How to Use AJAX in a WordPress Shortcode?
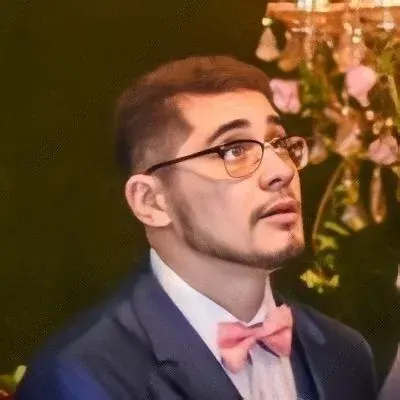
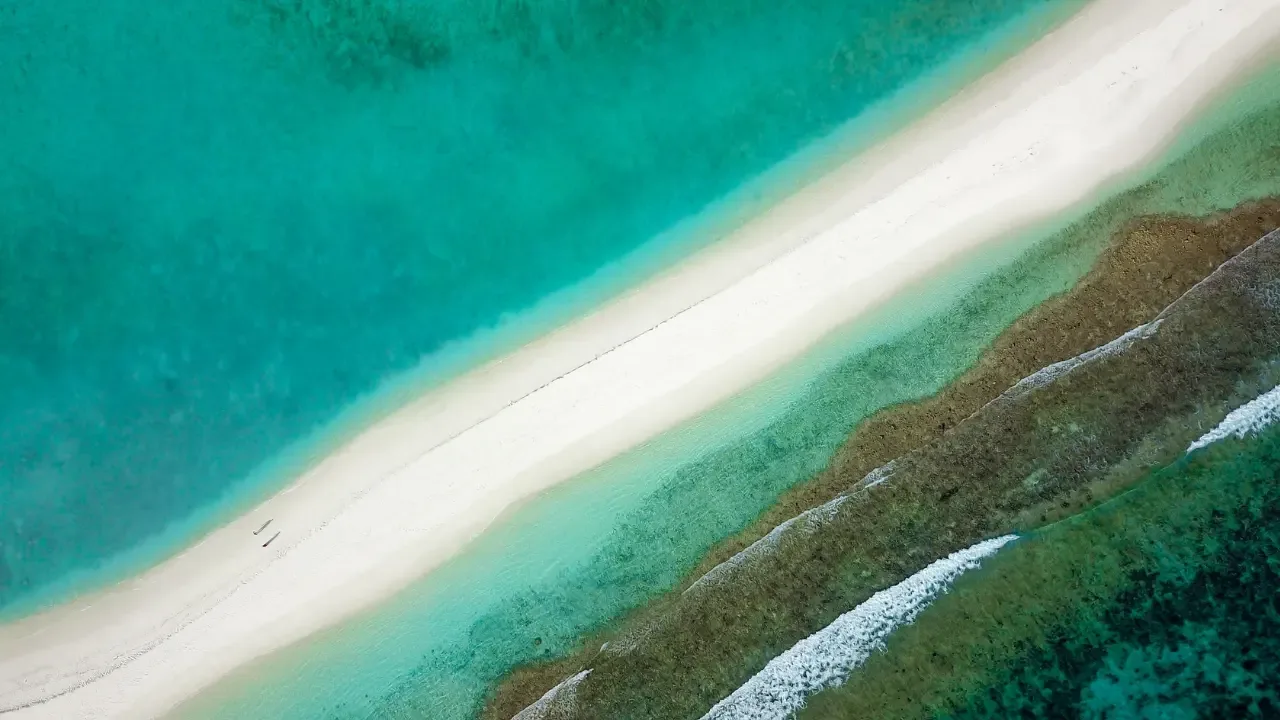
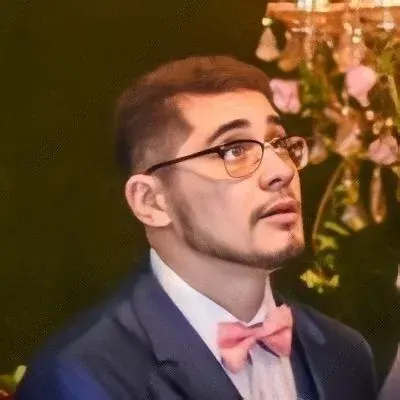
How to Use AJAX in a WordPress Shortcode? π»π
So, you want to update the content on your WordPress page using AJAX? Well, you've come to the right place! In this blog post, we will walk you through how to use AJAX in a WordPress shortcode, addressing common issues and providing easy solutions to help you achieve your goal. Let's dive in! π
The Problem π«
One of our readers had a code to display a random quote on their WordPress site. They had all the necessary PHP, HTML, and jQuery code in place, but for some reason, the AJAX update wasn't working. When they clicked the "New Quote" button, nothing happened. π¬
The Solution π‘
To fix this issue, we need to make a few adjustments to the code in the following files:
1. The PHP File π
Let's start by looking at the PHP file where the AJAX request is being handled. In this case, the file is located at /wp-content/themes/%your_theme%/js/ajax-load-quote.php
. Make sure the file path matches your theme directory.
