Laravel Eloquent where field is X or null
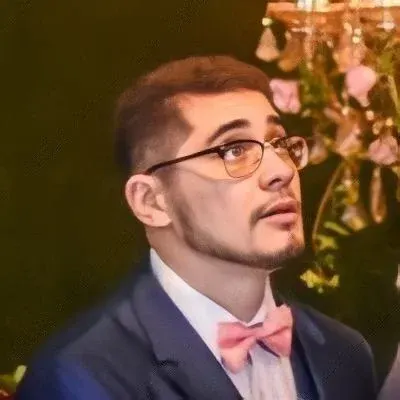
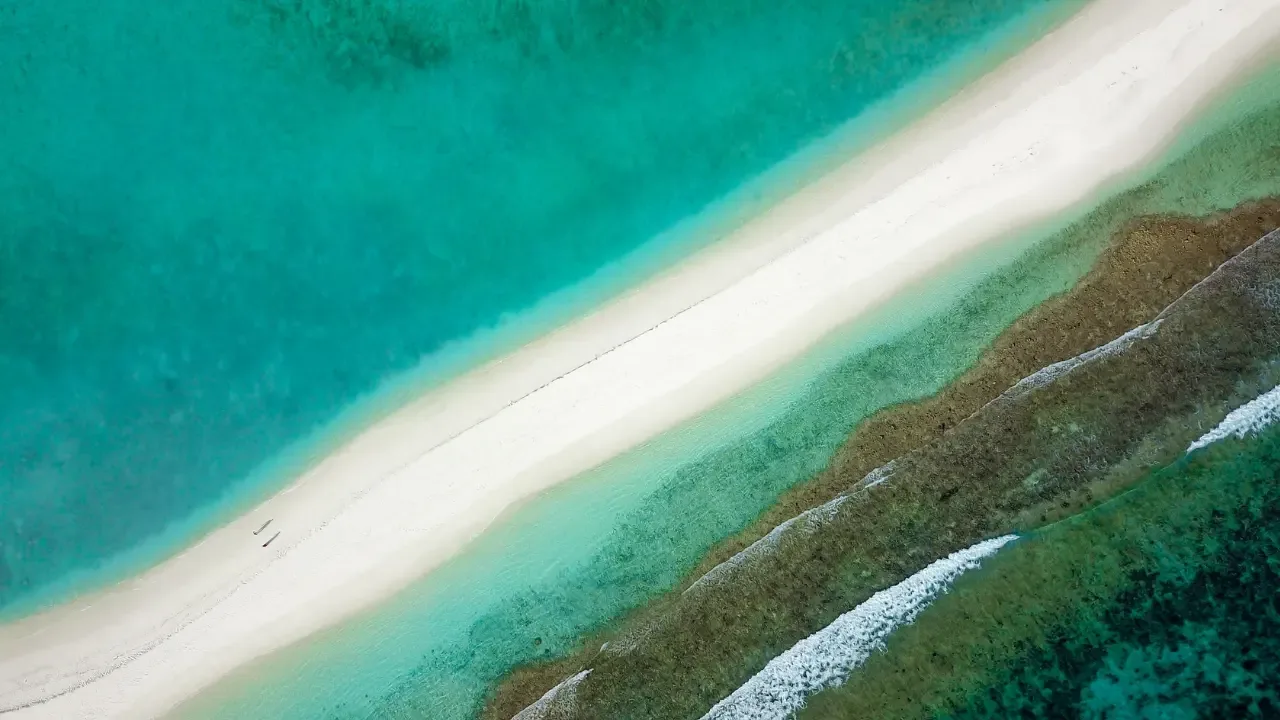
š Title: Mastering Laravel Eloquent: Filtering Results with Nullable Fields
š” Introduction: Are you struggling to filter your Laravel Eloquent results when dealing with nullable fields? Fear no more! In this guide, we'll tackle a common issue faced by developers and provide you with easy solutions to achieve the desired results. Whether you want to fetch all entries where a field is X or null, we've got you covered!
š Understanding the Problem:
Let's start by dissecting the problem at hand. You have a table with three fields: field1
, field2
(nullable), and datefield
(nullable). Your goal is to retrieve records where field1
is 1, field2
is null, and datefield
is smaller than X or null.
š§ The Initial Approach: Initially, you attempted using the following code snippet:
$query = Model::where('field1', 1)
->whereNull('field2')
->where('datefield', '<', $date)
->orWhereNull('datefield');
However, this approach did not produce the desired results. All entries with a null datefield
were returned, regardless of the values of field1
and field2
. Splitting the query into two separate queries yielded the same outcome.
