How to sort a Laravel query builder result by multiple columns?
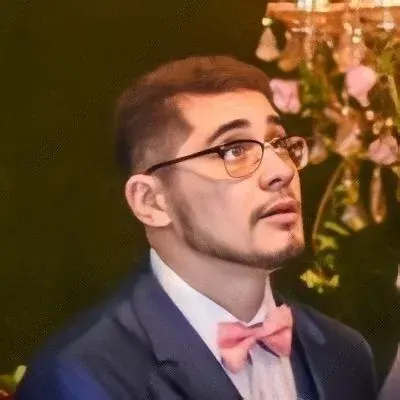
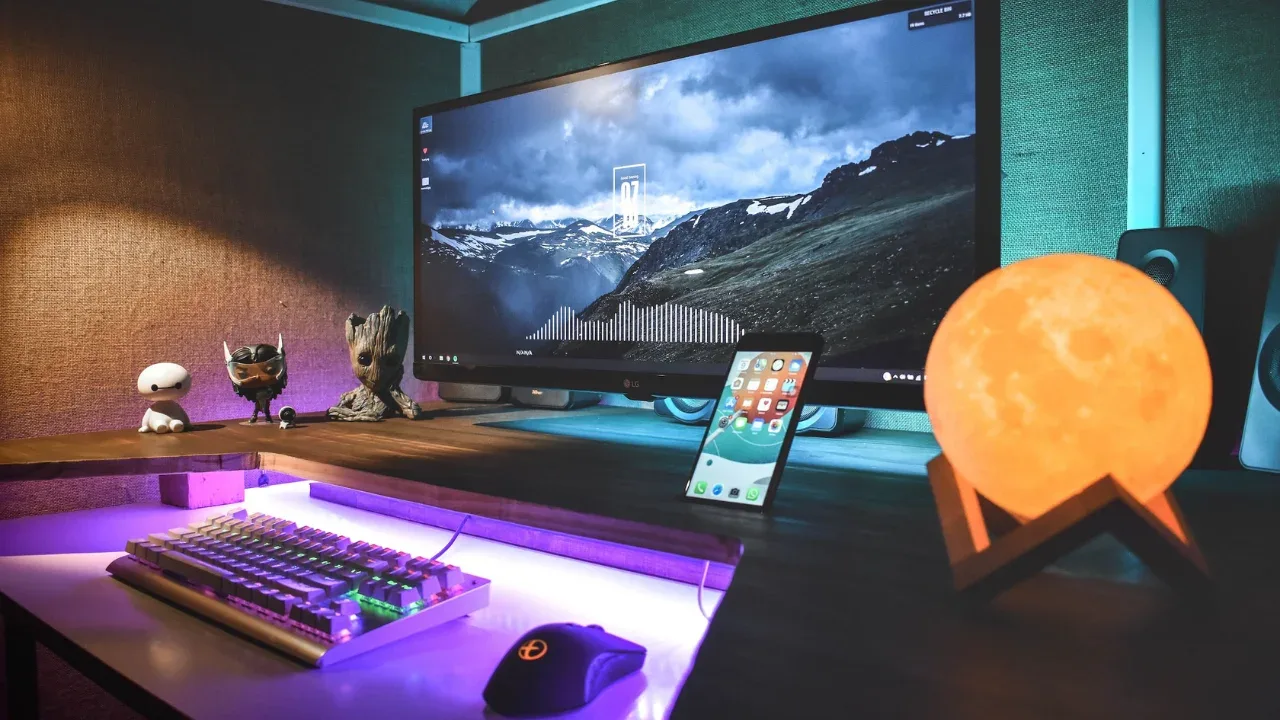
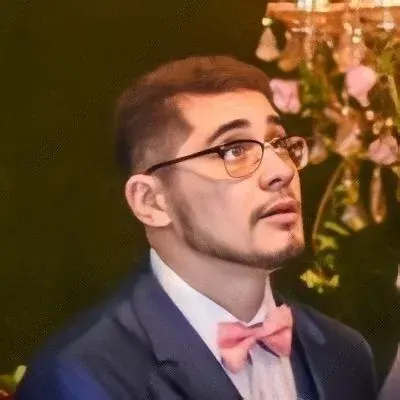
π‘Title: Sorting a Laravel Query Builder Result by Multiple Columns: A Comprehensive Guide
Intro:
Hey tech enthusiasts, ever found yourself dreading the complex process of sorting data in Laravel? Well, fear no more! In this guide, we'll walk you through a step-by-step process to sort a Laravel query builder result by multiple columns using the powerful orderBy()
method in Laravel Eloquent. Let's dive right in!
πUnderstanding the Issue
When it comes to sorting query builder results in Laravel, things can get a bit tricky, especially when you need to sort by multiple columns. The original question revolves around using the orderBy()
method in Laravel Eloquent to achieve this.
βοΈThe Solution: Sorting by Multiple Columns with orderBy()
To sort query builder results by multiple columns using the orderBy()
method, we can simply chain multiple calls to orderBy()
, each specifying the desired column and sorting order.
Here's an example:
$result = DB::table('mytable')
->orderBy('column1', 'desc')
->orderBy('column2', 'asc')
->get();
In the example above, we start by calling the orderBy()
method on the query builder and provide the name of the first column we want to sort (column1
in this case), followed by the desired sorting order (desc
for descending, or asc
for ascending). Then, we chain the second orderBy()
method call to sort by the second column (column2
), specifying the desired sorting order as well.
By chaining multiple orderBy()
methods, we can sort the query builder result by multiple columns in the desired order.
πExample Usage: Sorting a Laravel Query Builder Result by Multiple Columns
To demonstrate how to sort a Laravel query builder result by multiple columns, letβs consider an example where we have a table of users
and we want to sort the results by name
in ascending order, then by age
in descending order.
Here's the code:
$users = DB::table('users')
->orderBy('name', 'asc')
->orderBy('age', 'desc')
->get();
In the code snippet above, we start by querying the users
table, then we chain two orderBy()
method calls. The first call sorts the users' names in ascending order (asc
), while the second call sorts their ages in descending order (desc
).
Simple, right? Now you can flexibly sort query builder results by multiple columns to meet your specific needs.
πWrap Up
Sorting a Laravel query builder result by multiple columns is no longer a puzzle. By using the orderBy()
method and chaining multiple calls, you can efficiently sort query results based on your unique requirements. Remember, the order of the orderBy()
methods matters, as it defines the priority of the sorting columns.
So, what are you waiting for? Give it a shot yourself and enhance your Laravel projects' sorting capabilities!
Have you faced any issues with sorting query builder results in Laravel? How did you overcome them? Share your experiences with us in the comments below! Let's learn from each other.
πRead More
Continuously expand your Laravel knowledge and explore further topics by checking out these related articles:
πStay Connected
Stay up to date with the latest tech tutorials, guides, and insights by subscribing to our newsletter. Don't miss out on the opportunity to level up your skills and build amazing applications!
Remember, sorting query builder results in Laravel can be an art form β master it, and you'll have the power to create efficient and user-friendly applications.
Happy coding! β¨π