Laravel Middleware return variable to controller
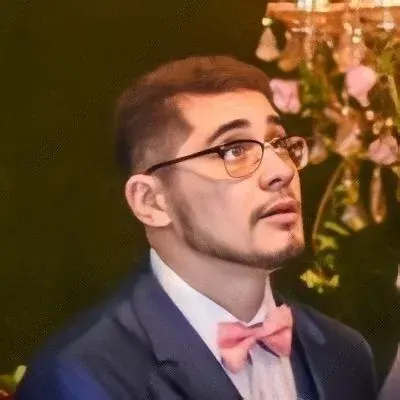
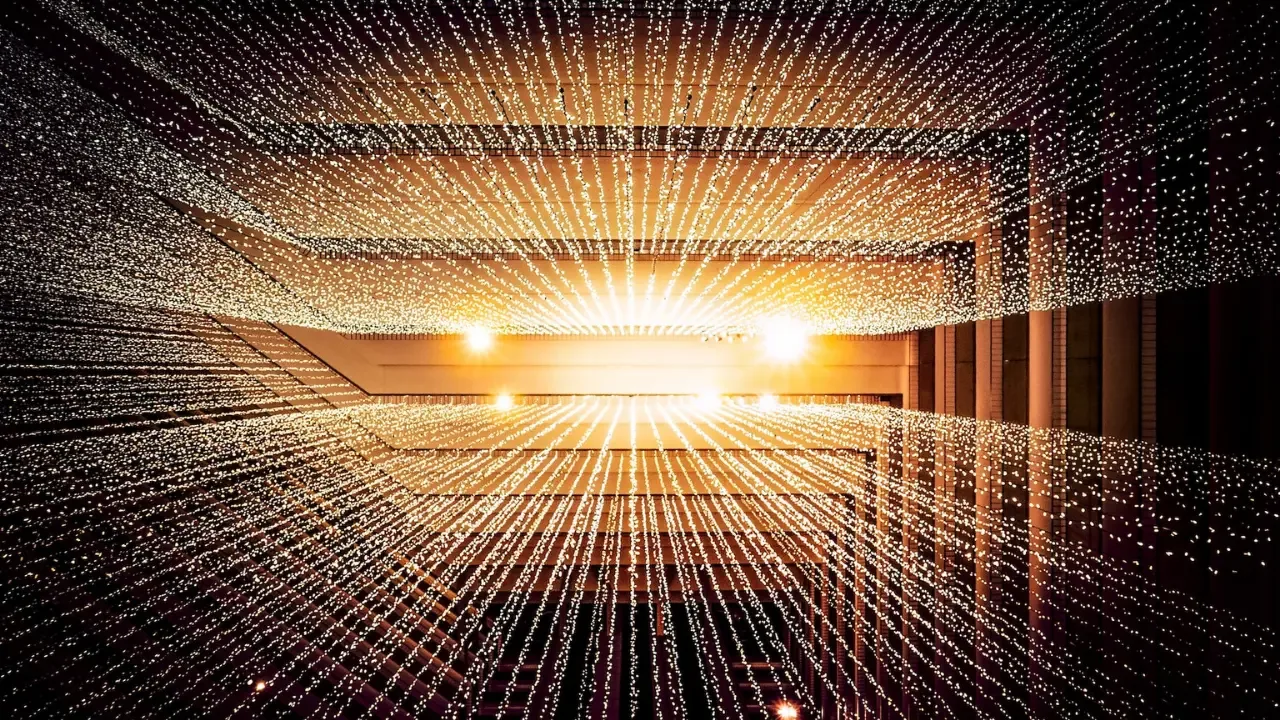
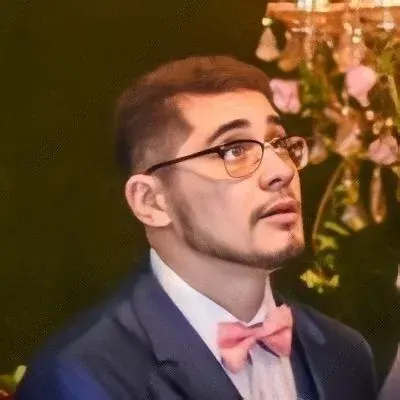
Laravel Middleware: Passing Variables to Controller
Are you facing the challenge of duplicating database queries between your Laravel middleware and controller? 🔄 Don't worry, we've got you covered! In this blog post, we'll guide you through the process of passing variables from middleware to a controller, simplifying your code and avoiding duplication. 💪
The Problem
Let's set the stage: you're running a permissions check in a Laravel application to determine if a user can view a specific page. You've implemented a middleware to handle this check before the request reaches the controller. However, you find yourself duplicating a database query in both the middleware and the controller before returning the data to the view. 🔄
Let's take a closer look at the example setup provided:
routes.php
Route::get('pages/{id}', [
'as' => 'pages',
'middleware' => 'pageUser',
'uses' => 'PagesController@view'
]);
PageUserMiddleware.php (class PageUserMiddleware)
public function handle($request, Closure $next)
{
//get the page
$pageId = $request->route('id');
//find the page with users
$page = Page::with('users')->where('id', $pageId)->first();
//check if the logged-in user exists for the page
if(!$page->users()->wherePivot('user_id', Auth::user()->id)->exists()) {
//redirect them if they don't exist
return redirect()->route('redirectRoute');
}
return $next($request);
}
PagesController.php
public function view($id)
{
$page = Page::with('users')->where('id', $id)->first();
return view('pages.view', ['page' => $page]);
}
As you can see, the code snippet Page::with('users')->where('id', $id)->first()
is duplicated in both the middleware and the controller. 🔄 This duplication is not only inefficient but also increases the risk of errors and makes your code harder to maintain.
The Solution: Passing Variables
To eliminate this duplication, we can pass the retrieved page variable from the middleware to the controller. By doing so, we can avoid repeating the same query and enhance the overall code efficiency. 💡 Here's how you can achieve this:
Step 1: Middleware
First, let's modify the middleware to store the page variable before passing it to the controller. We can achieve this by adding the variable as an attribute to the $request
object.
public function handle($request, Closure $next)
{
$pageId = $request->route('id');
$page = Page::with('users')->where('id', $pageId)->first();
if(!$page->users()->wherePivot('user_id', Auth::user()->id)->exists()) {
return redirect()->route('redirectRoute');
}
$request->attributes->set('page', $page); // Store page variable in `$request`
return $next($request);
}
Step 2: Controller
Now that the page variable is stored in the request, we can access it in the controller without duplicating the query.
public function view($id)
{
$page = request()->get('page'); // Retrieve the page variable from request
return view('pages.view', ['page' => $page]);
}
And that's it! 🎉 You have successfully passed the page variable from middleware to the controller. By following this approach, you have eliminated duplication and improved the efficiency of your code.
Share Your Thoughts!
We hope this guide has helped you solve the challenge of passing variables from Laravel middleware to controllers. But our journey doesn't end here! We're eager to hear about your experiences and the creative ways you apply this solution. Share your thoughts, code snippets, and any additional tips in the comments below. Let's learn from each other and make our Laravel development journey even more exciting! 🚀
So, what are you waiting for? Go ahead and implement this solution in your Laravel application. Don't forget to share your success stories and join the discussion in the comments section. We can't wait to hear from you! 😊