How to validate an email address in PHP
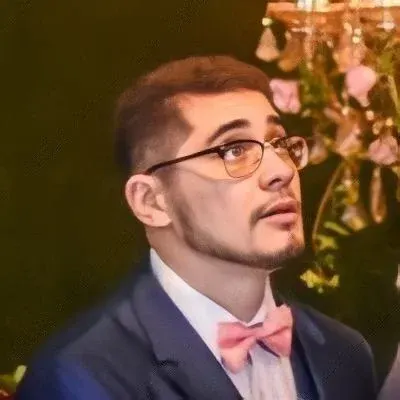
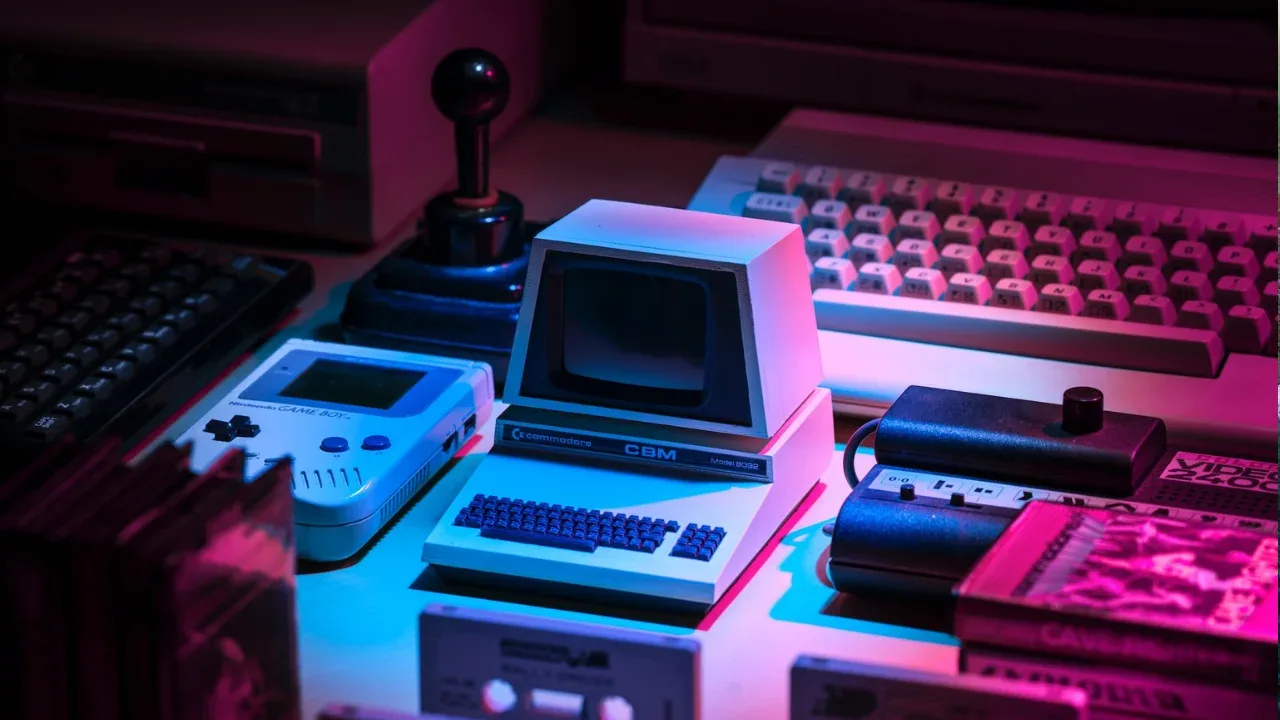
📧 How to validate an email address in PHP 📧
Have you ever wondered how to validate an email address in PHP? Whether you're building a registration form or implementing an email verification feature, it's important to ensure that the email address provided by the user is valid. In this blog post, we will address the common issues surrounding email validation in PHP and provide you with an easy solution.
The Function to Validate an Email Address
Let's start by taking a look at the function provided:
function validateEMAIL($EMAIL) {
$v = "/[a-zA-Z0-9_-.+]+@[a-zA-Z0-9-]+.[a-zA-Z]+/";
return (bool)preg_match($v, $EMAIL);
}
Now, this function uses regular expressions to match the email address pattern. While it may seem sufficient at first glance, it may not cover all possible valid email addresses. In fact, it has a few limitations that can lead to false positives or negatives.