š The Explanation: To understand why your initial approach fails, we need to dive into the conditions you've specified. Consider the following breakdown:
where('field1', 1)
: This condition filters records wherefield1
equals 1 (working as expected).whereNull('field2')
: This condition filters records wherefield2
is null (working as expected).where('datefield', '<', $date)
: This condition filters records wheredatefield
is smaller than X (working as expected).orWhereNull('datefield')
: This condition returns records wheredatefield
is null, regardless of the previous conditions (leads to undesired results).
As we can see, the orWhereNull
clause has a global effect, overriding the previous conditions and returning all records with a null datefield
.
š” The Solution: To overcome this challenge, we'll introduce a series of nested clauses using the power of Laravel Eloquent. Here's the updated code snippet:
$query = Model::where('field1', 1)
->whereNull('field2')
->where(function ($query) use ($date) {
$query->where('datefield', '<', $date)
->orWhereNull('datefield');
});
š Explanation of the Solution:
The key to achieving the desired results lies in the introduction of a nested clause. By encapsulating the where
and orWhereNull
conditions within a closure, we ensure that they are evaluated as a single unit. This prevents the orWhereNull
condition from overriding the earlier filters.
šŖ Call-to-Action: Congratulations! You have mastered the art of filtering Laravel Eloquent results when working with nullable fields. Start enhancing your application's querying capabilities and share your success stories with us in the comments below. If you found this guide helpful, don't forget to share it with your fellow developers!
š Conclusion: Filtering Laravel Eloquent results with nullable fields can be challenging, but with a clever approach, you can achieve the desired results. By understanding the problem, identifying the limitations of certain clauses, and utilizing nested clauses, you can overcome the hurdles. Keep exploring Laravel's powerful features, and empower your application with efficient querying techniques.
Now go ahead and fetch those records like a pro! š
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
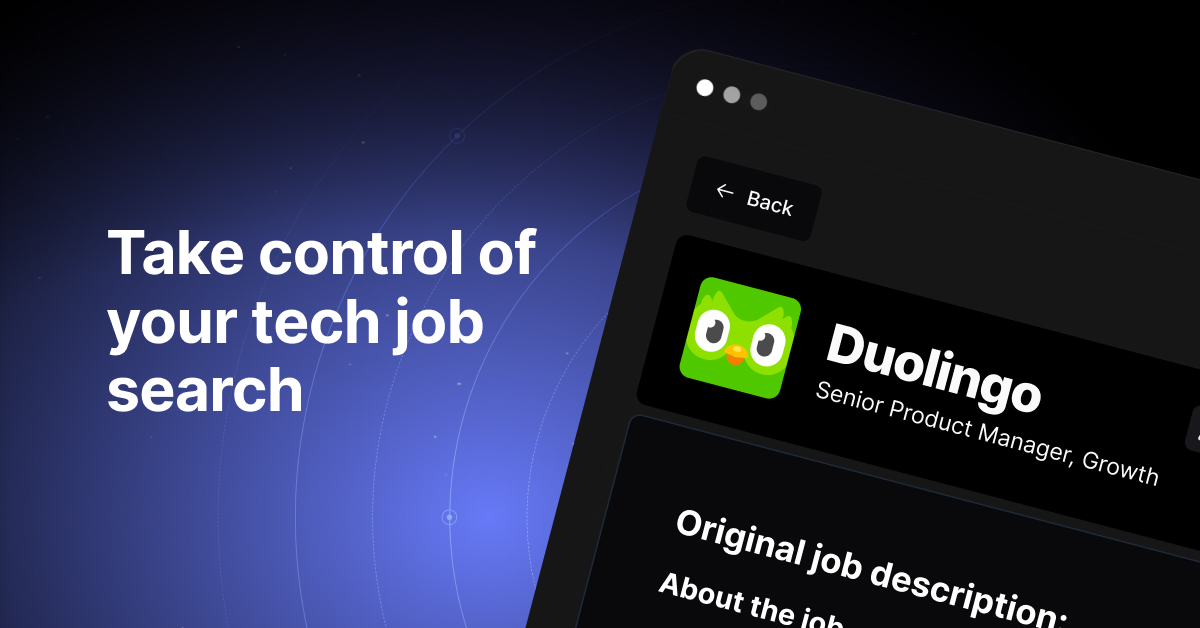