Invalid argument supplied for foreach()
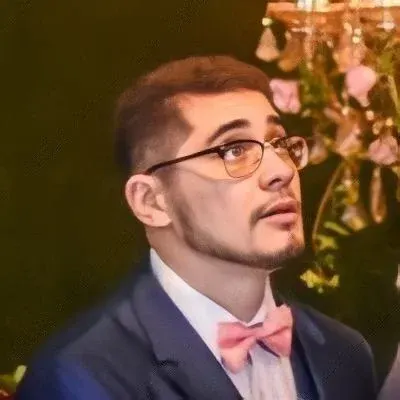

Invalid argument supplied for foreach(): How to Handle It?
šÆ Have you ever encountered the warning message "Invalid argument supplied for foreach()" in your PHP code? It's a common issue that arises when you try to iterate over a non-array variable with the foreach loop. But fret not, there are easy solutions to tackle this problem. In this blog post, we'll explore several approaches and guide you on choosing the cleanest and most efficient way to resolve this warning. Let's dive in! šāāļø
The Scenario
š Picture this: you have a data variable, let's call it $values
, which can be either an array or a null value. And you need to iterate over this variable using the foreach loop. Here's an example to illustrate the situation:
$values = get_values();
foreach ($values as $value){
...
}
Now, if get_values()
sometimes returns a non-array value (e.g., null), you will encounter the dreaded "Invalid argument supplied for foreach()" warning. š±
The Cleanest and Most Efficient Solutions
1ļøā£ Casting $values
to an Array
One way to handle this issue is by casting the variable $values
explicitly to an array. You can achieve this with the (array) type-casting operator. Here's how:
$values = (array) get_values();
foreach ($values as $value){
...
}
Casting ensures that even if $values
is not initially an array, it will be converted to an empty array. This allows the foreach loop to execute without triggering any warnings.
2ļøā£ Initializing $values
as an Array
Another approach is to initialize the variable $values
as an empty array before iterating over it. This can be done using the empty array syntax - []
. Take a look:
$values = get_values();
if (empty($values)) {
$values = [];
}
foreach ($values as $value){
...
}
By initializing $values
as an empty array when it's not an array, you ensure that the foreach loop won't complain about receiving an invalid argument.
3ļøā£ Wrapping the foreach
with an if
Statement
A third option is to explicitly check whether $values
is an array before entering the foreach loop. If it's not an array, you can gracefully handle the situation without triggering any warnings. Here's an example:
$values = get_values();
if (is_array($values)) {
foreach ($values as $value){
...
}
} else {
// Handle the non-array case gracefully
}
With this approach, you have full control over how to handle the non-array scenario without any unexpected warnings.
āļø Other Ideas?
Do you have any other suggestions or approaches to tackle this issue? We'd love to hear from you! Share your thoughts and ideas in the comments section below. Let's solve this problem together. š
Conclusion
ā”ļø You've made it to the end! Handling the "Invalid argument supplied for foreach()" warning is no longer a mystery for you. Today, we explored three clean and efficient solutions: casting to an array, initializing as an array, and wrapping with an if statement. Now, armed with this knowledge, you can confidently conquer this problem in your PHP projects. šŖ Remember to choose the solution that best fits your specific use case.
š Don't forget to share this blog post with your fellow developers who might also be struggling with this warning. And if you have any questions or need further assistance, feel free to reach out. Keep coding and keep conquering those bugs! šš„
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
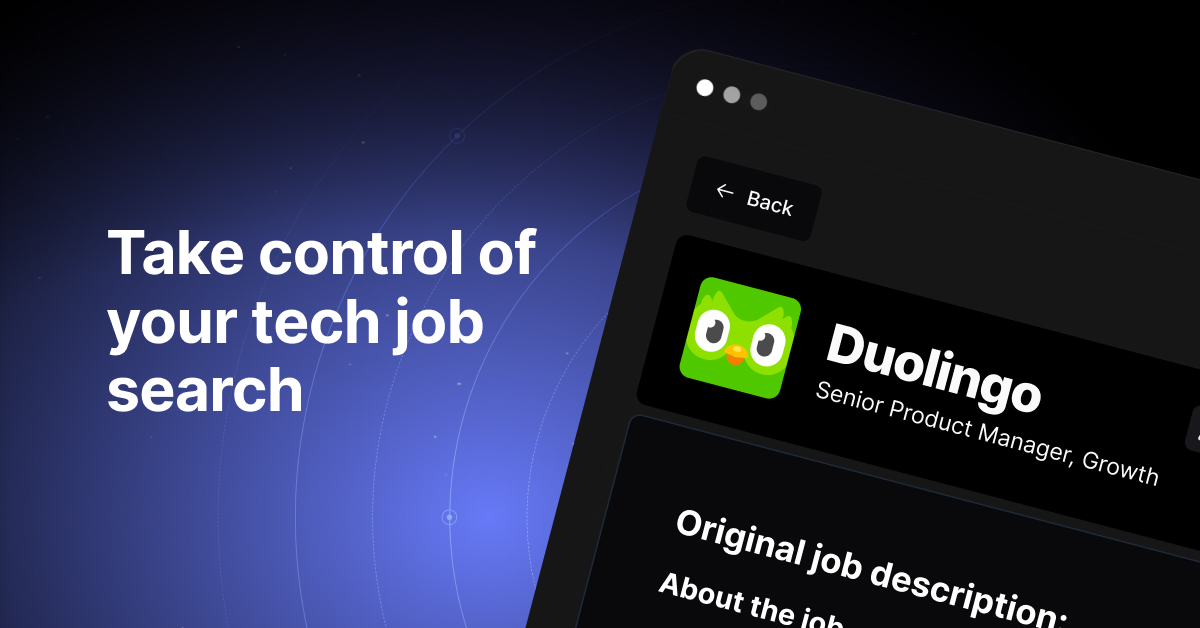