Laravel migration: unique key is too long, even if specified
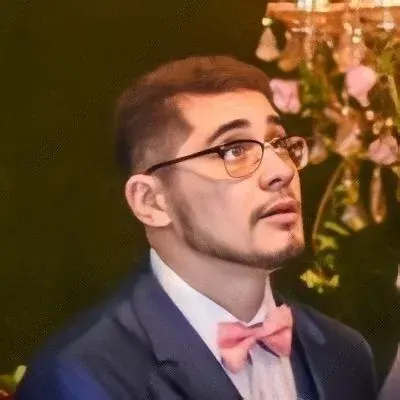
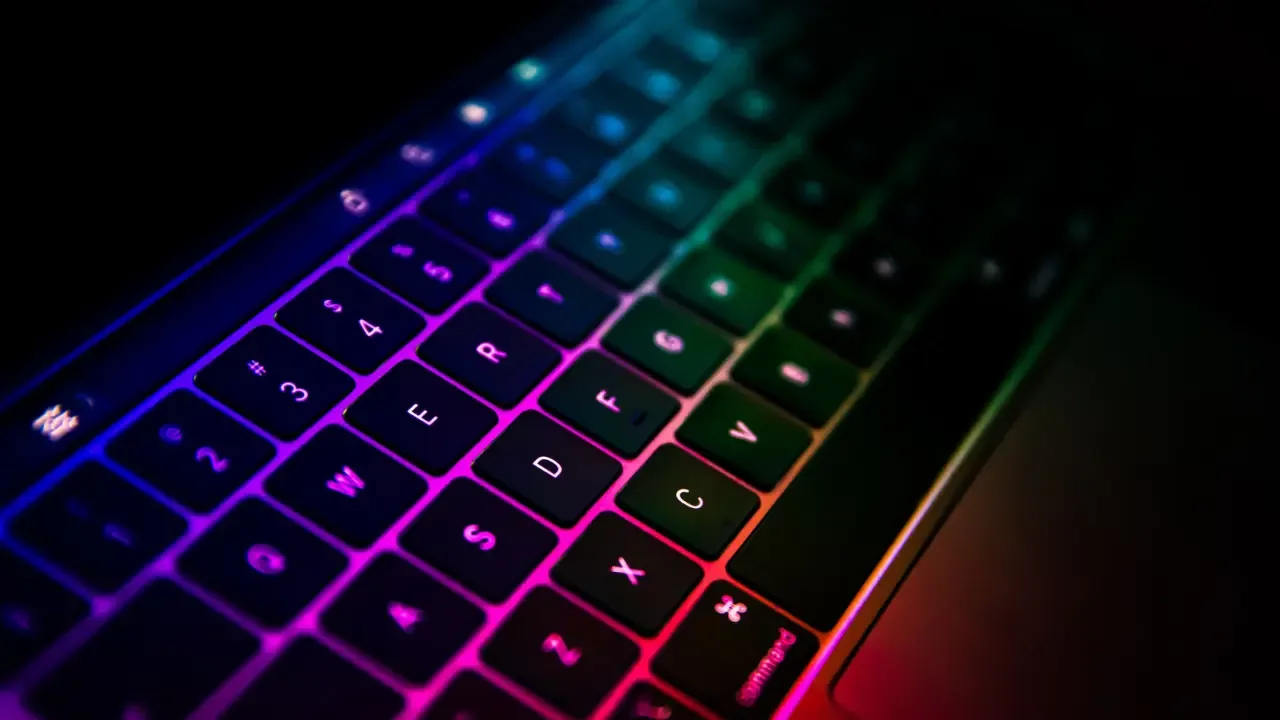
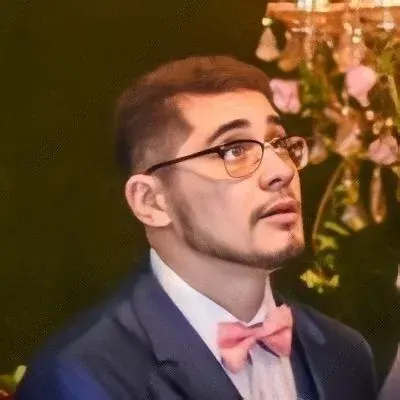
Laravel Migration: Unique Key Is Too Long, Even If Specified 😱
So, you're trying to migrate a users
table in Laravel, but when you run the migration, an error pops up, saying:
[Illuminate\Database\QueryException] SQLSTATE[42000]: Syntax error or access violation: 1071 Specified key was too long; max key length is 767 bytes (SQL: alter table users add unique users_email_uniq(email))
You've done some googling and found a bug report where Taylor, the creator of Laravel, suggested specifying the index key as the second parameter of unique()
, just like you did. But the error persists. What's happening here? 🤔
Understanding the Issue 🧐
The error message you're seeing is related to the maximum key length in your database. It appears that the maximum key length for your database is set to 767 bytes. This limit is often encountered when using the InnoDB storage engine with the default configuration in MySQL.
In Laravel, the unique()
method creates a unique index on a column. By default, Laravel uses the InnoDB storage engine, which has a maximum key length of 767 bytes when used with the utf8mb4 character set. This limitation affects columns with a length of more than 255 characters, like your email
column with a length of 320 characters.
Easy Solutions 🛠️
Luckily, there are a few simple workarounds to this issue:
Solution 1: Update Your Configuration 🛠️
One way to solve this problem is by updating your MySQL configuration file.
Locate your
my.cnf
ormy.ini
file, depending on your operating system.Open the file in a text editor and find the
[mysqld]
section.Add the line
innodb_large_prefix=true
to enable large index prefixes.Save the file and restart your MySQL server.
This solution allows you to increase the maximum key length and avoid the error.
Solution 2: Modify Your Migration 🛠️
If modifying the MySQL configuration is not an option for you, you can modify your migration to use a shorter key length.
Instead of using 320
as the length for the email
column, reduce it to a smaller value, such as 191
. This change ensures that the key length remains within the maximum limit.
$table->string('email', 191);
By making this adjustment, you should be able to migrate your table without encountering any errors.
The Compelling Call-To-Action 📢
Now that you understand the solution, it's time to take action! Choose the method that suits you best and implement it in your project. And if you found this guide helpful, make sure to share it with your developer friends who might encounter the same issue. Sharing is caring! ❤️
If you have any questions or need further assistance, feel free to leave a comment below. Let's conquer this migration hiccup together! 🚀