How to push both value and key into PHP array
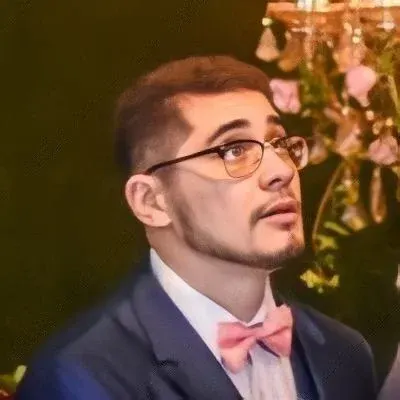
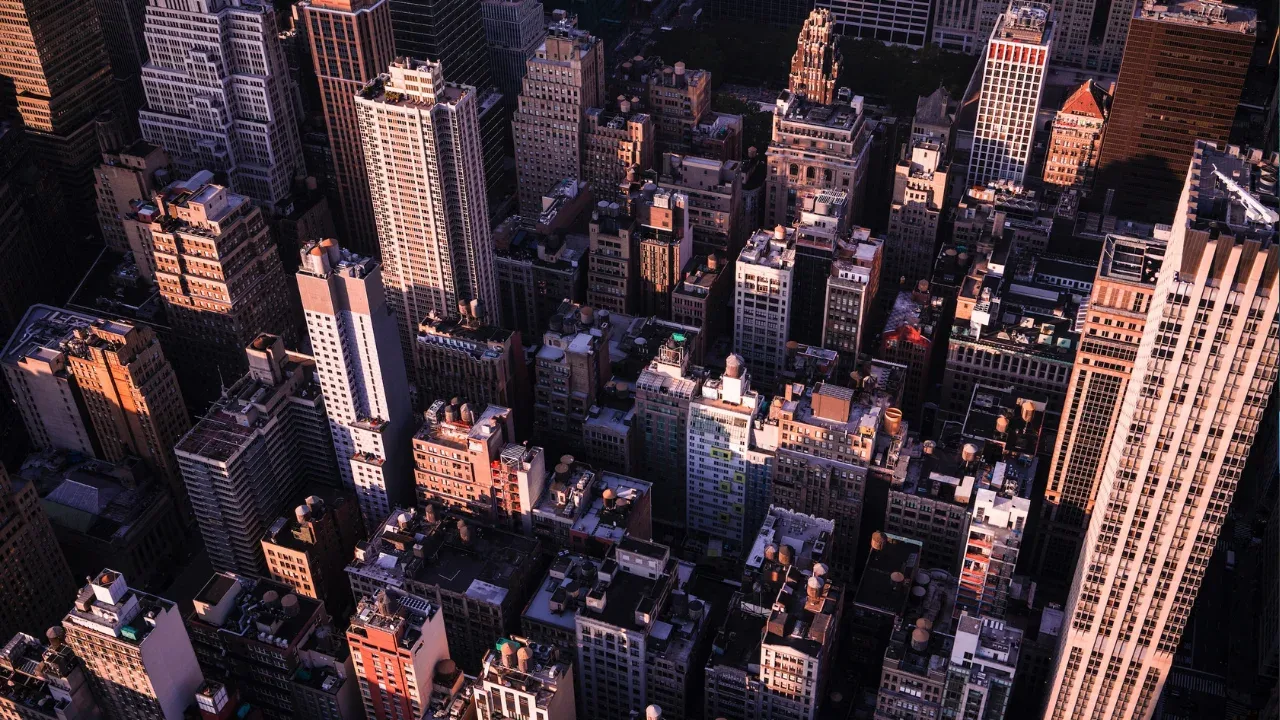
How to Push Both Value and Key into PHP Array 🚀
Are you struggling to push both the value and key into a PHP array? 😫 Don't worry, I've got you covered! In this guide, I'll walk you through a common issue faced by many developers and provide easy solutions to achieve the desired result. By the end, you'll be able to effortlessly push both the value and key into your PHP array. Let's get started, shall we? 💪
The Problem
Let's first understand the context around this question with a piece of code:
$GET = array();
$key = 'one=1';
$rule = explode('=', $key);
/* array_push($GET, $rule[0] => $rule[1]); */
The code snippet above shows an attempt to push both the key and value into the $GET
array. However, using the array_push
function in this way won't work. 😞
The Solution
To achieve the desired output ($GET[one => 1, two => 2, ...]
