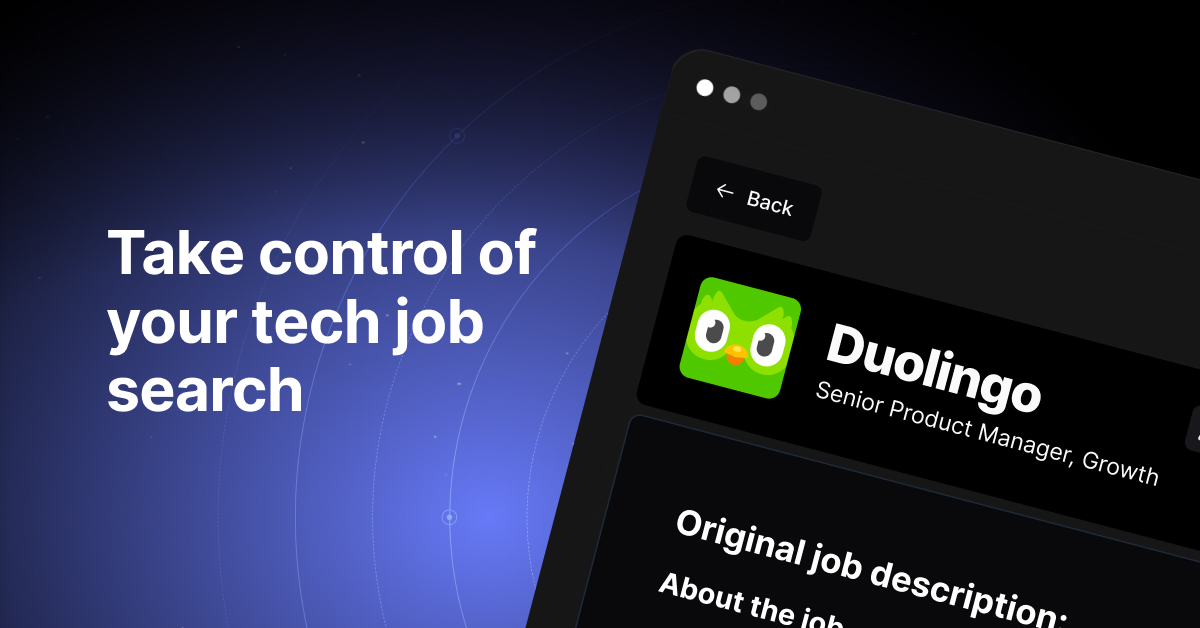