How to Merge Two Eloquent Collections?
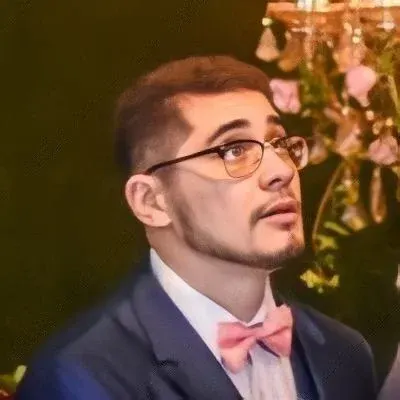
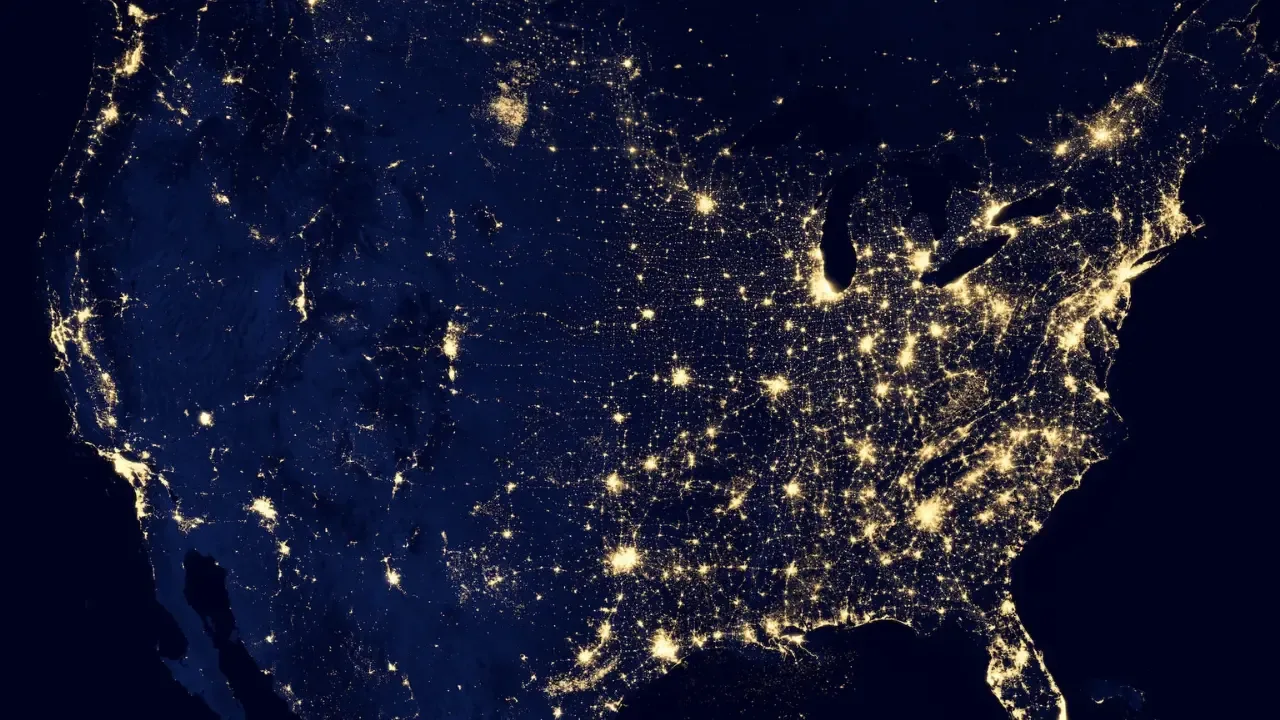
How to Merge Two Eloquent Collections? 😕🔀
So you want to fetch all questions from tags of a given question? 🤔😮 Well, fear not! This blog post is here to help you out. 🎉✨
Let's start by examining the problem at hand. You have a "questions" table and a "tags" table, with a many-to-many relationship defined in Eloquent as belongsToMany. You want to fetch all questions for a given set of tags. Seems straightforward, right? 😅
Our friend in the snippet you shared tried using the merge() method to combine the question collections obtained from each tag. But alas, it didn't work out as expected. 😞
The good news is, we have some easy solutions at our disposal! 🙌✨
❗️Solution 1: Use Eloquent's whereHas Method
Eloquent provides a handy method called whereHas that allows us to query based on the existence of a relationship.🔍🌟
Here's how you can achieve the desired result using whereHas:
$tagNames = ["Travel", "Trains", "Culture"];
$questions = Question::whereHas('tags', function($query) use ($tagNames) {
$query->whereIn('name', $tagNames);
})->get();
// Do something with the fetched questions...
