Laravel orderBy on a relationship
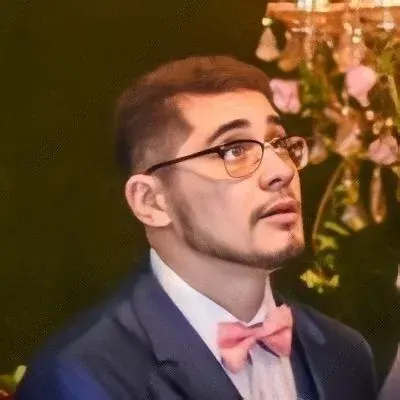
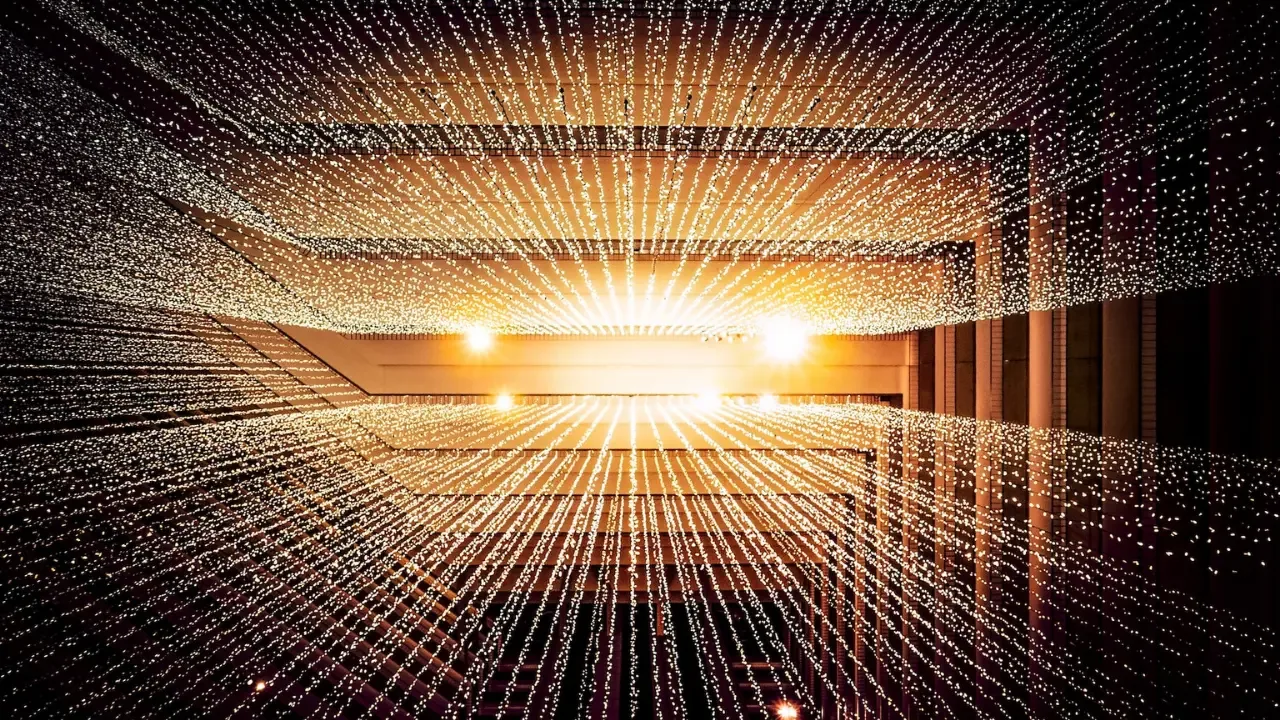
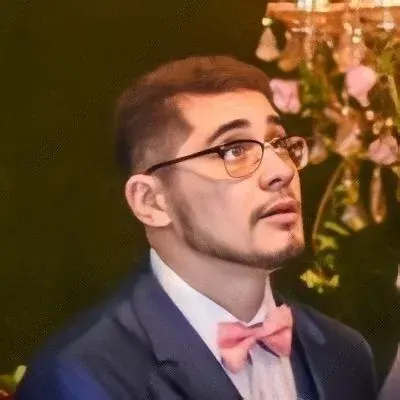
Laravel orderBy on a relationship: Sorting Comments by Post ID
Are you facing a challenge when it comes to sorting comments based on the associated post ID in Laravel? Look no further! In this guide, we will walk you through a common issue that developers encounter and provide easy solutions to help you sort your comments effectively.
The Problem
Let's set the context first. You have a loop that iterates over all the comments posted by the author of a specific post. While displaying these comments, you notice that they are not ordered correctly by the post ID.
@foreach($post->user->comments as $comment)
{
echo "<li>" . $comment->title . " (" . $comment->post->id . ")</li>";
}
This current implementation might produce the following output:
I love this post (3)
This is a comment (5)
This is the second Comment (3)
However, you want to order these comments by their associated post ID, resulting in the correct output:
I love this post (3)
This is the second Comment (3)
This is a comment (5)
The Solution
To achieve the desired ordering, you can make use of the orderBy
method available in Laravel's Eloquent ORM. By modifying your code, you can sort the comments by the post_id
column.
@foreach($post->user->comments()->orderBy('post_id')->get() as $comment)
{
echo "<li>" . $comment->title . " (" . $comment->post->id . ")</li>";
}
By appending the orderBy('post_id')
method to the comments()
relationship, you instruct Laravel to sort the comments in ascending order based on the post_id
column.
🌟 Pro Tip: Custom Sorting
If you need to sort comments in a specific order, such as descending or based on multiple columns, you can customize the orderBy
method accordingly. For example, to sort comments in descending order based on the created_at
column, you can update the code as follows:
@foreach($post->user->comments()->orderBy('created_at', 'desc')->get() as $comment)
{
echo "<li>" . $comment->title . " (" . $comment->post->id . ")</li>";
}
Conclusion
Sorting comments by the associated post ID in Laravel is a breeze with the help of the orderBy
method. By utilizing this convenient method, you can effortlessly order your comments to suit your needs. So, go ahead, implement these techniques, and enhance the display of your comments for a superior user experience.
If you found this guide helpful, feel free to share it with your developer friends. And don't hesitate to comment below if you have any further questions or want to share your experiences. Happy coding! ✨🚀