), we need to use a different approach. Fortunately, PHP provides us with a handy function called array_combine
that does exactly what we need. 🎉
Here's how you can solve the problem using array_combine
:
$GET = array();
$key = 'one=1';
$rule = explode('=', $key);
$GET = array_combine([$rule[0]], [$rule[1]]);
In the code above, we first initialize the $GET
array. Next, we explode the $key
string using the =
delimiter to separate the key and value. Finally, we use array_combine
to combine the key and value arrays into a single associative array.
Explanation
Let's break down the solution step by step:
Initialize the
$GET
array:
$GET = array();
Explode the
$key
string into an array:
$key = 'one=1';
$rule = explode('=', $key);
After this step, the $rule
array will contain the key and value respectively, i.e., $rule[0] => 'one'
and $rule[1] => 1
.
Use
array_combine
to combine the key and value arrays:
$GET = array_combine([$rule[0]], [$rule[1]]);
By passing an array containing the key ($rule[0]
) and an array containing the value ($rule[1]
) to array_combine
, we create the final associative array where the key ($rule[0]
) points to the value ($rule[1]
).
And that's it! You have successfully pushed both the value and key into the PHP array using array_combine
. 🎉
Better Approach: Associative Array Initialization
Alternatively, you can also initialize the $GET
array with the key and value directly, eliminating the need for array_combine
. Here's how:
$GET = array(
$rule[0] => $rule[1]
);
By assigning the key and value directly in the array initialization, you achieve the same result without using array_combine
.
Conclusion
Pushing both the value and key into a PHP array may seem daunting at first, but with the help of array_combine
or direct initialization, you can tackle it effortlessly. 🙌
Now that you have learned the solutions, it's time to apply them in your own code. Give it a try and see the magic happen! 💫
If you found this guide helpful or have any questions, feel free to leave a comment below. Happy coding! 😄
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
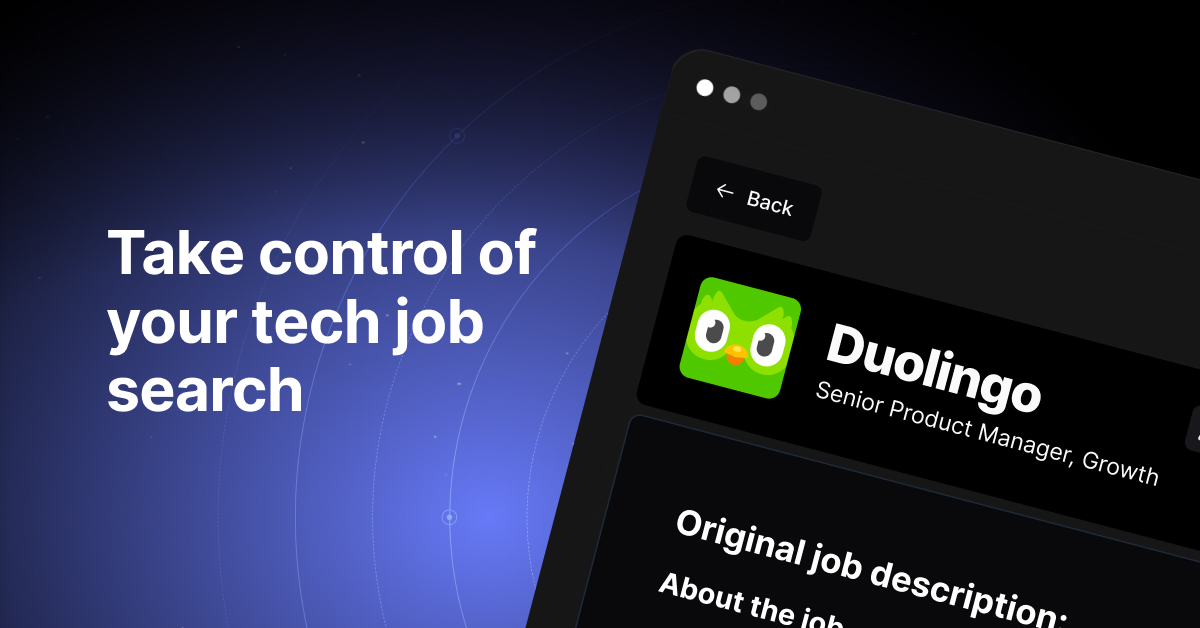