How to query between two dates using Laravel and Eloquent?
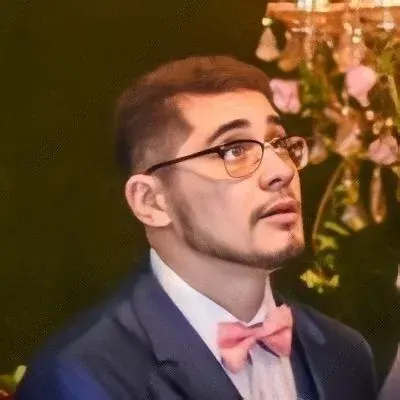
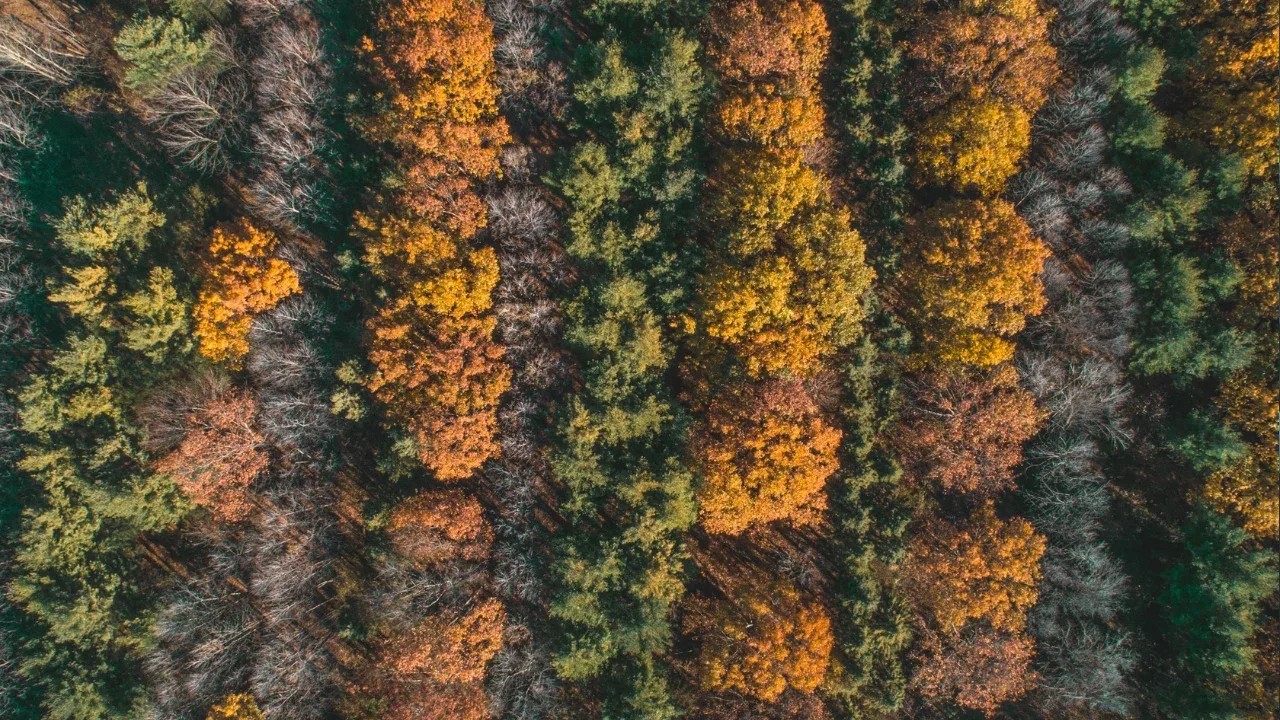
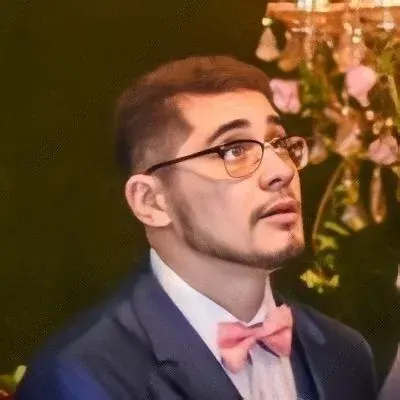
How to Query Between Two Dates using Laravel and Eloquent?
Are you trying to create a report page that shows reports from a specific date to another specific date? 📅 Well, look no further because we've got you covered! In this blog post, we'll walk you through the process of querying between two dates using Laravel and Eloquent, providing easy-to-follow solutions to common issues you may encounter along the way. Let's get started! 💪
The Context:
Let's begin by addressing the code snippet you provided:
$now = date('Y-m-d');
$reservations = Reservation::where('reservation_from', $now)->get();
In plain SQL, this code performs the following query:
select * from table where reservation_from = $now
However, you mentioned that you want to convert this query into an Eloquent query that checks for a range of dates using the BETWEEN
clause. Don't worry, we've got a solution for you! 🙌
The Solution:
To query between two dates using Laravel and Eloquent, you can utilize the whereBetween
method. Let's take a look at how you can modify your code to achieve this:
$from = '2022-01-01'; // Specify your desired "from" date
$to = '2022-01-31'; // Specify your desired "to" date
$reservations = Reservation::whereBetween('reservation_from', [$from, $to])->get();
In the code above, we're using the whereBetween
method to specify the column reservation_from
and the range of dates we're interested in ($from
and $to
). This query is equivalent to the following plain SQL query:
SELECT * FROM table WHERE reservation_from BETWEEN '$from' AND '$to'
Now you have an eloquent query that meets your requirements! 🎉
Enhancing the Query:
If you want to further refine your query by including the end date in the results, you can use the whereDate
method in conjunction with the whereBetween
method:
$from = '2022-01-01'; // Specify your desired "from" date
$to = '2022-01-31'; // Specify your desired "to" date
$reservations = Reservation::whereDate('reservation_from', '>=', $from)
->whereDate('reservation_from', '<=', $to)
->get();
The code above will include records that match the start and end dates within the given range. This additional refinement can be useful if you want to capture the entire range inclusively.
Wrap-up:
Querying between two dates using Laravel and Eloquent is now within your reach! 🚀 With the solutions provided in this blog post, you can easily modify your code to perform the desired query. Remember to replace the static dates ($from
and $to
) with your own variables or user inputs to make it dynamic.
If you have any questions or faced any issues while implementing these solutions, feel free to leave a comment below. We're here to help! 👩💻👨💻
Now it's your turn! Go ahead and give it a try. Happy coding! 💻
Do you find this blog post helpful? Share your thoughts and experiences in the comments section below! 📝✨