Laravel 4: how to "order by" using Eloquent ORM
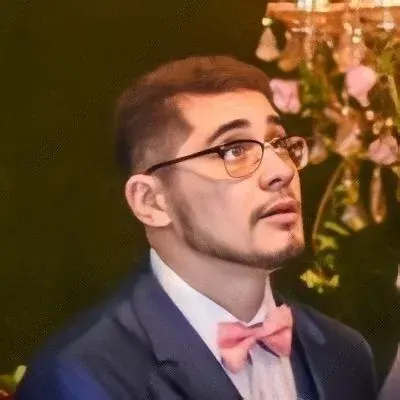
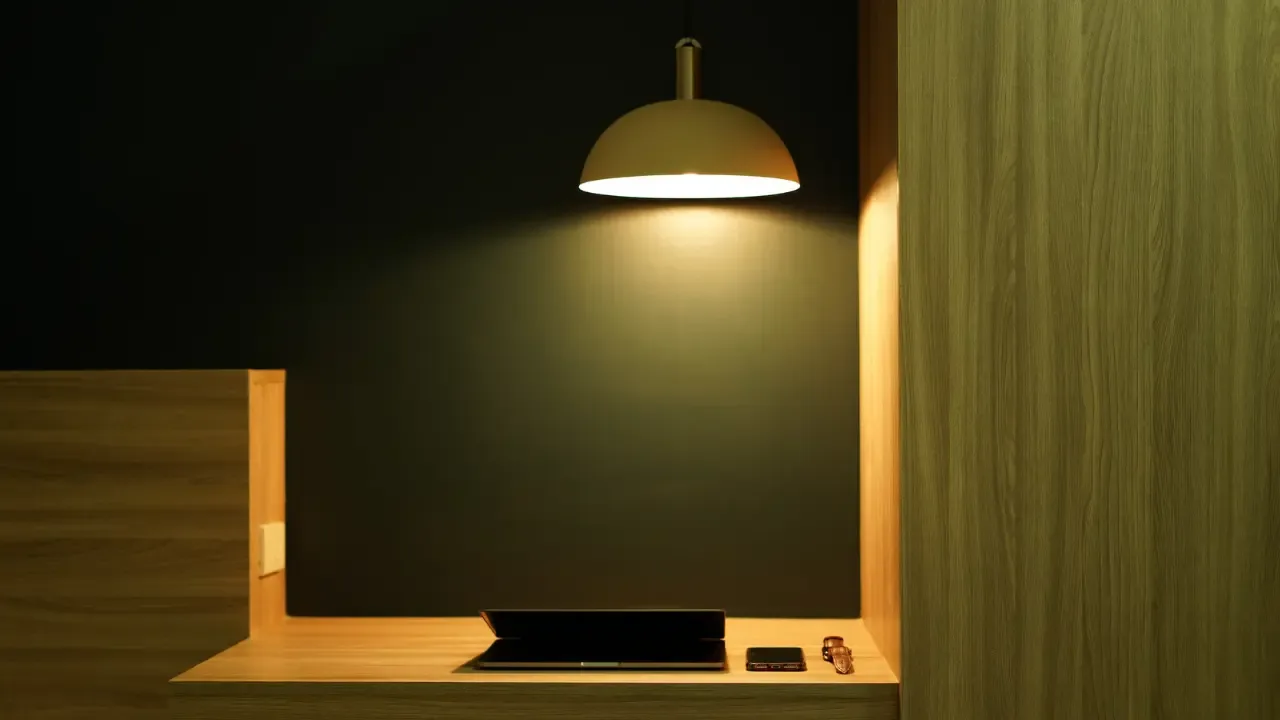
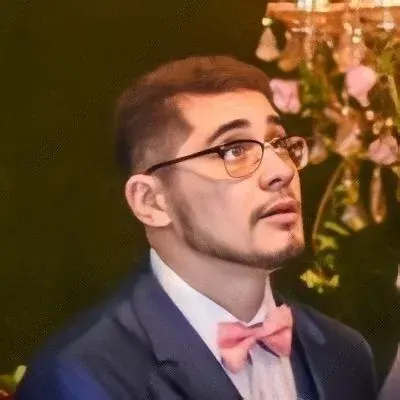
How to "Order By" using Laravel 4 Eloquent ORM 😎
So, you want to order your records in Laravel 4 using the Eloquent ORM? No worries, I got you covered! 🙌
The Problem 😕
You have a simple question: "How do I order by 'id' descending in Laravel 4?" You provided some context and mentioned your code, but you're not sure how the orderBy
method fits in. Let's break it down step by step! 💡
The Solution 💡
To order your records by the 'id' column in descending order, you need to make a small adjustment to your code. Here's what you need to do:
$posts = $this->post->orderBy('id', 'desc')->get();
In the above code, we modified your existing line by adding the orderBy
method call before the get
method. The 'id'
parameter indicates that you want to sort by the 'id' column, and the 'desc'
parameter ensures it's in descending order.
That's it! You're all set! Your records will now be ordered by 'id' in descending order. 👍
What if you want to order by multiple columns? 🤔
Let's say you want to order your records by 'id' in descending order first, and then by 'name' in ascending order. Here's how you can achieve that:
$posts = $this->post->orderBy('id', 'desc')->orderBy('name', 'asc')->get();
You can simply chain multiple orderBy
methods, each specifying the column and the sort direction.
Conclusion and Call-to-Action 🚀
Ordering records in Laravel 4 using the Eloquent ORM is now a piece of cake! 🍰 Take advantage of the orderBy
method and customize your sorting based on your needs. Whether it's a single column or multiple columns, Laravel makes it super easy for you. 😎
If you found this blog post helpful, feel free to share it with your friends who might be grappling with the same issue. And don't forget to leave a comment below if you have any further questions or suggestions. Happy coding! 💻