Laravel Eloquent update just if changes have been made
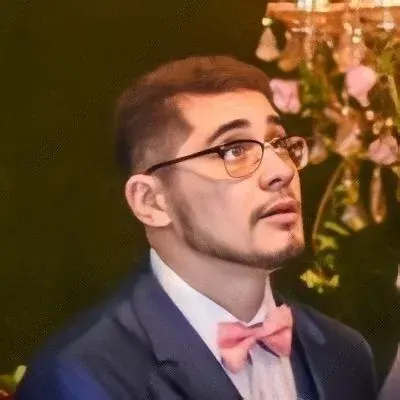
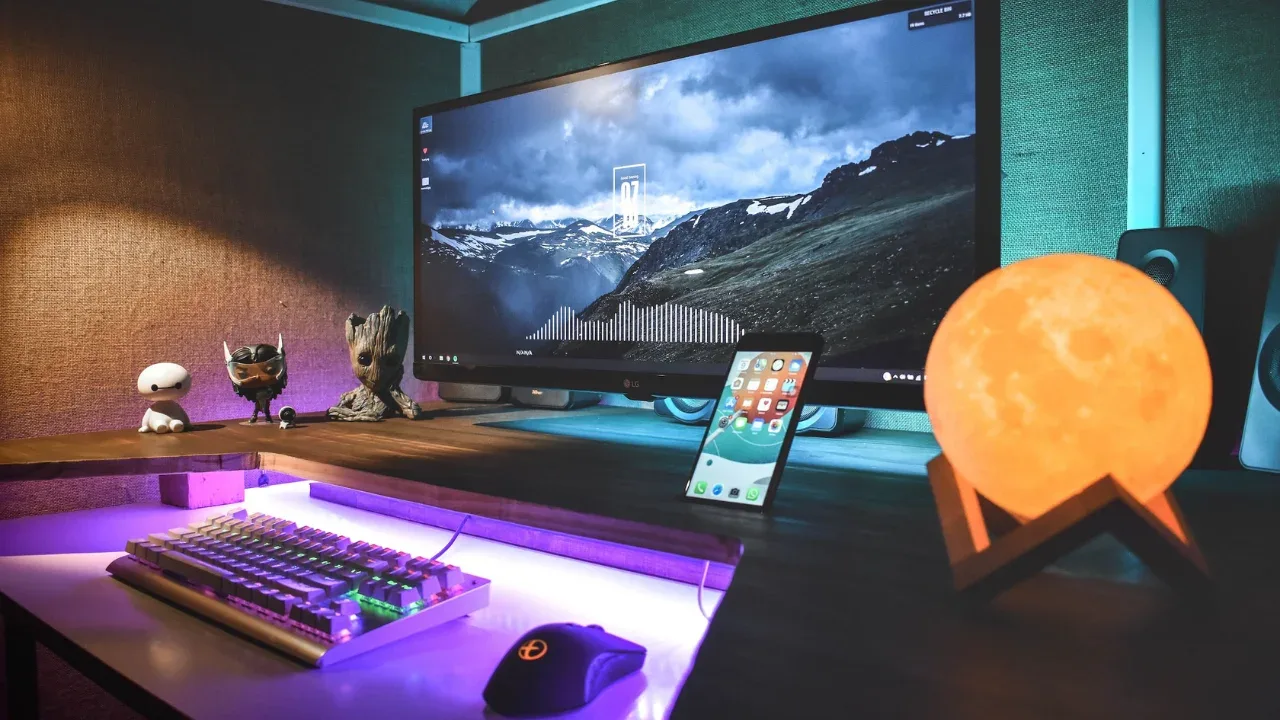
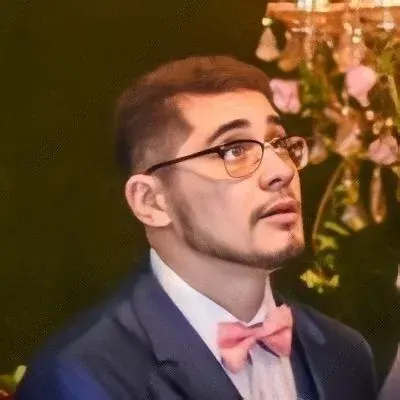
Updating Laravel Eloquent Models Only When Changes are Made 😮💾
Do you ever find yourself continuously hitting that save button, even when no changes have been made to your record? 🔄 Don't waste your precious server resources on unnecessary database requests! In this blog post, we'll explore how you can leverage Laravel Eloquent to update records only if changes have actually been made. 🚀
The Challenge: Avoiding Unnecessary Database Requests
So let's set the scene here: you have a Laravel application, and you want to update a record using Eloquent models, but you only want to do so if there have been actual changes made to that record. 📝💥
Usually, you would update a record like this:
$product = Product::find($data["id"]);
$product->title = $data["title"];
$product->description = $data["description"];
$product->price = $data["price"];
// etc (string values were previously sanitized for XSS attacks)
$product->save();
The issue here is that every time this code executes, regardless of whether there were any changes, it will trigger a database update. 😱 This means unnecessary overhead and wasted resources on both the server and the database. Not cool, right? 🙅♀️
The Elegant Solution: The isDirty
Method
Laravel Eloquent provides a handy method called isDirty()
that allows us to check if a model has been modified. 💡
Here's how you can modify your code to leverage this cool functionality:
$product = Product::find($data["id"]);
if ($product->isDirty()) {
$product->title = $data["title"];
$product->description = $data["description"];
$product->price = $data["price"];
// etc
$product->save();
}
Now, with a simple conditional check, we will only update the record if changes have actually been made. 👍✨
The isDirty()
method does all the hard work for you. It compares the model's attributes with their original values to determine if any changes have occurred. If there are changes, the method will return true
; otherwise, it will return false
.
Why You'll Love This Approach
By utilizing the isDirty()
method, you can dramatically reduce the number of unnecessary database requests and improve the performance of your application. 📈💪 No more wasting valuable server resources on pointless updates!
💡 Pro Tip: You can also use the isDirty
method on individual attributes. For example, if you only want to check whether the title
attribute has changed, you can call $product->isDirty('title')
.
Take It to the Next Level: Automate with Events
If you want to take this concept even further and automate the process, Laravel provides a powerful feature called events. 🚀🎉 By leveraging events, you can listen for updates to your model and perform actions only when changes occur.
Check out the Laravel documentation on events to explore how you can add custom listeners and automate actions based on changes made to your Eloquent models!
Conclusion and Your Turn!
Updating Laravel Eloquent models while avoiding unnecessary database requests is a breeze with the isDirty()
method. 👏💻 Be smart about resource utilization and ensure your code only updates records when changes have been made. Your server and database will thank you! 😄💾
Now it's your turn to give it a try! Update your code snippets and watch those extra database requests fade away. If you have any questions or cool tips to share, drop them in the comments below. Let's optimize together! 🙌🎯