How to get body of a POST in php?
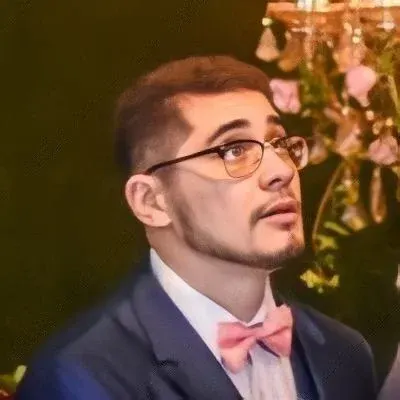
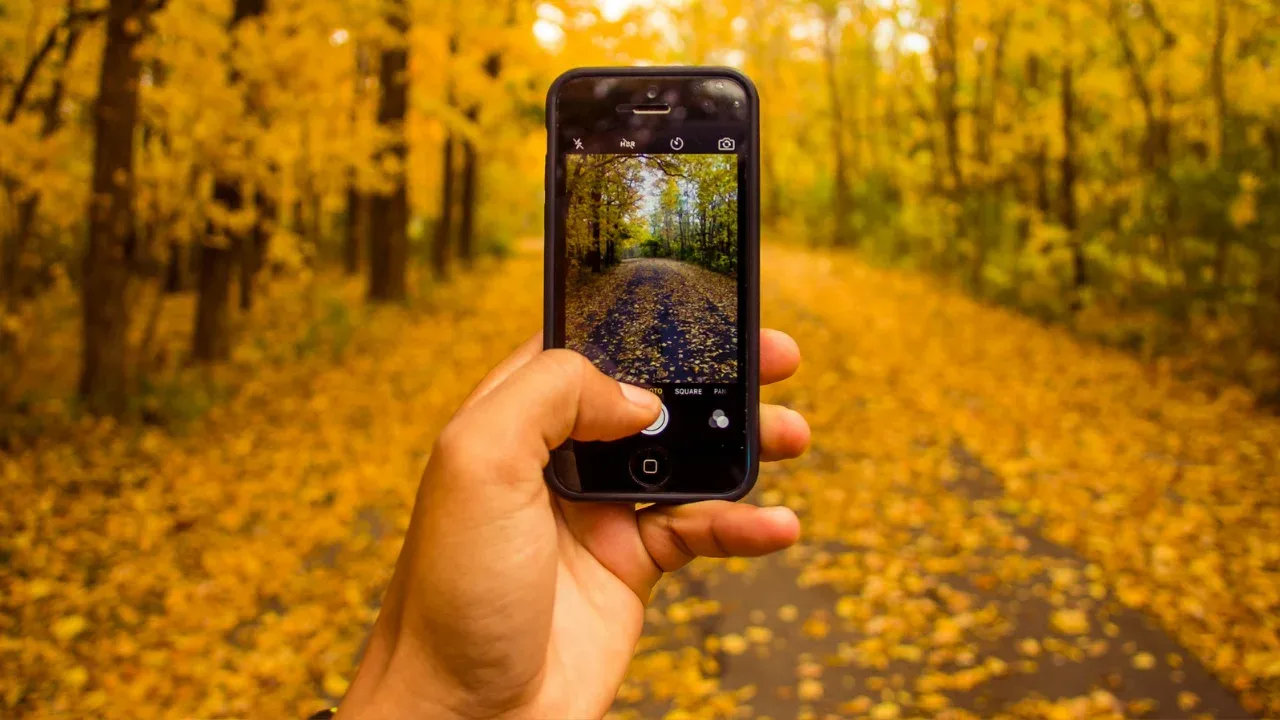
How to Get the Body of a POST in PHP? 📱
So, you have made a POST request to a PHP page and you are wondering how to extract the body of the request in PHP. 🤔 Don't worry, I've got you covered! In this blog post, I'll guide you through the common issues and provide you with easy solutions. Let's get started! 🚀
The Problem 👾
Let's say you have submitted the following JSON as a POST request body to a PHP page:
{ "a": 1 }
And you want to extract this value in PHP so that you can use it in your code. But when you use the var_dump($_POST)
function, it doesn't give you the desired result. 😫
The Solution 💡
To extract the body of a POST request in PHP, you need to use the php://input
stream. This stream allows you to read the raw HTTP request body data. Here's how you can do it:
$request_body = file_get_contents('php://input');
$data = json_decode($request_body, true);
Let's see what we did here. First, we used the file_get_contents()
function and passed 'php://input'
as the file name. This function reads the input stream and returns the raw data from the request body.