<?php
$array = file($_POST['file_path']); // file path in $_POST, as from the js
$r = rand(0, count($array) - 1);
return '<p>' . $array[$r] . '</p>';
?>
Here, we are fetching the file path sent via the AJAX request and using it to retrieve a random quote from the file. The quote is then returned as HTML wrapped in <p>
tags.
2. The HTML Structure ποΈ
Next, let's take a look at the HTML structure that contains the quote content and the "New Quote" button. You can adjust this structure according to your preference, but for now, let's go with the example provided.
<div id="randomquotes">
<p>I would rather have my ignorance than another manβs knowledge,
because I have so much more of it.<br/>
-- Mark Twain, American author & Playwright</p>
</div>
<a id="newquote" class="button" href="#" title="Gimme a new one!">New Quote</a>
In this example, we have a div
element with an id of "randomquotes" that contains the quote text. We also have an a
element with an id of "newquote" that represents the "New Quote" button.
3. The jQuery Code π₯οΈ
Now, let's move on to the jQuery code that handles the AJAX request and updates the quote content.
function ajaxQuote() {
var theQuote = jQuery.ajax({
type: 'POST',
url: ajaxParams.themeURI + 'js/ajax-load-quote.php',
data: { file_path: ajaxParams.filePath },
beforeSend: function() {
ajaxLoadingScreen(true, '#randomquotes');
},
success: function(data) {
jQuery('#randomquotes').find('p').remove();
jQuery('#randomquotes').prepend(data);
},
complete: function() {
ajaxLoadingScreen(false, '#randomquotes');
}
});
return theQuote;
}
/* Loading screen to be displayed during the process, optional */
function ajaxLoadingScreen(switchOn, element) {
// ...
}
/* Triggering the above via the click event */
jQuery('#newquote').click(function() {
var theQuote = ajaxQuote();
return false;
});
In this code, we define the ajaxQuote
function that sends a POST request to the ajax-load-quote.php
file. We pass the file path as a POST variable using the data
parameter. In the success callback, we update the quote content by removing the existing <p>
element and prepending the new quote data. We also use the ajaxLoadingScreen
function to display a loading screen while the AJAX request is being processed (this is optional).
4. The functions.php File π
Finally, we need to add the necessary code to the functions.php
file to handle the shortcode and enqueue the jQuery script.
function random_quote($atts) {
extract(shortcode_atts(array(
'path' => get_template_directory_uri() . '/quotes.txt'
), $atts));
$array = file($path);
$r = rand(0, count($array) - 1);
$output = '<div id="randomquotes">' .
'<p>' . $array[$r] . '</p>' .
'</div>' .
'<a id="newquote" class="button" href="#" title="Gimme a new one!">New Quote</a>';
wp_enqueue_script('ajax-quote');
wp_localize_script(
'ajax-quote',
'ajaxParams',
array(
'filePath' => $path,
'themeURI' => get_template_directory_uri() . '/'
)
);
return $output;
}
add_shortcode('randomquotes', 'random_quote');
function wpse72974_load_scripts() {
if (!is_admin()) {
wp_register_script(
'ajax-quote',
get_template_directory_uri() . '/js/ajax-load-quote.js',
array('jquery'),
'1.0',
true
);
}
}
add_action('init', 'wpse72974_load_scripts');
Here, we define the random_quote
function that handles the shortcode. It extracts the value of the path
shortcode attribute and uses it to fetch a random quote. We also enqueue and localize the ajax-quote
script, passing the file path and theme URI as variables.
Time for Action! β°
Now that we have the code in place, it's time to test it out! Simply add the [randomquotes]
shortcode to any WordPress page or post, and you should see the random quote and the "New Quote" button. Clicking the button will trigger the AJAX request and update the quote content without reloading the page. How cool is that? π
Conclusion π
Using AJAX in a WordPress shortcode can be incredibly useful in updating page content dynamically. By following the steps outlined in this blog post, you'll be able to implement AJAX functionality and impress your site visitors with smooth and seamless updates. So go ahead, try it out, and let us know how it goes! π
Have any questions or encountered any issues along the way? We're here to help! Leave a comment below, and we'll get back to you. π
Happy coding! π»β¨