How to insert multiple rows from a single query using eloquent/fluent
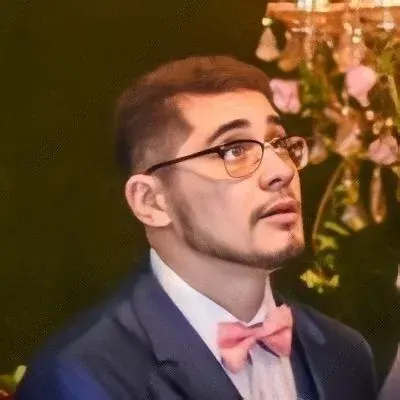
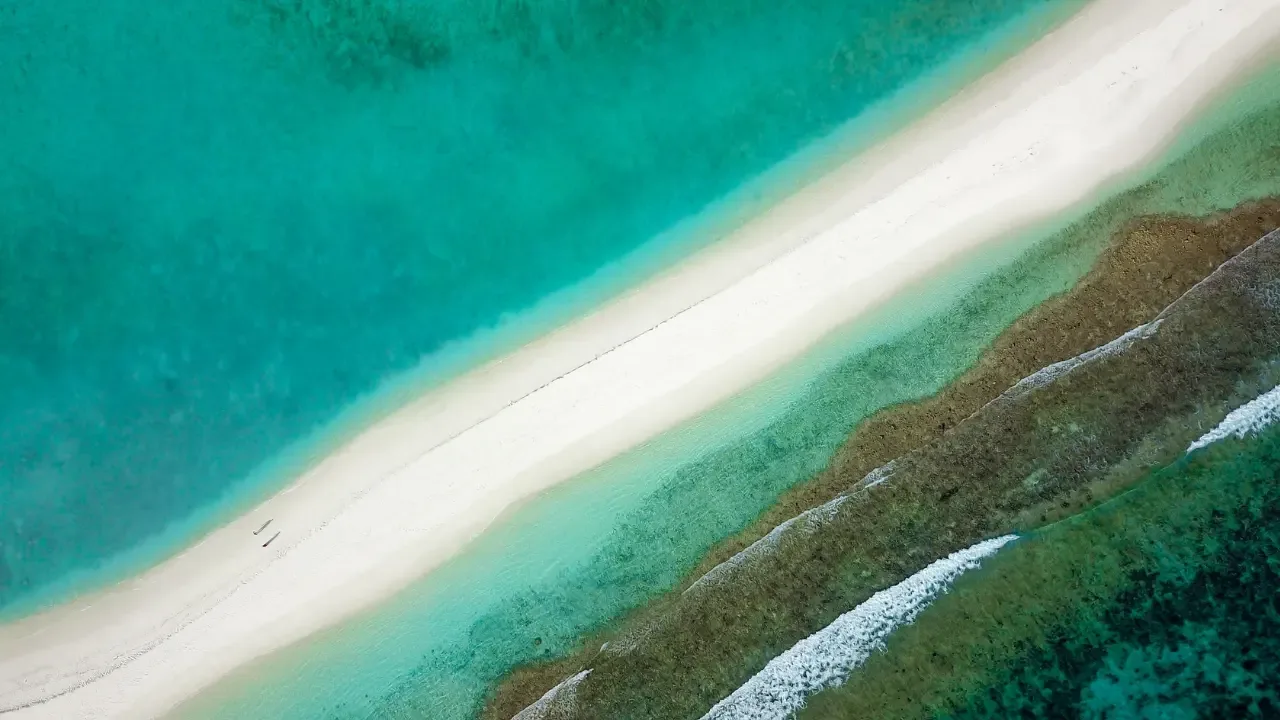
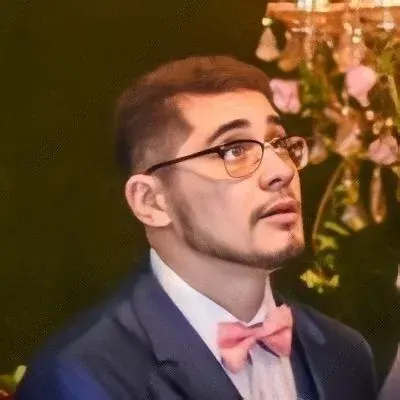
How to Insert Multiple Rows from a Single Query Using Eloquent/Fluent
Are you struggling with inserting multiple rows from a single query using Eloquent/Fluent? 🤔 Don't worry, you're not alone! Many developers find this task challenging, but fear no more. In this blog post, we'll walk you through the common issues and provide easy solutions to help you achieve your goal effortlessly. Let's get started! 🚀
The Problem
Let's say you have the following query:
$query = UserSubject::where('user_id', Auth::id())->select('subject_id')->get();
This query returns a result like this:
[{"user_id":8,"subject_id":9},{"user_id":8,"subject_id":2}]
And you want to copy this result into another table, so it looks like this:
ID|user_id|subject_id
1 |8 |9
2 |8 |2
The challenge here is that the $query
can have any number of rows, and you're unsure how to iterate through an unknown number of rows. 😓
The Solution
To solve this problem, we can use the insert()
method provided by Eloquent/Fluent. This method allows us to insert multiple rows from a single query.
Here's how you can do it:
Get the result from the
$query
by calling thetoArray()
method:$result = $query->toArray();
Assign the
user_id
value to each row in the result. We'll use themap()
method to iterate through each row and update the value:$result = collect($result)->map(function ($row) { $row['user_id'] = Auth::id(); return $row; });
Use the
insert()
method to insert the transformed result into the new table, assuming the new table is namednew_table
:DB::table('new_table')->insert($result->toArray());
That's it! 🎉 You have successfully inserted multiple rows from a single query using Eloquent/Fluent.
Conclusion
Inserting multiple rows from a single query can be a stumbling block, but with this handy solution using Eloquent/Fluent, it's a piece of cake! 🍰 Give it a try and let us know how it worked for you.
If you have any questions or alternative approaches, feel free to share them in the comments below. We'd love to hear from you! 💬