How to use multiple databases in Laravel
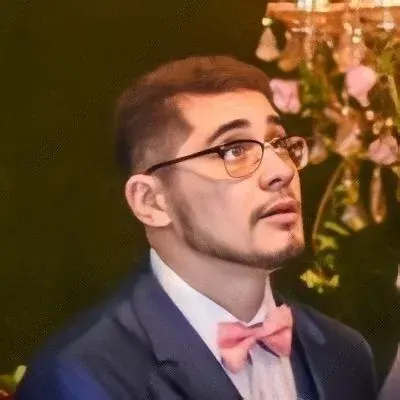
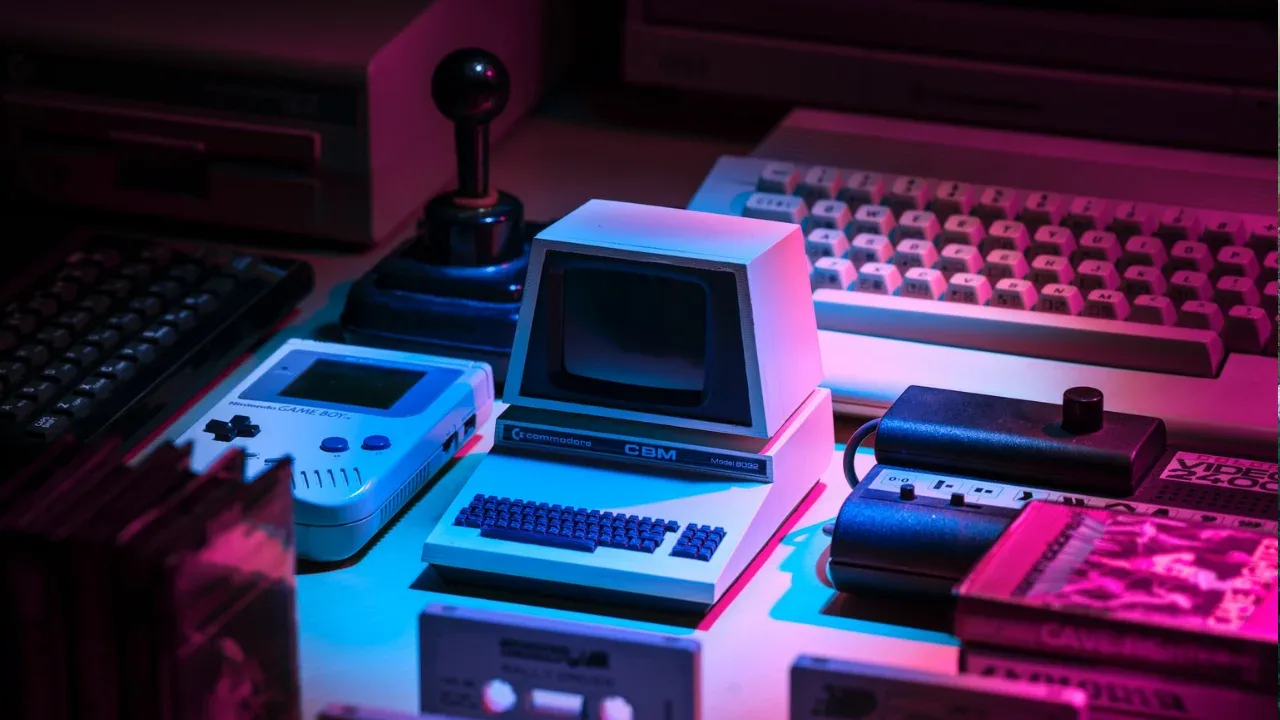
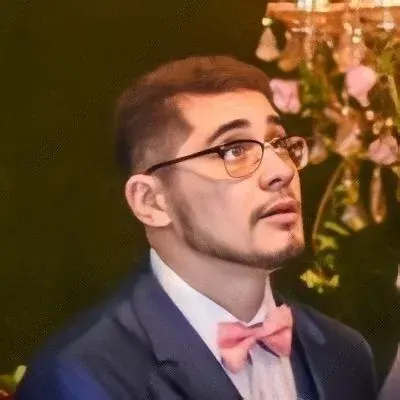
How to Use Multiple Databases in Laravel 💾
Are you facing some serious 💥 database challenges in your Laravel application? Do you need to combine multiple databases of different types? Well, worry not! Laravel has got your back! 🙌 In this blog post, we will explore the different options and provide easy solutions to help you successfully manage multiple databases in your Laravel application. Let's dive in! 🏊♂️
The Challenge: Dealing with Multiple Databases of Different Types
So, you want to have a system that effectively combines multiple databases. Maybe you're currently using MySQL, but you want to be prepared for the future when the admin can generate reports from heterogeneous database systems. 🤔
Now, the burning question is: Does Laravel provide any Facade to deal with such situations? Or is there another framework that offers more suitable capabilities? 🤷♂️
The Laravel Solution: Database Connections to the Rescue! 🚀
Laravel, being the flexible and powerful framework that it is, provides an elegant solution for working with multiple databases. Meet Database Connections! 💪
With Laravel, you can easily manage multiple databases by defining separate database connections that correspond to each database you need to access. Let's see how it's done step-by-step:
Step 1: Configuration Setup 🛠️
To begin, open your .env
file and add the necessary database connection details. For example, let's say we have two databases: mysql
and sqlite
. We need to define their respective connection details like so:
DB_CONNECTION=mysql
DB_HOST=your_mysql_host
DB_PORT=3306
DB_DATABASE=mysql_database_name
DB_USERNAME=mysql_username
DB_PASSWORD=mysql_password
DB_CONNECTION_SECONDARY=sqlite
DB_DATABASE_SECONDARY=/path/to/sqlite/database.sqlite
Step 2: Create Database Connection Configuration 📝
Now that we have our database connections defined in the .env
file, we need to create the corresponding configuration in the Laravel framework.
Open your config/database.php
file and add a new array element to the 'connections'
array for each additional database connection you need. Here's an example:
'connections' => [
'mysql' => [
'driver' => 'mysql',
'host' => env('DB_HOST'),
'port' => env('DB_PORT'),
'database' => env('DB_DATABASE'),
'username' => env('DB_USERNAME'),
'password' => env('DB_PASSWORD'),
'charset' => 'utf8mb4',
'collation' => 'utf8mb4_unicode_ci',
'prefix' => '',
'strict' => true,
'engine' => null,
],
'secondary' => [
'driver' => 'sqlite',
'database' => env('DB_DATABASE_SECONDARY'),
'prefix' => '',
],
],
Step 3: Accessing Multiple Databases in Code 💻
Now that we have our database connections configured, we can easily switch between them while executing queries. Here's an example of how you can use the DB
facade to specify the desired database connection:
$users = DB::connection('secondary')->table('users')->get();
And just like that, you can access any table in any configured database by specifying the appropriate connection using DB::connection('your_connection_name')
. It's that easy! 😎
Your Turn! 💪
Now that you understand how to use multiple databases in Laravel, it's time to give it a try! Implementing this feature in your application can provide immense flexibility and future-proof your system for any reporting needs. 📈
If you encounter any difficulties or have any questions, feel free to drop a comment below. Our community is always ready to help! 🤝
Happy coding and multiplexing databases with Laravel! 🚀✨