Laravel Check If Related Model Exists
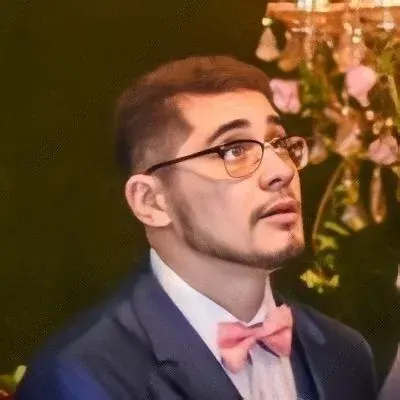
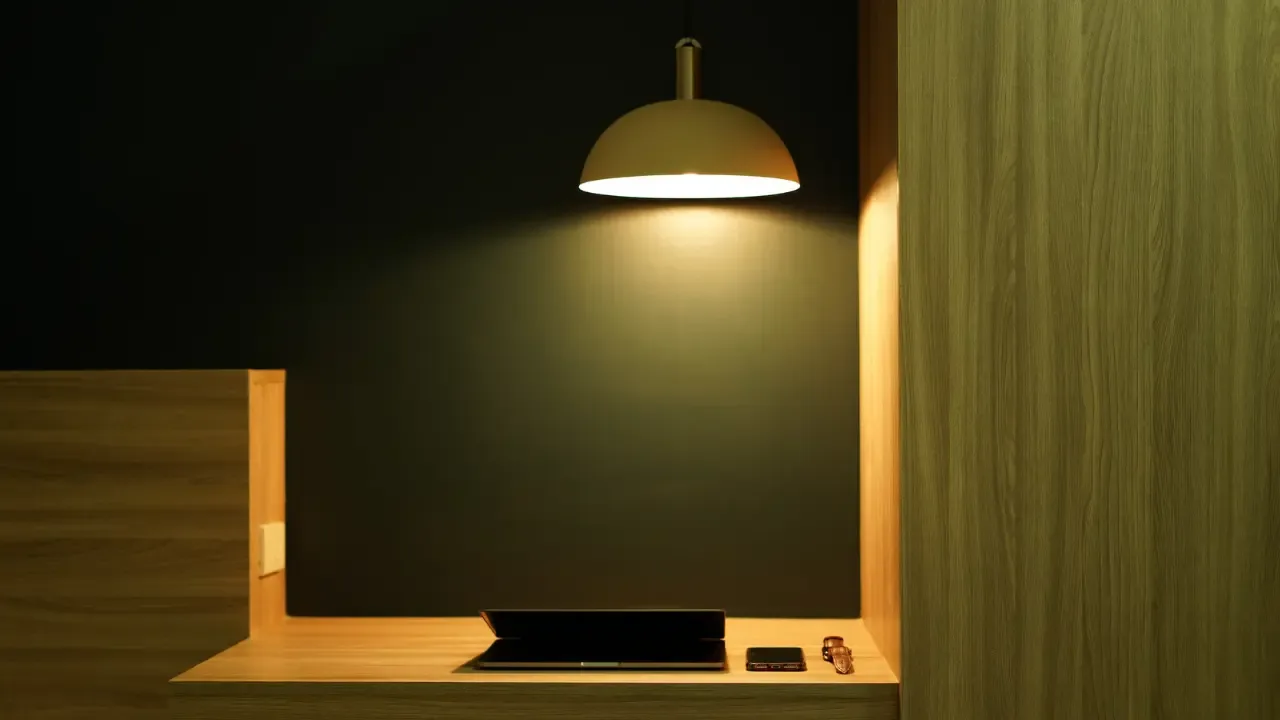
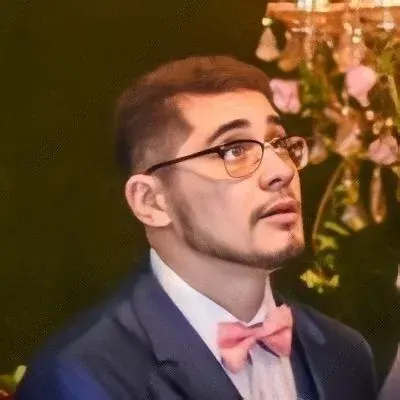
Laravel Check If Related Model Exists
š Have you ever encountered the problem of checking if a related model exists in Laravel? It can be quite tricky but don't worry, I'm here to help you! In this article, I will guide you through common issues and provide easy solutions to solve this challenge.
The Problem
š” Let's first understand the problem at hand. You have an Eloquent model with a related model, like this:
public function option() {
return $this->hasOne('RepairOption', 'repair_item_id');
}
public function setOptionArrayAttribute($values)
{
$this->option->update($values);
}
š¤ The issue arises when you need to check if the related model exists. You want to either update the existing model or create a new one depending on the scenario. Your code snippet looks like this:
$model = RepairItem::find($id);
if (Input::has('option')) {
if (<related_model_exists>) {
// Create a new model
} else {
// Update the existing model
}
}
ā The missing piece of the puzzle is the code to determine if the related model exists or not.
The Solution
š” To solve this problem, Laravel provides a convenient method called exists()
that you can use to check if a related model exists. Let's modify your code to incorporate this solution:
$model = RepairItem::find($id);
if (Input::has('option')) {
if ($model->option->exists()) {
// Create a new model
} else {
// Update the existing model
}
}
š By simply using the exists()
method on the related model, you can now determine if it exists or not. This allows you to take the appropriate action based on your needs.
ā Here's a breakdown of the updated code:
Retrieve the main model using
RepairItem::find($id)
.Check if the "option" input is present using
Input::has('option')
.Use the
exists()
method on the related model to determine if it exists or not.Perform the desired action based on the result.
š That's it! You have successfully resolved the issue of checking if a related model exists in Laravel.
Conclusion
š In this article, we explored the problem of checking if a related model exists in Laravel. We learned that Laravel provides a convenient solution with the exists()
method. By implementing this solution, you can easily determine if a related model exists and take the appropriate action.
šŖ Now that you know how to handle this challenge, go ahead and apply it to your own projects. Happy coding! š
⨠If you have any questions, feel free to leave a comment below. Let's discuss and learn together!