Is there any way to detect if a database table exists with Laravel
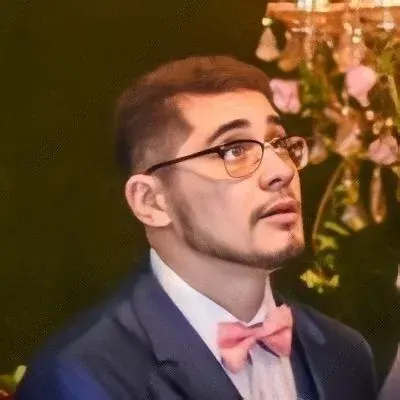
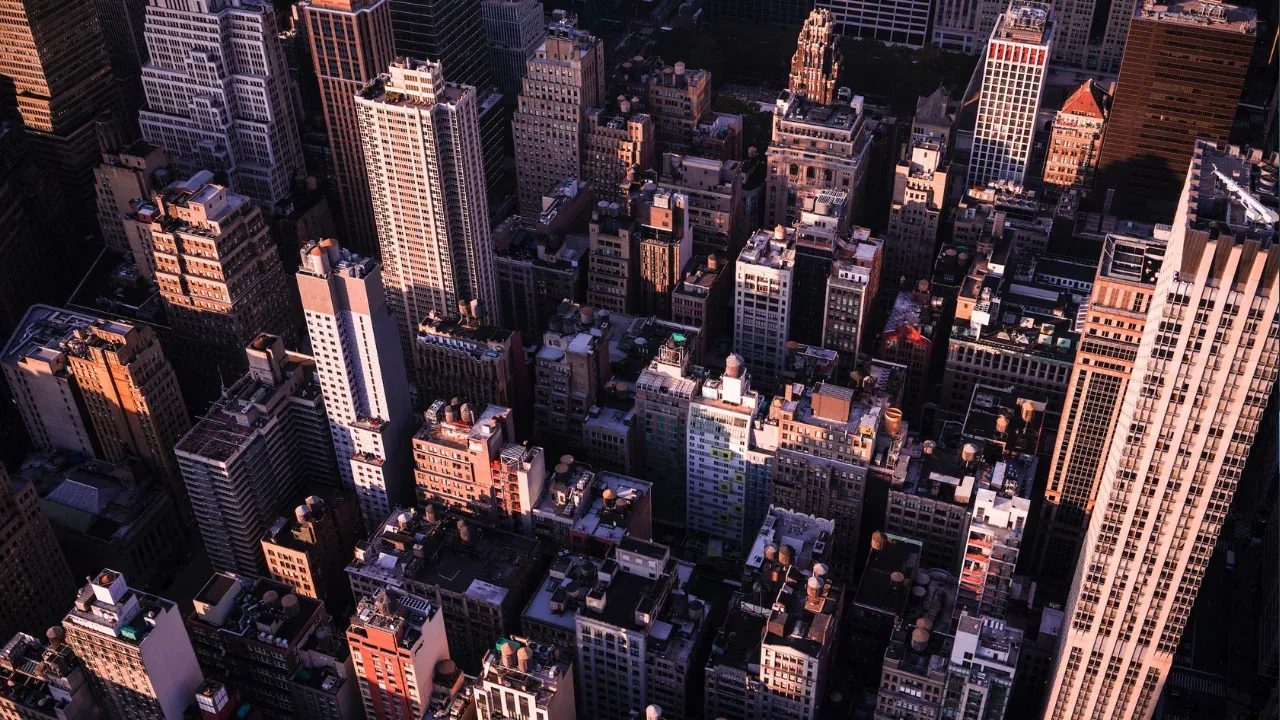
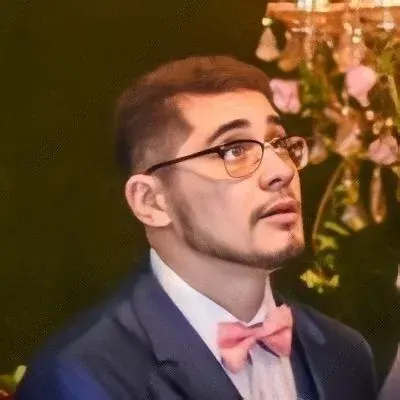
📖 How to Detect If a Database Table Exists with Laravel
So you want to create a table in your Laravel application, but before doing so, you want to check if the table already exists. You might be wondering if there's a built-in function like Schema::exists('mytable')
to handle this, but unfortunately, such a function doesn't exist. But don't worry! There are easy solutions to accomplish this task. Let's dive in! 💪🚀
The Challenge: Checking Table Existence
The question at hand is how to detect if a database table exists in Laravel. To solve this problem, we need to find an alternative method to check the existence of a table before attempting to create it.
Solution 1: Use the hasTable
Method
One way to check table existence is by using the hasTable
method provided by Laravel's Schema builder. This method allows you to verify if a table exists in the database. Here's an example:
use Illuminate\Support\Facades\Schema;
if (Schema::hasTable('mytable')) {
// Table exists, handle accordingly
} else {
// Table does not exist, proceed with creation
}
With this simple approach, you can easily determine whether a table exists or not before performing any relevant actions.
Solution 2: Use the listTableNames
Method
Another approach is to retrieve a list of all table names using the listTableNames
method and check if the desired table name exists in the array. Here's how you can do it:
use Illuminate\Support\Facades\Schema;
$tables = Schema::getConnection()->getDoctrineSchemaManager()->listTableNames();
if (in_array('mytable', $tables)) {
// Table exists, handle accordingly
} else {
// Table does not exist, proceed with creation
}
This method allows you to obtain a list of all table names in the database and then check if the desired table name exists within it.
Conclusion
Checking if a database table exists in Laravel is a common challenge faced by developers. Although Laravel doesn't provide a built-in function specifically for this task, we have explored two easy solutions that can help you address this issue effectively.
Whether you choose Solution 1 using the hasTable
method or Solution 2 using the listTableNames
method, the key takeaway is to ensure that your code handles both scenarios: when the table exists and when it doesn't.
So, go ahead and implement one of these solutions in your Laravel project and avoid any unwanted errors or conflicts during table creation!
Do you have any further questions or suggestions? Let us know in the comments below. Happy coding! 😄👩💻👨💻