In Laravel is there a way to add values to a request array?
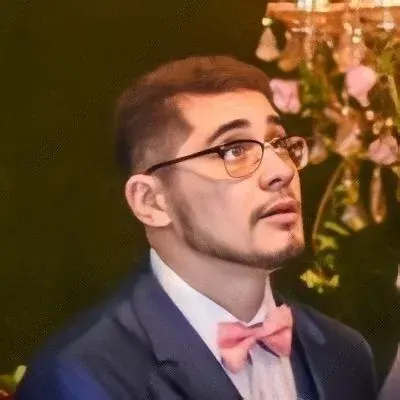
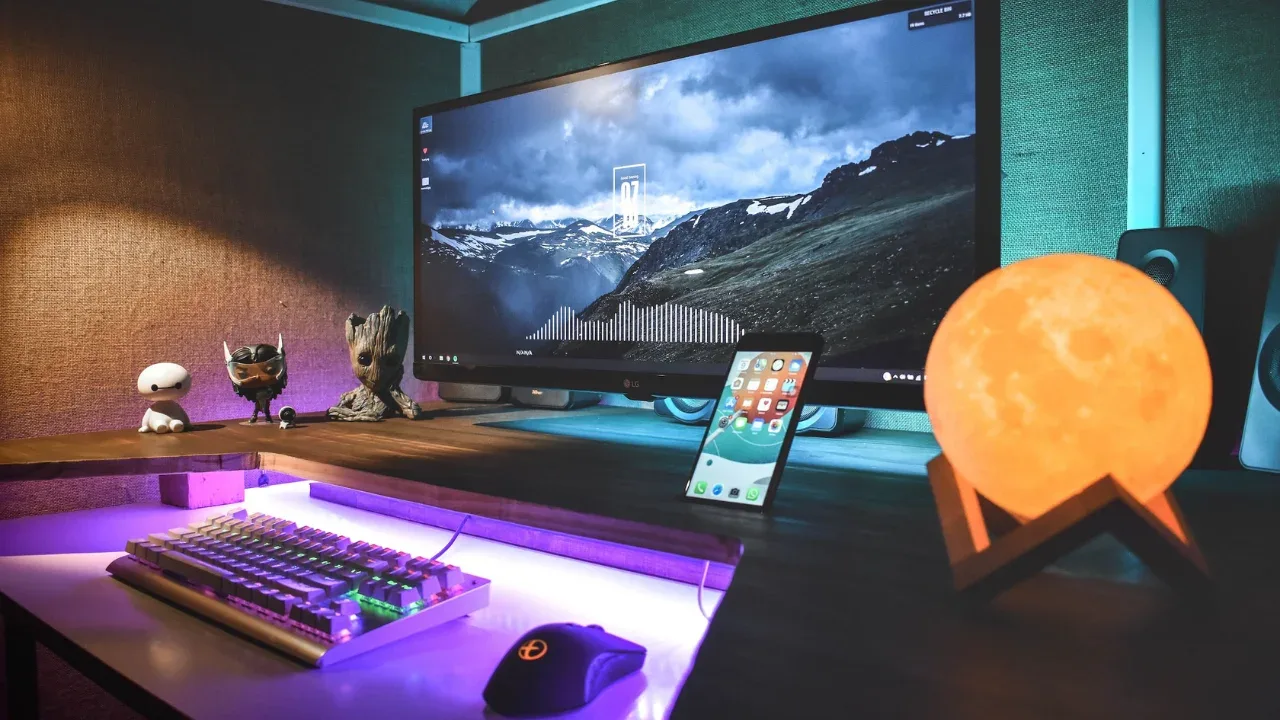
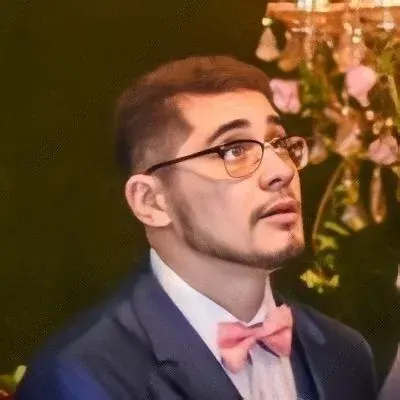
🚀 Supercharge Your Laravel Requests with Additional Values
Are you tired of the limitations when handling Laravel requests? Do you often find yourself needing to add extra values to the request array before performing a store or update operation? Fear not, because I'm here to show you an easy and elegant solution!
The Problem
Let's begin by addressing the underlying issue. In Laravel, when you call the store()
or update()
method with a Request
parameter, you might need to include additional values in the request array. This could be necessary for various reasons, such as manipulating data before updating the database or automatically populating certain fields.
🔥 A common scenario where this problem arises is when you want to associate the currently authenticated user with the resource being stored or updated. It's a super cool feature, trust me!
The Solution
To add values to the request array, you can leverage Laravel's powerful form request validation feature. Here's how you can do it:
Create a new form request class by running the following command in your terminal:
php artisan make:request MyCustomRequest
Open the newly created
MyCustomRequest
class located in theapp/Http/Requests
directory. Inside this class, you will find arules()
method. Within this method, you can define any additional data you want to include in your request array.
public function rules()
{
return [
'name' => 'required|string',
'email' => 'required|email',
// Add any other validation rules you need
'additional_value1' => 'nullable', // Add any additional values you want
'additional_value2' => 'nullable',
];
}
Now, let's update our controller method to use this custom request class instead of the default
Request
. Open your controller and import your newMyCustomRequest
class at the top:
use App\Http\Requests\MyCustomRequest;
Update your method signature to include the
MyCustomRequest
as the parameter:
public function store(MyCustomRequest $request)
{
// Your additional logic or checking goes here
// Access the additional values using $request->input('additional_value1')
User::create($request->all());
}
And that's it! 🎉 You've successfully added additional values to the request array.
Why This Solution Rocks
By using Laravel's form request validation, you not only add values to the request array, but you also benefit from enhanced validation capabilities. You can easily validate the incoming data, enforce specific rules, and prevent invalid requests from reaching your controller.
💡 Pro Tip: If you don't need to validate the extra values you're adding, you can simply set the rules to 'nullable'
or 'required'
as per your requirements.
Join the Laravel Revolution!
Now that you've learned this neat trick for adding values to the request array in Laravel, sky's the limit! Get creative and explore the endless possibilities that Laravel has to offer. 💪
If you have any questions or other Laravel-related topics you'd like me to dive into, let me know in the comments below. I'd love to hear from you! 😊
💌 Don't forget to share this post with your fellow Laravel enthusiasts. Together, we can revolutionize web development!
Keep coding, keep exploring, and keep slaying the tech world! ✨💻
Happy Larave(ling) 🌟