How to Make Laravel Eloquent "IN" Query?
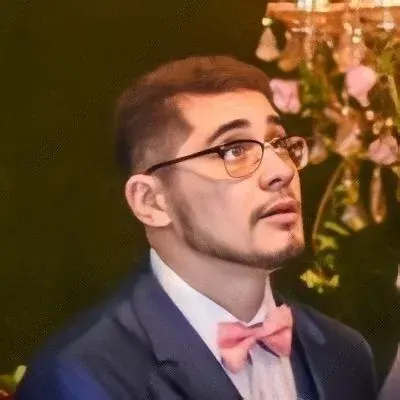
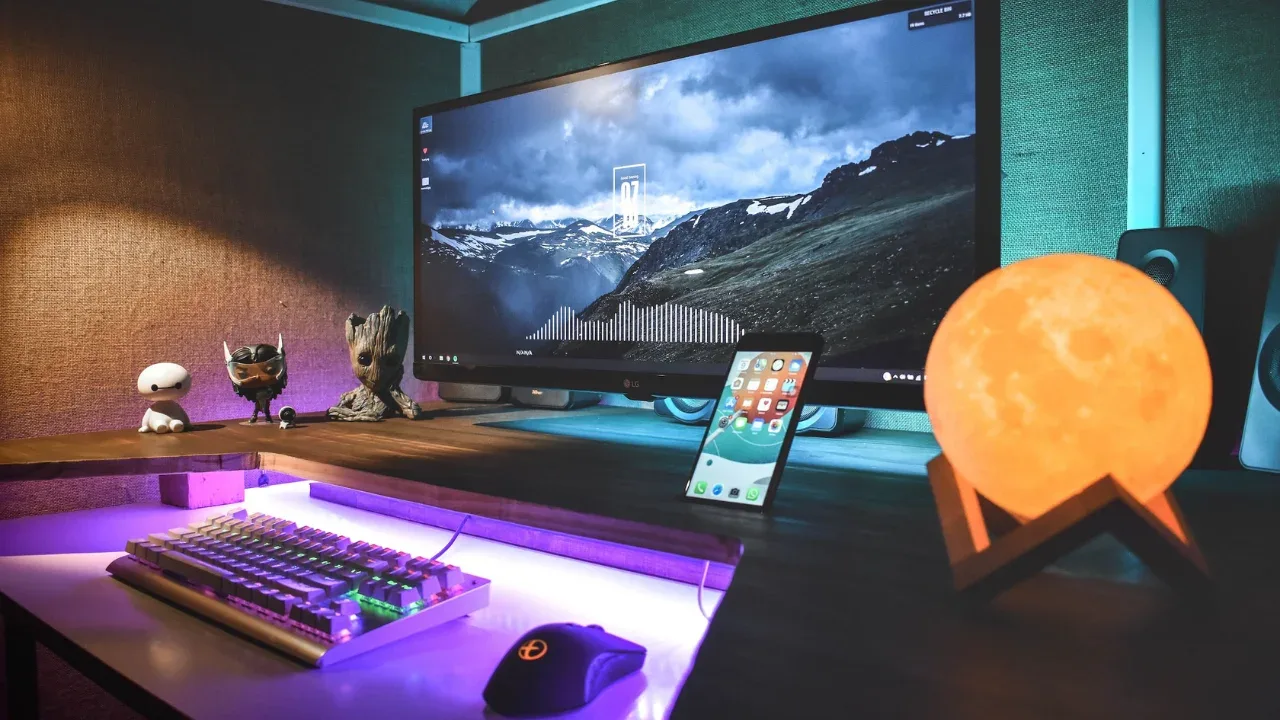
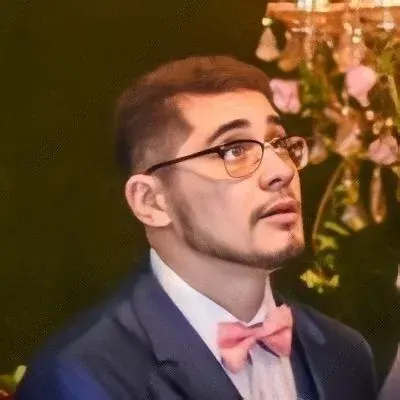
How to Make Laravel Eloquent "IN" Query? 🚀
Are you facing trouble while trying to make an "IN" query using Laravel Eloquent? You're not alone! Many developers struggle with this, but fear not, we've got your back! In this post, we will walk you through the steps to make this query work seamlessly in Laravel Eloquent. 💪
The Problem 💥
Many developers, just like you, struggle to find the correct syntax to perform an "IN" query using Laravel Eloquent. Trying to imitate a raw MySQL query, like the one shown below, often leads to frustration:
SELECT * FROM exampleTbl WHERE id IN (1, 2, 3, 4)
Attempting something like this using Laravel's Eloquent ORM doesn't yield the expected results:
DB::where("id IN(23, 25)")->get();
The query above is incorrect, and it won't work as expected. But don't worry! We'll show you the proper way to solve this.
The Solution ✅
To execute an "IN" query properly, we need to leverage Laravel Eloquent's syntax. The correct way to execute this query is by utilizing the whereIn
method and passing an array of values.
$data = DB::table('exampleTbl')->whereIn('id', [1, 2, 3, 4])->get();
By using the whereIn
method, we can pass the column name as the first argument ('id' in this case). We then pass the array of values we want to check against. This will ensure that our query returns the desired results.
Explanation and Examples 📚
Let's break down the solution further with some explanation and examples for better understanding. 😉
The whereIn
method in Laravel Eloquent acts as a filter and matches values from the specified column against an array of values. If a match is found, the row is included in the result set.
Here's an example using the whereIn
method in a practical scenario:
$desiredIds = [1, 2, 3, 4];
$data = DB::table('exampleTbl')->whereIn('id', $desiredIds)->get();
In this example, $desiredIds
is an array containing the values we want to check for in the 'id' column of the 'exampleTbl' table. The whereIn
method allows us to make multiple comparisons using a single query.
Now, the $data
variable will hold all the rows from the 'exampleTbl' table where the 'id' column contains values that match the values from the $desiredIds
array.
Call to Action 📢
That's it! You now know how to effectively use the "IN" query with Laravel Eloquent. ✨
No more frustration or confusion when creating such queries. Upgrade your Laravel skills and improve your coding productivity by utilizing the whereIn
method.
If you found this guide helpful, feel free to share it with your fellow developers who might be struggling with the same issue. Happy coding! 🎉