Laravel Eloquent Sum of relation"s column
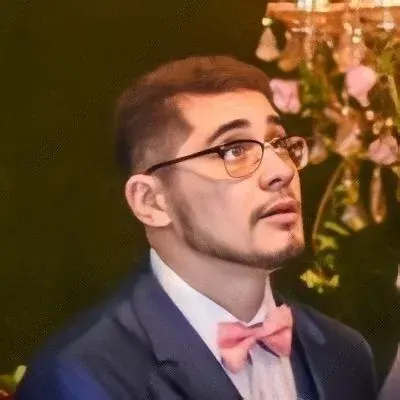
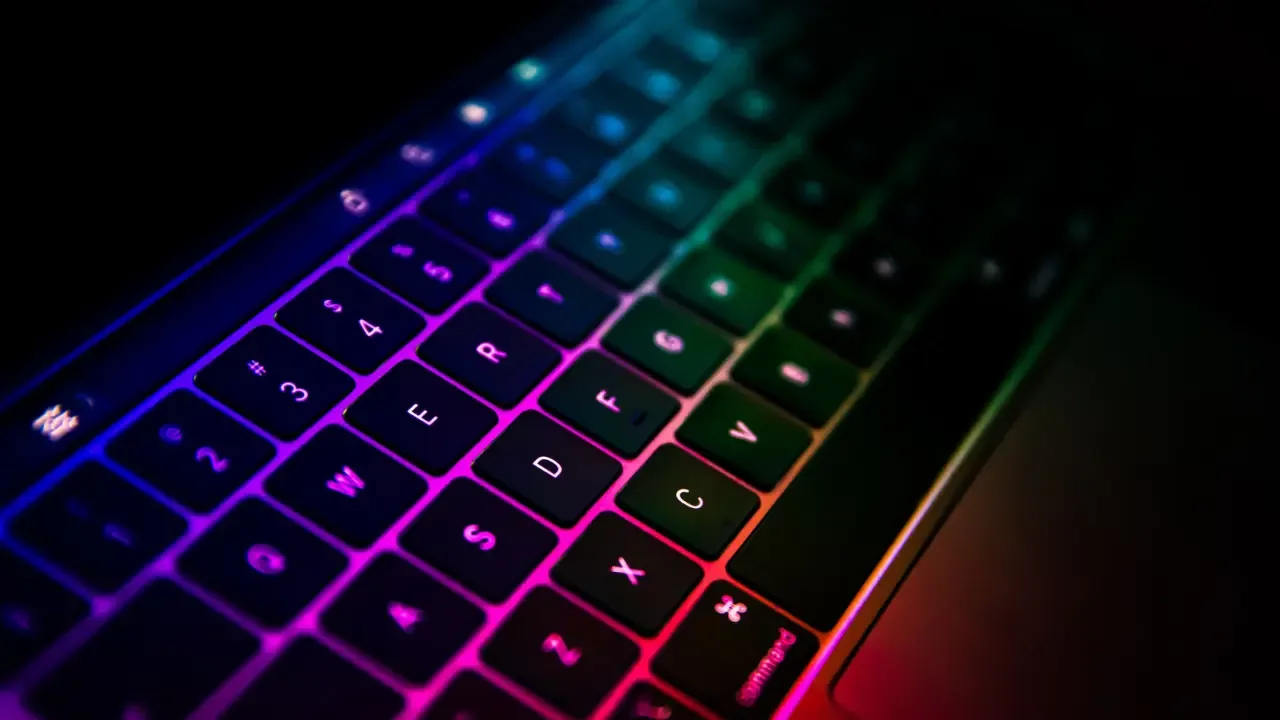
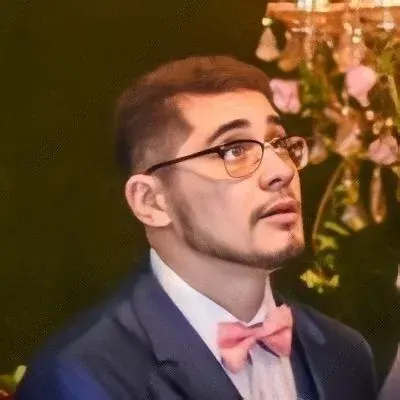
Laravel Eloquent Sum of relation's column
š Welcome to another exciting blog post! Today, we're going to tackle a common issue in Laravel: calculating the sum of a related column using Eloquent. š§®
The Scenario
š Let's imagine we have a shopping cart application with three main objects: User, Product, and Cart. The Cart table only contains the following columns: id
, user_id
, product_id
, and timestamps. The relationships are set up as follows:
The User model
hasMany
Carts because a user can store multiple products.The Cart model
belongsTo
a User andhasMany
Products.
The Challenge
š¤ Now, here's the challenge: how can we calculate the sum of the prices (a column of product) of the products in the cart for a specific user? And how can we achieve this using the elegance of Laravel's Eloquent ORM? šŖ
The Eloquent Solution
⨠Fortunately, Laravel's Eloquent provides a straightforward solution to this problem. To calculate the sum of a related column, we can leverage the power of the withSum
method. Let's see how it's done!
$totalPrice = User::withSum('cart.products', 'price')->find($userId)->cart_sum_price;
š In the code above, we use the withSum
method to specify the relation to sum (cart.products
) and the column to sum (price
). cart_sum_price
will then contain the sum of the prices for the products in the user's cart.
An Example
š Let's illustrate this with an example. Assuming we have a user with ID 1, let's calculate the sum of the prices for their cart's products:
$userId = 1;
$totalPrice = User::withSum('cart.products', 'price')->find($userId)->cart_sum_price;
š° The $totalPrice
variable will now hold the sum of the prices for the products in the user's cart!
Why Choose Eloquent?
š You might wonder why we would choose Eloquent instead of writing a raw query. Well, one of the main advantages is that Eloquent keeps our code cleaner and more maintainable. Instead of juggling with complex query syntax, we can let Eloquent do the heavy lifting and focus on writing beautiful, readable code. š
𤩠Imagine being able to effortlessly calculate the sum of a related column using just a single Eloquent method call! It truly adds magic to our workflow. āØ
Get Summing Today!
š Now that you know how to calculate the sum of a related column using Laravel's Eloquent, it's time to put it into action! š„ Start utilizing this powerful feature to enhance your shopping cart application or any other project that requires summing related columns.
š¬ Share your thoughts, experiences, and any other techniques you've used to tackle similar problems in the comments below. Let's learn from each other and build great Laravel applications together!
š Happy coding with Eloquent! āØ