List of Big-O for PHP functions
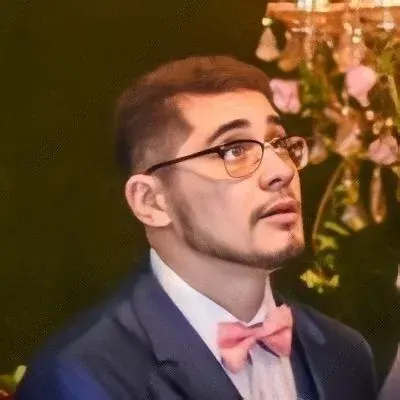
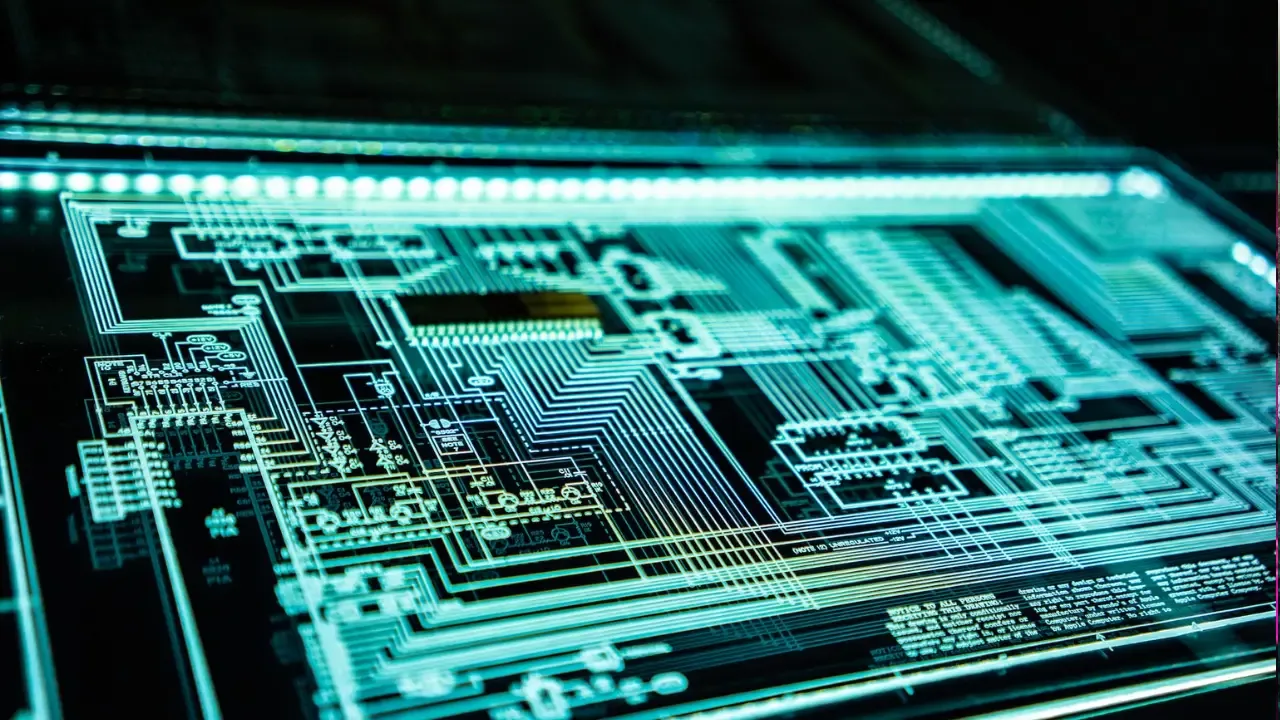
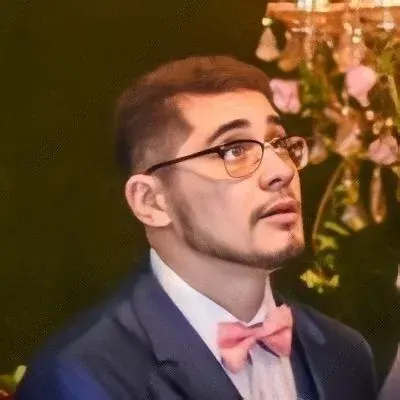
📝 The Big-O Cheat Sheet for PHP Functions
If you've been using PHP for a while, you might have noticed that not all built-in PHP functions perform as fast as you expected. In some cases, the speed of a function can vary depending on the size of the data you're working with. Understanding the time complexity of these functions can help you optimize your code and improve performance. 🚀
Let's dive into some common issues and solutions when it comes to the time complexity (Big-O) of PHP functions.
🐢 Slow vs. 🚄 Fast: A Prime Example
Consider the two possible implementations of a function that checks if a number is prime using a cached array of primes:
// ⏰ Very slow for large $prime_array
$prime_array = array(2, 3, 5, 7, 11, 13, .... 104729, ...);
$result_array = array();
foreach ($prime_array as $number) {
$result_array[$number] = in_array($number, $large_prime_array);
}
// ⏰ Speed is much less dependent on the size of $prime_array and runs much faster.
$prime_array = array(2 => NULL, 3 => NULL, 5 => NULL, 7 => NULL,
11 => NULL, 13 => NULL, .... 104729 => NULL, ... );
foreach ($prime_array as $number) {
$result_array[$number] = array_key_exists($number, $large_prime_array);
}
In the first implementation, we use the in_array
function, which performs a linear search with a time complexity of O(n). This means that as the size of $prime_array
grows, the function will slow down linearly. On the other hand, the second implementation utilizes the array_key_exists
function, which provides a hash lookup implementation with a constant time complexity of O(1). It will remain fast even with a growing hash table unless it becomes extremely populated (in which case it becomes O(n)).
📚 The Big O Mystery
Now, you might be wondering if there's a comprehensive list of the theoretical or practical Big-O times for all the built-in PHP functions. Unfortunately, the exact time complexity can be challenging to predict due to the unknown core data structures used by PHP.
Take functions like array_merge
, array_merge_recursive
, array_reverse
, array_intersect
, array_combine
, and str_replace
(with array inputs), for example. Their Big-O times are harder to pin down because their implementation depends on the underlying data structures of PHP. 🕵️♂️
🤔 Trial and Error No More: Some Practical Tips
While a complete and definitive list of Big-O times for PHP functions might not exist, here are some practical tips to keep in mind:
Leverage the power of associative arrays: As demonstrated in our prime number example, using associative arrays and hash lookups (
array_key_exists
) can often yield better performance than linear searches (in_array
). Whenever possible, consider whether you can utilize associative arrays to optimize your code.Consider the worst-case scenario: If a function's implementation relies on unknown core data structures, assume the worst-case time complexity. In general, this would mean planning for a linear search (O(n)) unless you know that the underlying implementation is optimized for a faster lookup.
Measure, benchmark, and profile: When dealing with performance-critical code, it's essential to measure the execution time and profile your application. Tools like Xdebug, Blackfire, or XHProf can help you identify the bottlenecks and areas where you can improve the time complexity.
📣 Share Your Insights and Engage!
We hope these tips and insights help you navigate the Big-O complexities of PHP functions. While a definitive list may be elusive, sharing your knowledge and experiences can benefit the community.
📢 Have you found any interesting Big-O times for specific PHP functions? Share them in the comments below and join the conversation! Together, we can unlock the secrets of PHP performance optimization and make our code run like lightning. ⚡️✨