Laravel where on relationship object
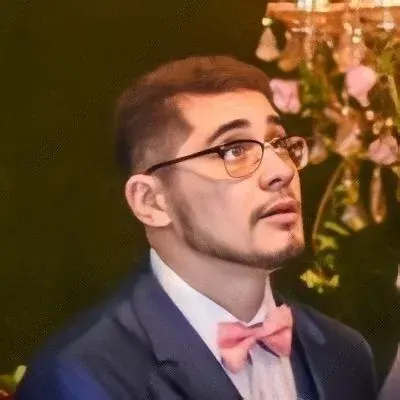
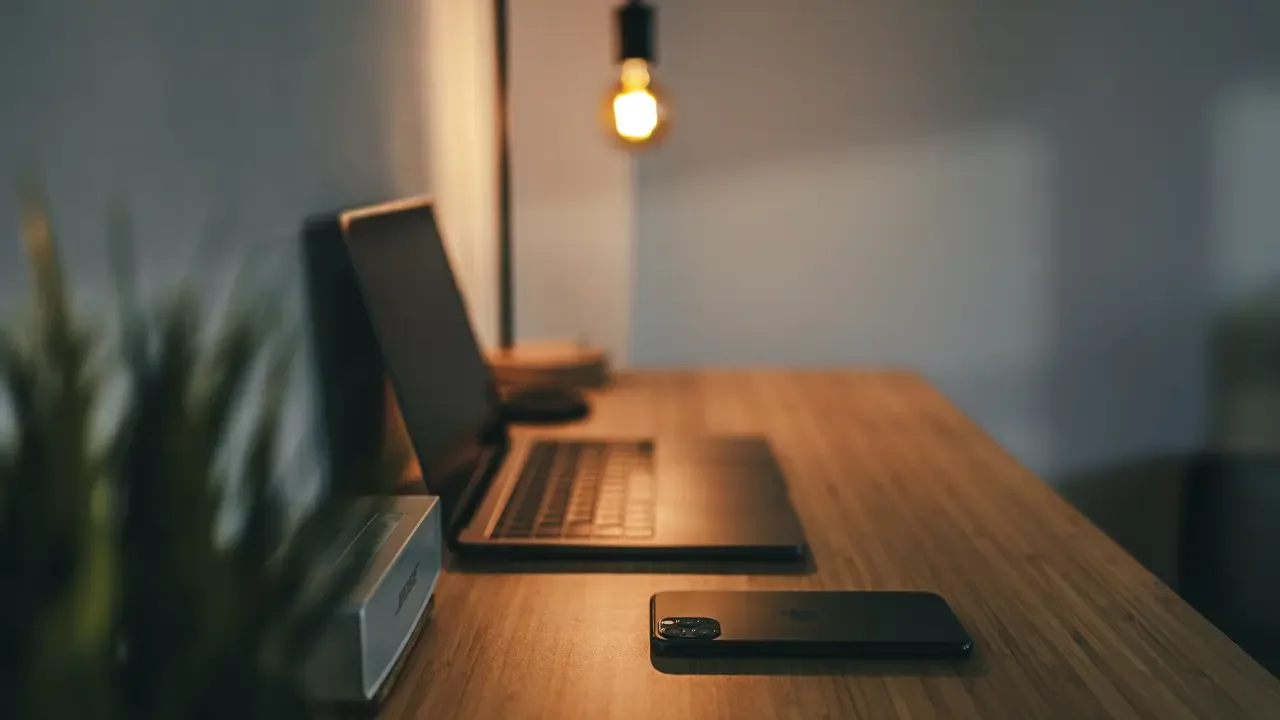
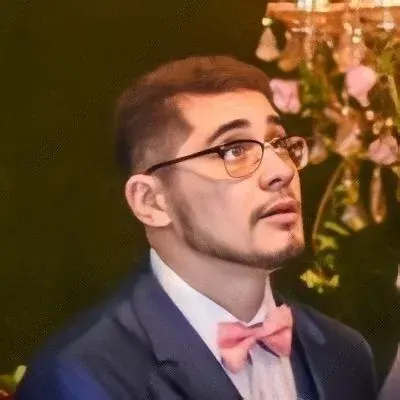
How to Perform a "where" Query on Laravel Relationship Objects: A Complete Guide
So you're developing a web API using Laravel and you've come across the need to perform a "where" query on a relationship object. 🤔 You want to get all the events with a specific participant, but you're not sure how to do it efficiently using Laravel 5 and Eloquent. Don't worry, we've got you covered! In this guide, we'll walk you through the common issues and provide you with easy solutions.
Understanding the Classes and Relationships
First, let's take a quick look at the classes and their relationships to better understand the context.
class Event extends Model {
protected $table = 'events';
public $timestamps = false;
public function participants()
{
return $this->hasMany('App\Participant', 'IDEvent', 'ID');
}
public function owner()
{
return $this->hasOne('App\User', 'ID', 'IDOwner');
}
}
class Participant extends Model {
protected $table = 'participants';
public $timestamps = false;
public function user()
{
return $this->belongsTo('App\User', 'IDUser', 'ID');
}
public function event()
{
return $this->belongsTo('App\Event', 'IDEvent', 'ID');
}
}
In this example, an Event
can have multiple Participants
, and each Participant
belongs to a single Event
. Now, let's dive into the query problem and its solutions.
The Query Issue and Potential Solutions
The query you initially tried was:
Event::with('participants')->where('IDUser', 1)->get();
However, this applies the where
condition on the Event
model itself, not on its Participants
. So, it doesn't give you the desired results. 🙁
The next attempt was:
Participant::where('IDUser', 1)->event()->get();
But this throws an exception. The issue here is that you're trying to use the event()
relationship method as a query builder method, which is incorrect.
Solution 1: Chaining the "where" Query
To address this, you can chain the where
query directly on the relationship object. Here's how you can modify the query:
Event::whereHas('participants', function ($query) {
$query->where('IDUser', 1);
})->get();
In this solution, we use the whereHas
method, which allows us to specify additional filters on the related models. We pass a closure to the method and define our where
condition inside that closure.
Solution 2: Using "with" and "where" Together
Another method is to combine the with
and where
methods to achieve the desired result. Here's how it can be done:
Event::with(['participants' => function ($query) {
$query->where('IDUser', 1);
}])->get();
In this solution, we pass an array to the with
method, where the key is the relationship name ('participants') and the value is a closure where we define the additional where
condition.
Choosing the Best Approach
Between Solution 1 and Solution 2, both methods are valid and will give you the desired results. The choice between them depends on your specific use case and preferences.
Solution 1 (whereHas
) might be more suitable if you want to get only the events that have at least one participant matching the given condition. It allows you to filter the events directly based on the relationship.
Solution 2 (with
and where
) is useful when you want to retrieve all events with their participants, but only include the participants matching the given condition. It enables you to eager load the relationship while applying the where
clause.
Conclusion
Performing a "where" query on Laravel relationship objects may seem tricky at first, but with the right approach, it becomes a breeze. By using either the whereHas
method or combining the with
and where
methods, you can efficiently filter your data based on relationship conditions.
Now that you've learned how to tackle this common issue, go ahead and give it a try in your own Laravel project! Feel free to experiment with different queries and explore the full potential of Laravel's Eloquent ORM.
If you have any questions or want to share your experience, feel free to leave a comment below. Happy coding! 😄🚀