1. Limited TLD Support
The regex used in the function assumes that the top-level domain (TLD) will always consist of only letters. This means that emails with TLDs like ".info" or ".xyz" will be considered invalid. Considering the variety of TLDs available today, it's essential to support all valid TLDs.
2. Special Characters
The function doesn't account for email addresses with special characters like hyphens, underscores, or plus symbols. These characters are valid in both the local-part and domain of an email address.
3. Case Sensitivity
The regex used in the function also assumes that the domain and TLD will only consist of uppercase or lowercase letters. However, email addresses are case-insensitive, and the domain and TLD can contain a mix of uppercase and lowercase letters.
A More Comprehensive Solution
To overcome these limitations and ensure accurate email validation, it's recommended to use a more comprehensive solution like PHP's built-in filter_var
function with the FILTER_VALIDATE_EMAIL
filter.
Here's an example of how you can utilize filter_var
to validate an email address:
function validateEmail($email) {
return filter_var($email, FILTER_VALIDATE_EMAIL) !== false;
}
With this approach, you can confidently validate all email addresses, regardless of their TLDs or the presence of special characters.
🎉 Your Turn! Share Your Thoughts! 🎉
Now that you understand the limitations of the original function and have a better solution at hand, what are your thoughts? Have you encountered any email validation issues in your PHP projects? Share your experiences and suggestions in the comments below!
👉 Don't forget to subscribe to our newsletter to receive more PHP tips and tricks straight to your inbox.
Conclusion
Validating email addresses in PHP is an essential task to ensure data integrity and user experience. While the initial function provided may seem sufficient, it has limitations that can lead to inaccurate validation results. By utilizing PHP's filter_var
function with the FILTER_VALIDATE_EMAIL
filter, you can create a more comprehensive and reliable solution.
Remember, an accurate email validation process helps you prevent spam registrations, ensure communication reaches the intended recipients, and deliver a seamless user experience. Keep coding and make your email validation rock-solid! 💪✉️
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
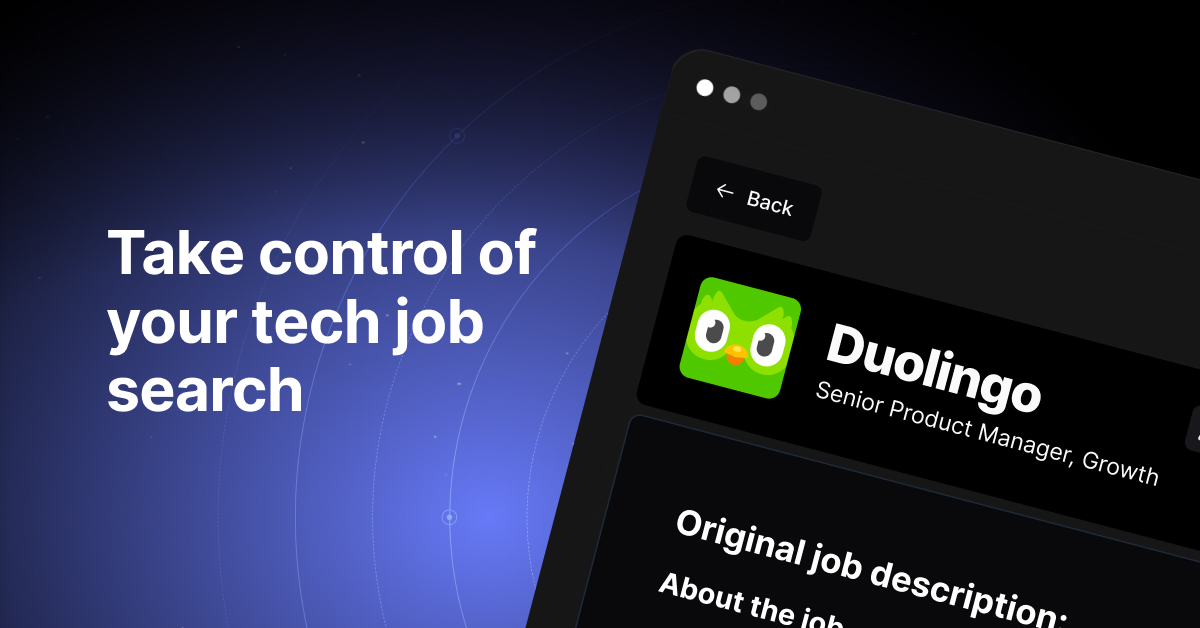