How to get the file extension in PHP?
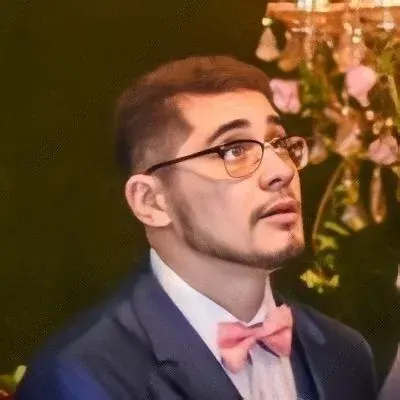
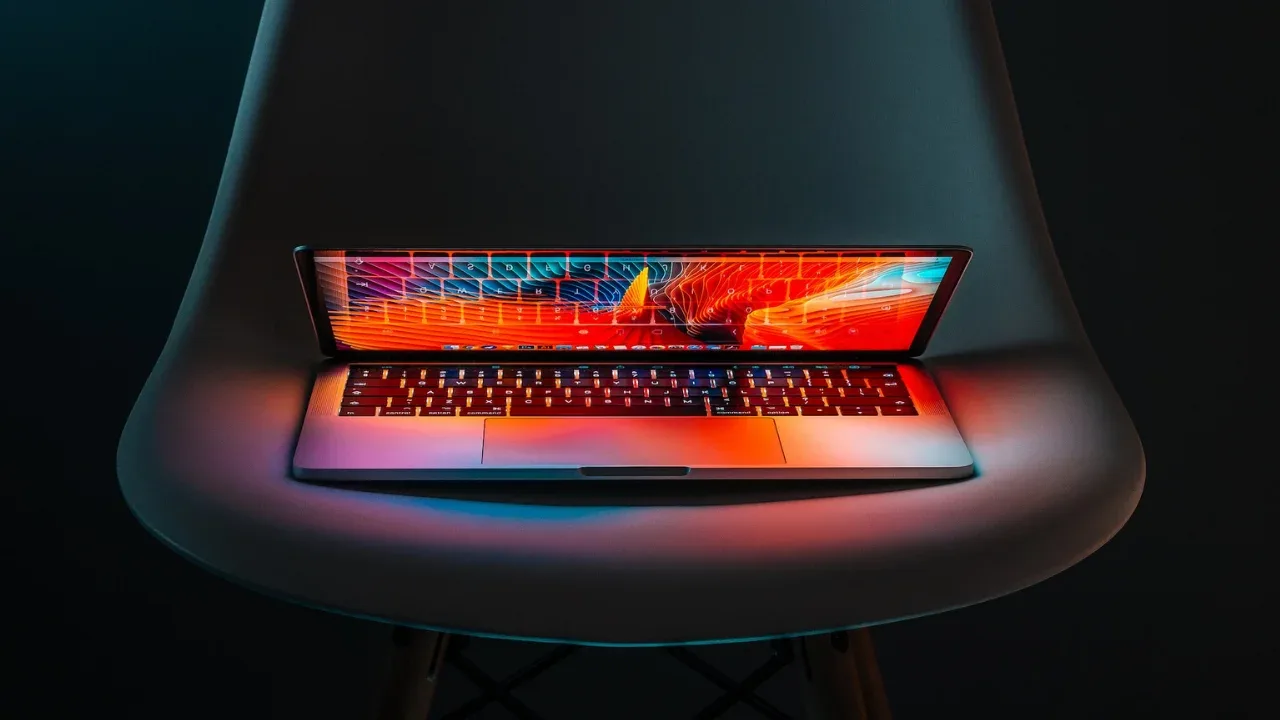
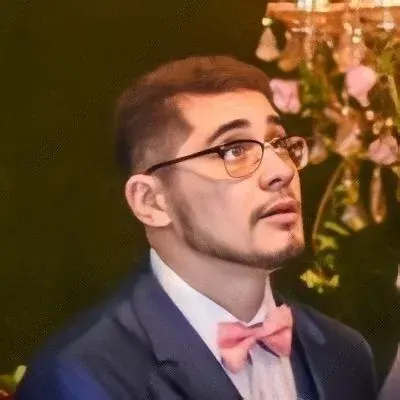
đ How to Get the File Extension in PHP? đ¤
So, you're trying to get the file extension of an image you're uploading in PHP. đŧī¸ But all you get is an array back. Don't worry, I've got you covered! In this blog post, I'll walk you through the common issues and provide easy solutions to fetch the file extension in PHP. Let's dive right in! đĒ
The Problem: Getting an Array Instead of Just the File Extension đ¤ˇââī¸
Here's the code snippet you provided, where you're trying to get the file extension:
$userfile_name = $_FILES['image']['name'];
$userfile_extn = explode(".", strtolower($_FILES['image']['name']));
But the result is an array and not just the extension itself. âšī¸
The Solution: Extracting Only the File Extension đ
To get just the file extension, you can use the pathinfo()
function in PHP. đ This function returns an associative array containing information about the file path, including the extension.
Here's how you can modify your code to get only the file extension:
$userfile_name = $_FILES['image']['name'];
$userfile_extn = pathinfo($userfile_name, PATHINFO_EXTENSION);
That's it! With this simple change, you can now fetch the file extension of the uploaded image. đ
A Complete Example and Explanation đ
Let me provide you with a complete example to make it crystal clear. đ
// Assuming you have a file input named 'image' in your HTML form
// Retrieve the file name from the uploaded image
$userfile_name = $_FILES['image']['name'];
// Extract just the file extension using pathinfo()
$userfile_extn = pathinfo($userfile_name, PATHINFO_EXTENSION);
// Print the file extension
echo "The file extension is: " . $userfile_extn;
So, when you upload an image with the name "myimage.jpg", the output will be:
The file extension is: jpg
Wrapping Up and Encouraging Your Feedback đđĸ
Now you know how to extract the file extension in PHP using the pathinfo()
function. It's a simple solution that saves you from the hassle of dealing with arrays and allows you to get just the extension you need. đ
If you found this blog post helpful, don't forget to share it with your fellow developers! And if you have any questions, suggestions, or additional tips, please let me know in the comments below. I'd love to hear from you! đ
Happy coding! đģđ