Laravel: Auth::user()->id trying to get a property of a non-object
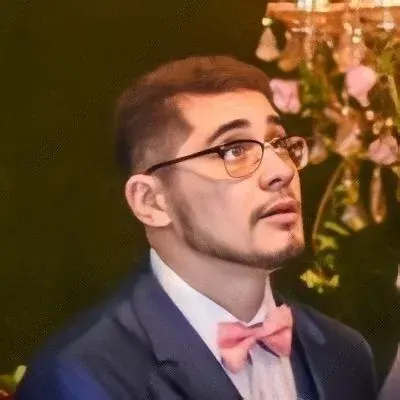
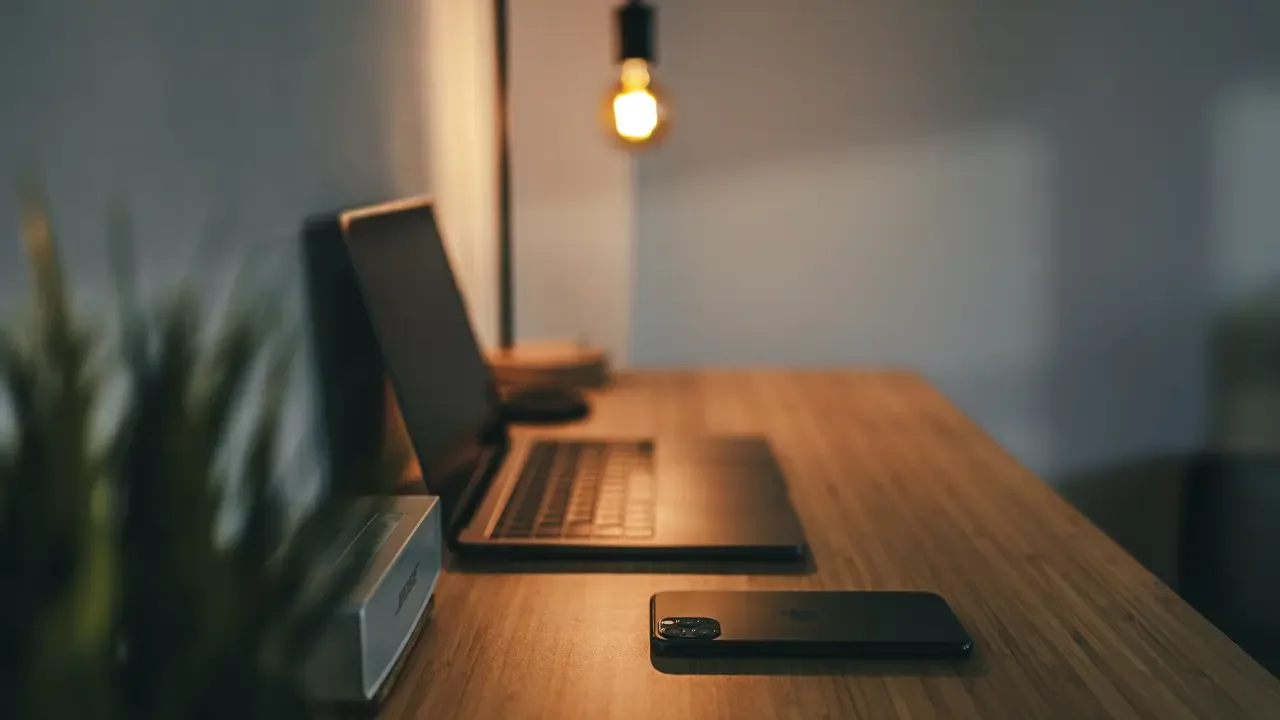
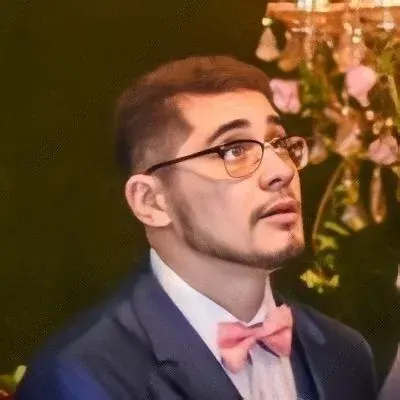
ππ§Laravel: Auth::user()->id trying to get a property of a non-object π«π
Are you encountering the error "trying to get a property of a non-object" in Laravel when trying to retrieve the user ID using Auth::user()->id
? You're not alone! This common issue often occurs when there is no authenticated user, resulting in Auth::user()
returning null instead of an instance of the User model.
π Understanding the Problem
In the provided code snippet, the error occurs on the first line where you try to access the id
property of the Auth::user()
. This error message indicates that the Auth::user()
is not returning an object, meaning there is no authenticated user.
π‘ Easy Solutions Here are a few possible solutions to resolve this problem:
1οΈβ£ Check Authentication: Double-check if your user is properly authenticated before accessing the id
property. Laravel's Auth::check()
method can be used to determine if a user is logged in. Wrap your code within an if (Auth::check())
statement to ensure that the user is authenticated before accessing Auth::user()->id
.
β Example:
if (Auth::check()) {
$id = Auth::user()->id;
// Rest of your code
}
2οΈβ£ Middleware: Ensure that the route or controller where this code resides is protected with proper authentication middleware. This will ensure that only authenticated users can access it.
β Example:
Route::middleware('auth')->group(function () {
// Your routes or controllers here
});
3οΈβ£ Check Configuration: Verify that your authentication configuration is correctly set up. Ensure that you have properly configured the authentication driver, provider, and guard in your config/auth.php
file.
4οΈβ£ Debug the Sentry Configuration: Since you mentioned using the Sentry 2 authentication bundle, make sure you have correctly set up Sentry and configured it to work with your authentication setup. Review the documentation and verify if your User model extends the Sentry UserInterface
.
π£ Engage with Us! We hope these solutions help you fix the "trying to get a property of a non-object" error! If you have any further questions or run into any other Laravel-related issues, reach out to our community or leave a comment below. Let's help each other out! ππ¬
π Have you encountered this error before? Share your experience or any additional solutions you found!
Happy coding! π»π