Next, we used the json_decode()
function to convert the raw data into a PHP array. The second parameter, true
, tells PHP to return an associative array instead of an object. This makes it easier to work with the data.
Now that you have the extracted data stored in the $data
variable, you can use it as desired in your PHP code. 🎉
Example Usage 🌟
Let's suppose you want to access the 'a'
value from the JSON data we submitted earlier. You can do it like this:
if (isset($data['a'])) {
$a = $data['a'];
// Do something with $a
} else {
// 'a' key not found in the JSON data
// Handle the error gracefully
}
In this example, we check if the 'a'
key exists in the $data
array using isset()
. If it exists, we assign its value to the $a
variable, and then you can perform any desired actions with it. If the key doesn't exist, you can handle the error gracefully to ensure a smooth user experience.
Conclusion ⏱️
Extracting the body of a POST request in PHP using the php://input
stream is a simple and effective solution. By following the steps outlined in this blog post, you can easily access the raw body data and use it as per your requirements.
Remember, when working with JSON data, don't forget to decode it using json_decode()
to convert it into a PHP array or object.
I hope this guide has helped you in solving the problem you were facing. If you have any further questions or suggestions, feel free to leave a comment below. Happy coding! 💻🎉
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
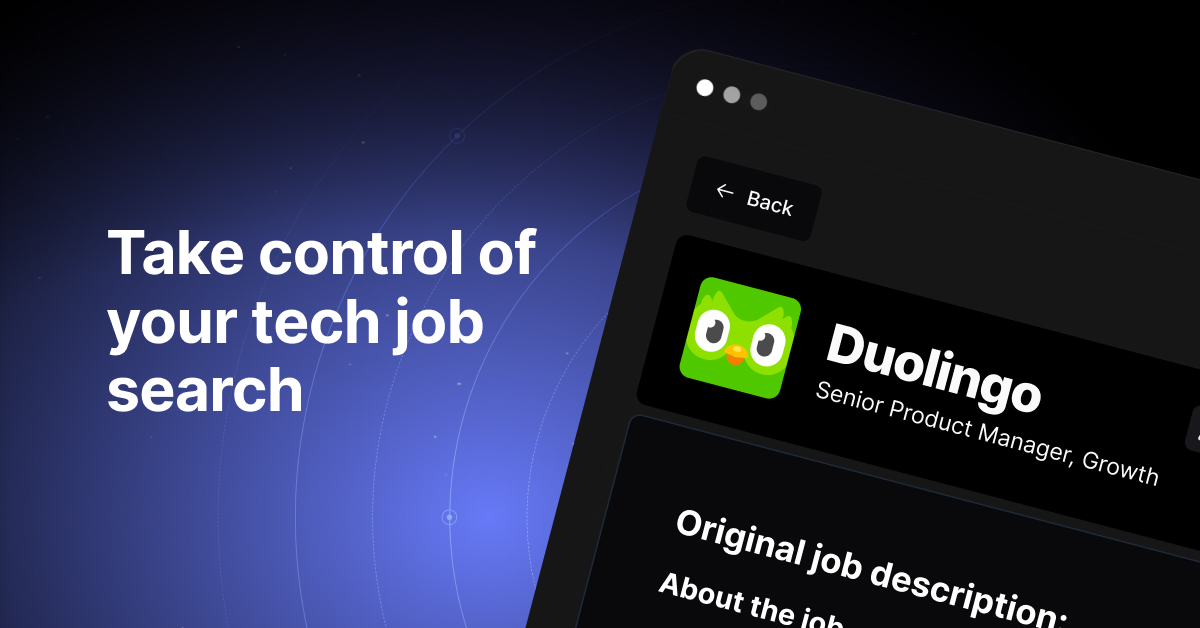