How to get all rows (soft deleted too) from a table in Laravel?
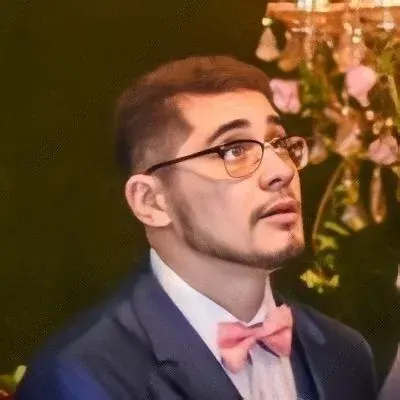
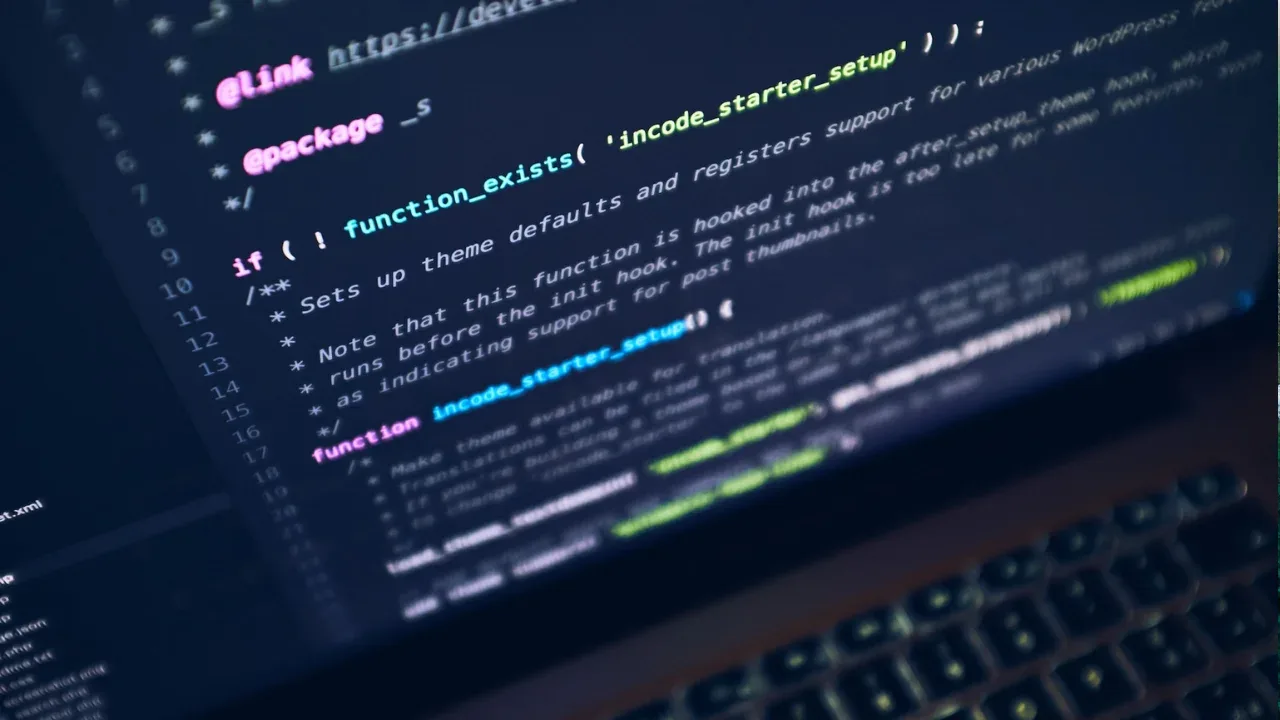
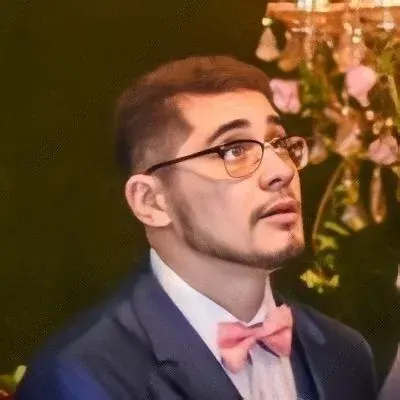
How to Get All Rows (Including Soft Deleted) from a Table in Laravel?
š Welcome to our blog! In this post, we will address a common issue that many Laravel developers face: retrieving all rows from a table, including the soft deleted ones. We believe that with a little bit of Eloquent magic, this problem will be solved in no time! So, let's dive in!
Understanding the Problem
By default, when we use Model::all()
, Laravel only fetches the non-soft deleted records from the table. However, there might be situations where we need to retrieve even the soft deleted rows. Luckily, Laravel's Eloquent ORM provides us with a simple solution.
Solution: Using withTrashed()
To get all rows from a table, including the soft deleted ones, we can use the withTrashed()
method provided by Eloquent. This method will instruct Laravel to also include the soft deleted records in the query result.
Here's an example of how to use withTrashed()
:
use App\Models\Model;
$allRows = Model::withTrashed()->get();
With this code, the $allRows
variable will contain both the non-soft deleted rows and the soft deleted rows from the table associated with the Model
class.
Understanding the withTrashed()
Method
The withTrashed()
method is part of Laravel's Soft Delete feature. When we enable soft delete on a model, instead of permanently deleting records, Laravel marks them as deleted by setting a deleted_at
timestamp column.
By default, when we retrieve records using the get()
method, Laravel applies a constraint to exclude any rows with a non-null deleted_at
column. However, by using withTrashed()
, we can disable this constraint and retrieve both the non-soft deleted and soft deleted records.
Adding a Where Clause to Retrieve Specific Rows
If you want to retrieve only specific rows (soft deleted or not), you can also combine withTrashed()
with other query constraints. Here's an example:
$specificRows = Model::withTrashed()
->where('column', 'value')
->get();
In this example, the where()
method is used to add a condition to the query, so we only get rows that match a certain column value. The withTrashed()
method ensures that both non-soft deleted and soft deleted rows meeting the condition are included.
Call-to-Action: Share Your Thoughts and Experiences
Have you ever struggled with retrieving all rows, including soft deleted ones, in Laravel? How did you solve it? We would love to hear your thoughts and experiences! Share them in the comments section below and let's start a conversation.
Thank you for reading! If you found this blog post helpful, don't forget to share it with your fellow Laravel developers. Happy coding! šš