Here, we're using whereIn to specify that we want tags with names that match the ones in our $tagNames array. The result is a collection of questions that have at least one of the specified tags. Boom! 💥
❗️Solution 2: Lazy Loading Approach
If you don't need to load all the questions at once, you can use lazy loading to load the questions dynamically. 🐢💨
Consider the following code:
class Question extends Model
{
// ...
public function getRelatedQuestionsAttribute()
{
return $this->tags->flatMap(function ($tag) {
return $tag->questions;
});
}
}
By adding a getter for the relatedQuestions attribute, you can access the related questions using the dot notation. For example:
$relatedQuestions = $question->relatedQuestions;
This lazy loading approach allows you to fetch the related questions on-demand, only loading them when accessed. 🚀
🌟Engage with the Community!
Now that you've learned how to merge two Eloquent collections, it's time to put your newfound knowledge into action. Share your experiences, ask questions, and engage with other developers facing similar challenges. Together, we can learn and grow! 💪💻
What other Eloquent challenges have you encountered? Share your stories, code snippets, and tips in the comments below! Let's level up our Eloquent skills together. 🎓🔥
Happy merging! ✨🔄
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
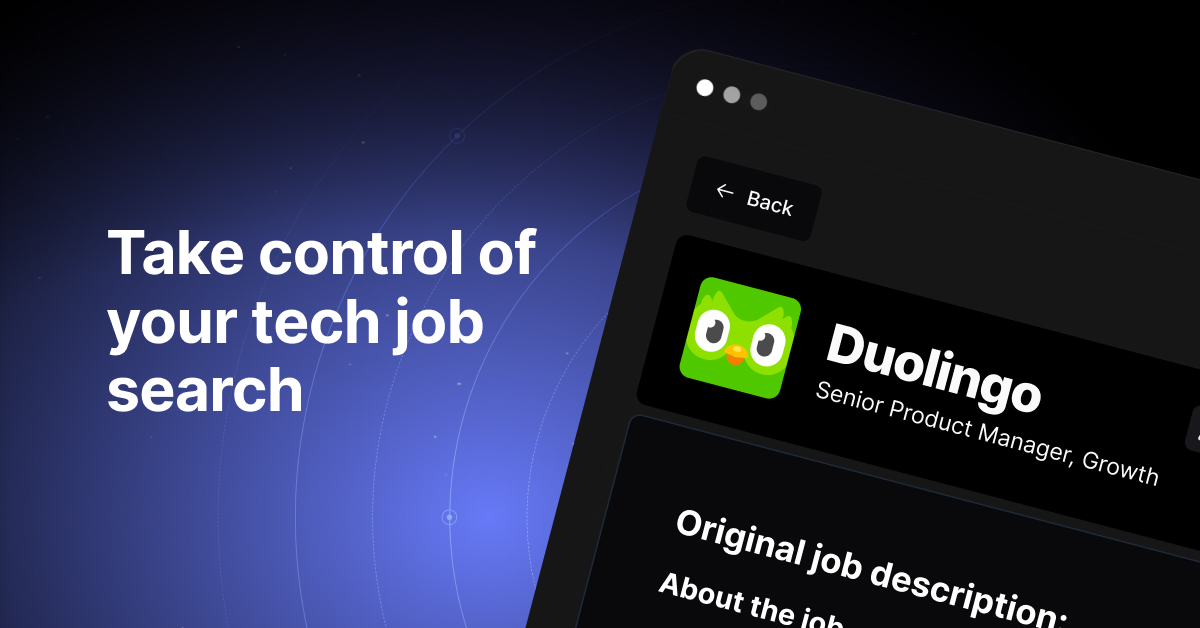