Laravel - Eloquent or Fluent random row
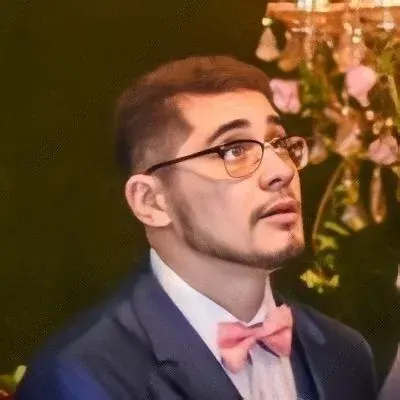
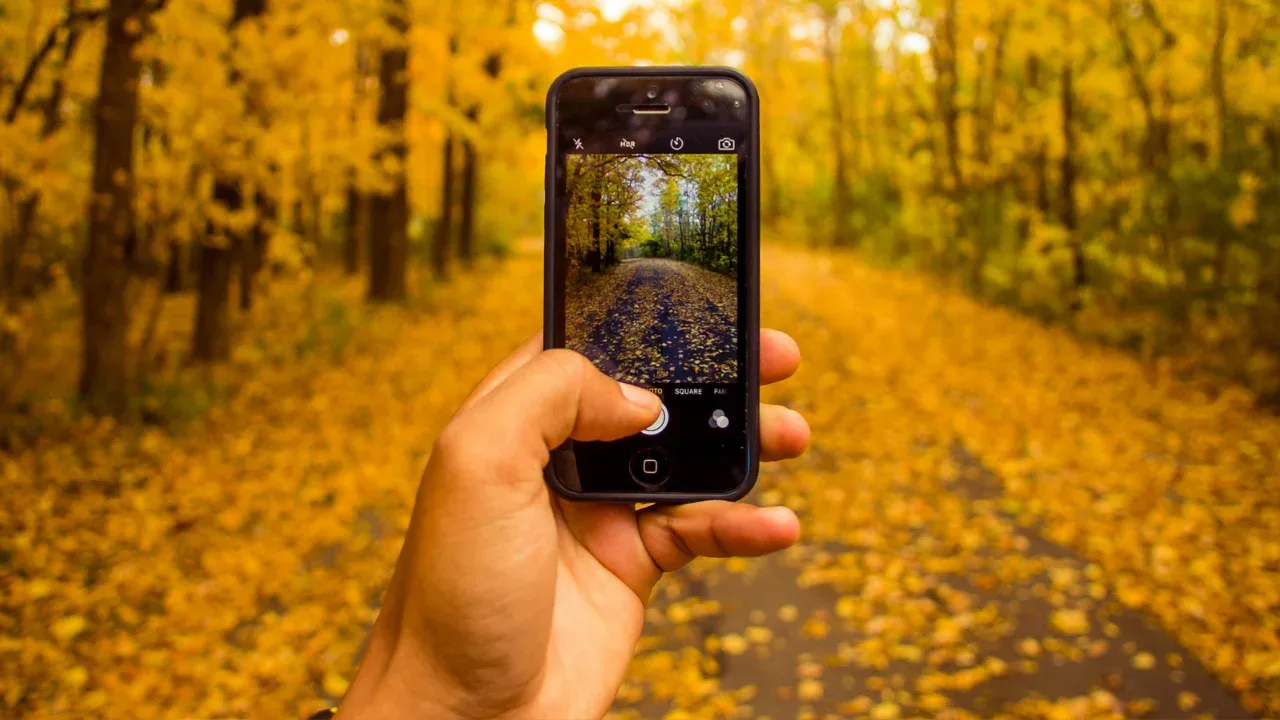
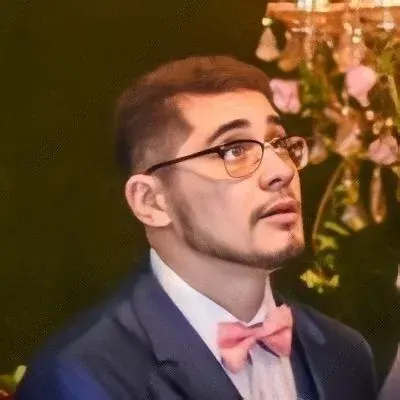
Laravel - Eloquent vs. Fluent: Unlocking the Mystery of Random Rows π€π
So, you're working on a Laravel project and you find yourself in a bit of a pickle π₯. You need to select a random row from your database using either Eloquent or Fluent, but you're scratching your head π€ trying to figure out the best approach. Fear not, dear reader, for I have the answers you seek!
The Problem: Random Rows and SQL Queries ππ²
The question at hand is pretty straightforward. You want to select a random row from your database without having to perform a count on the number of records before making the query. And while you know that using SQL, you could simply do an ORDER BY RAND()
, Laravel offers two different query builders to choose from - Eloquent and Fluent - adding a layer of confusion to the mix.
The Solution: Eloquent's inRandomOrder()
and Fluent's inRandomOrder()
πΊπ
Luckily for us, Laravel provides handy methods in both Eloquent and Fluent that make selecting random rows a breeze! Let's explore these solutions step-by-step:
Using Eloquent - The Eloquent Way π
If you're using Eloquent, the Laravel ORM (Object-Relational Mapping), you're in for a treat! Laravel offers a super simple way to fetch random rows using the inRandomOrder()
method.
Here's how it works:
$randomRow = Model::inRandomOrder()->first();
That's it! π By chaining the inRandomOrder()
method to your query builder, you will get a randomly ordered result set. To retrieve just one random row, we can call the first()
method at the end of the query.
Using Fluent - The Fluent Way π
If you prefer to use Fluent, Laravel's database query builder, never fear! Fluent also rocks a inRandomOrder()
method that does the trick.
Here's an example:
$randomRow = DB::table('your_table')->inRandomOrder()->first();
Just like with Eloquent, you simply chain the inRandomOrder()
method to your Fluent query. The first()
method fetches only one random row from the result set.
The Call-to-Action: Share your Randomness! π’β
Now that you know how to fetch random rows effortlessly using Laravel, it's time to put your newfound knowledge to the test! Try implementing it in your own code and see how it feels. And hey, if you happen to stumble upon any cool use cases or have some tricks up your sleeve, share them with us in the comments below! Let's embrace the randomness and learn from one another. Happy coding! π»π
Are you struggling with any other Laravel queries? Check out our blog for more helpful tips and tricks!