Laravel Unknown Column "updated_at"
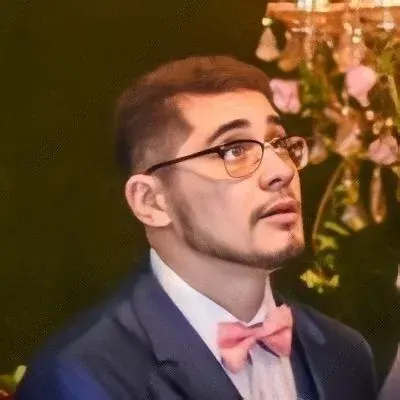
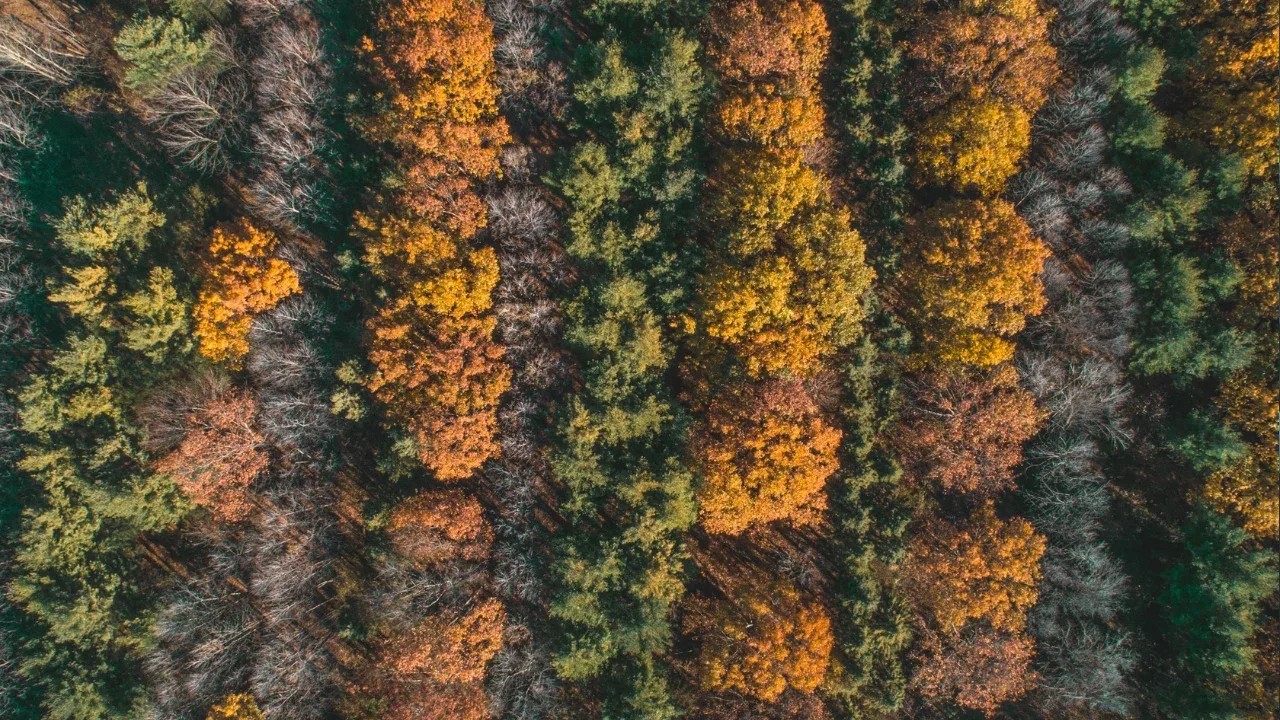
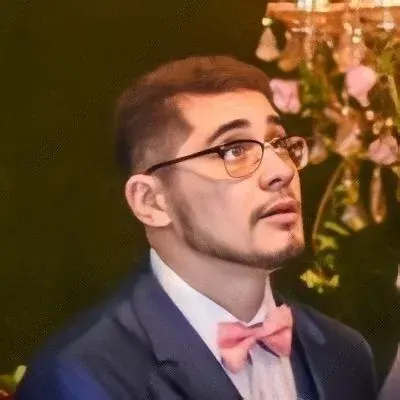
Laravel Unknown Column 'updated_at' Error: Easy Fixes and Troubleshooting
So, you're diving into the world of Laravel, and all was going smoothly until you encountered the dreaded "Unknown column 'updated_at'" error. Don't panic! This common issue can be quickly resolved with a few simple steps. Let's get into it. 💻🌟
Understanding the Error
The error message you received is triggered by Laravel's timestamp feature, which automatically adds the updated_at
and created_at
columns to your database table. However, in your specific case, you're not actively using the updated_at
field, causing the error to pop up.
Analyzing the Code
Let's take a closer look at the code you provided to identify the root cause.
Controller
public function created()
{
if (!User::isValidRegister(Input::all())) {
return Redirect::back()->withInput()->withErrors(User::$errors);
}
// Register the new user or whatever.
$user = new User;
$user->naam = Input::get('naam');
$user->wachtwoord = Hash::make(Input::get('password'));
$user->save();
return Redirect::to('/users');
}
Route
Route::get('created', 'UserController@created');
Model
public static $rules_register = [
'naam' => 'unique:gebruikers,naam'
];
public static $errors;
protected $table = 'gebruikers';
public static function isValidRegister($data)
{
$validation = Validator::make($data, static::$rules_register);
if ($validation->passes()) {
return true;
}
static::$errors = $validation->messages();
return false;
}
Finding a Solution
To fix the "Unknown column 'updated_at'" error, here are two potential solutions, depending on your specific requirements:
Solution 1: Disable Timestamps
If you're not utilizing the updated_at
and created_at
fields in your application, disabling the timestamps feature will prevent Laravel from expecting these columns.
To disable timestamps for a specific model, add the $timestamps
property to your model class and set it to false
. Here's an example:
class User extends Eloquent
{
public $timestamps = false;
// ...
}
By disabling timestamps, the error should no longer persist. However, keep in mind that this option is only suitable if you don't require these timestamp fields in your application.
Solution 2: Add Timestamp Columns
On the other hand, if you do need to keep track of the updated_at
field, you can manually add the missing column to your database table.
Open a database migration file for the
gebruikers
table.Locate the
up
method and add the following line to create theupdated_at
column:
$table->timestamps();
Save the migration file and run the migration command:
php artisan migrate
By including the timestamps()
method in your migration file, Laravel will create the updated_at
and created_at
columns for you.
Conclusion
There you have it! 🎉 To sum it up, the "Unknown column 'updated_at'" error commonly arises when Laravel expects the updated_at
field but cannot find it. By either disabling the timestamps feature or adding the missing column, you can resolve this issue and continue building your Laravel application hassle-free.
If you have any questions or encountered any other roadblocks while working with Laravel, feel free to leave a comment. Your engagement is essential to us as we continue providing helpful content and support to fellow developers. Happy coding! 💪💻