Laravel: Using try...catch with DB::transaction()
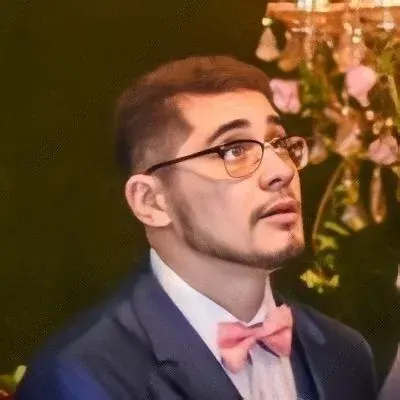
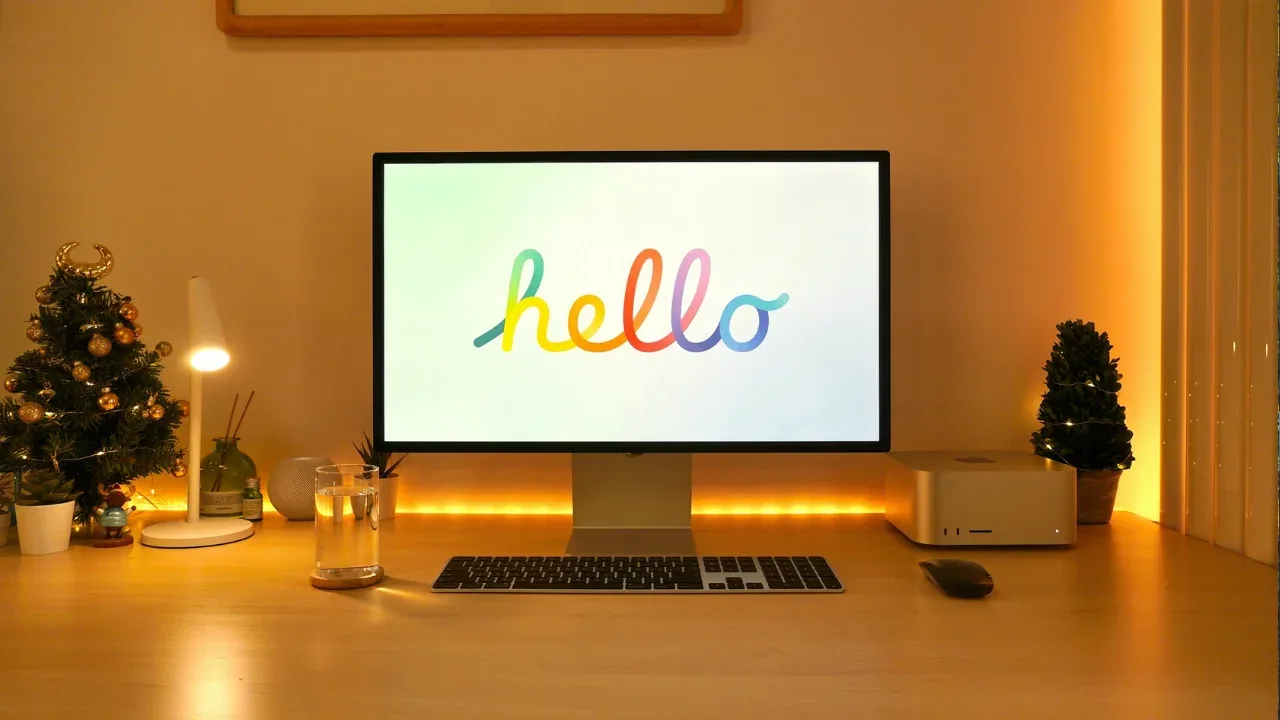
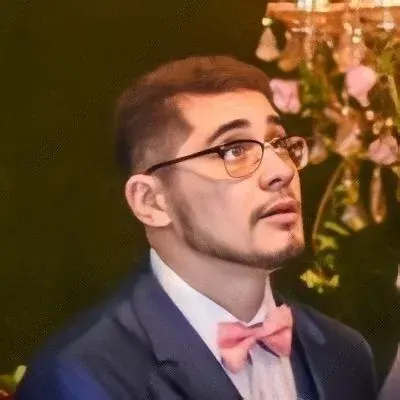
Laravel: Using try...catch with DB::transaction() 💻💥
When working with Laravel and handling multiple insert queries, you might come across the question of whether to use a try...catch
block inside or outside of the DB::transaction()
method. Is it even necessary to include a try...catch
when a transaction will automatically fail if something goes wrong? Let's dive into these scenarios and find the best approach. 😊
🔄 Wrapping DB::transaction() with try...catch
// try...catch
try {
// Transaction
$exception = DB::transaction(function() {
// Do your SQL here
});
if(is_null($exception)) {
return true;
} else {
throw new Exception;
}
}
catch(Exception $e) {
return false;
}
In this scenario, we wrap the DB::transaction()
method with a try...catch
block. It allows us to handle any exceptions that may occur within the transaction, regardless of whether it's a database-related issue or any other exception. If an exception is caught, the transaction will be rolled back automatically.
🔄 Wrapping try...catch within DB::transaction()
// Transaction
$exception = DB::transaction(function() {
// try...catch
try {
// Do your SQL here
}
catch(Exception $e) {
return $e;
}
});
return is_null($exception) ? true : false;
In this scenario, we place the try...catch
block nested within the DB::transaction()
method. The advantage of this approach is that you can handle specific exceptions differently within the transaction. If a database-related exception occurs, it will still trigger a rollback, ensuring data integrity.
🔄 Transaction only, without try...catch
// Transaction only
$exception = DB::transaction(function() {
// Do your SQL here
});
return is_null($exception) ? true : false;
Lastly, you might wonder if it's okay to skip the try...catch
block altogether. In this case, Laravel's DB::transaction()
method automatically wraps the SQL queries within a transaction and rolls back if any exception occurs. However, it's important to note that in this scenario, you won't be able to handle specific exceptions within the transaction.
📍 Conclusion and Best Practice
While it's technically possible to use any of the approaches mentioned above, it's recommended to wrap the DB::transaction()
method with a try...catch
block. This allows for better exception handling, ensuring that you can handle different types of exceptions within the transaction and perform necessary rollbacks if needed.
So next time you find yourself using DB::transaction()
in Laravel, remember to include that try...catch
block for a more robust error handling experience! 😎
Is there a specific approach you prefer when using DB::transaction()
? Let us know in the comments below! Happy coding! 👩💻👨💻