How to generate XML file dynamically using PHP?
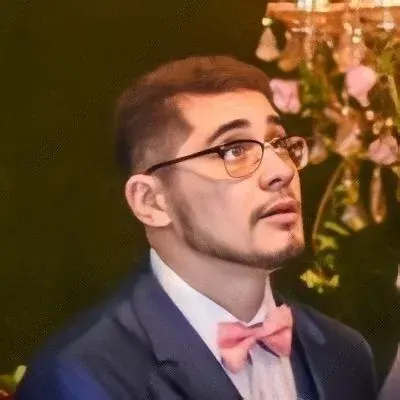
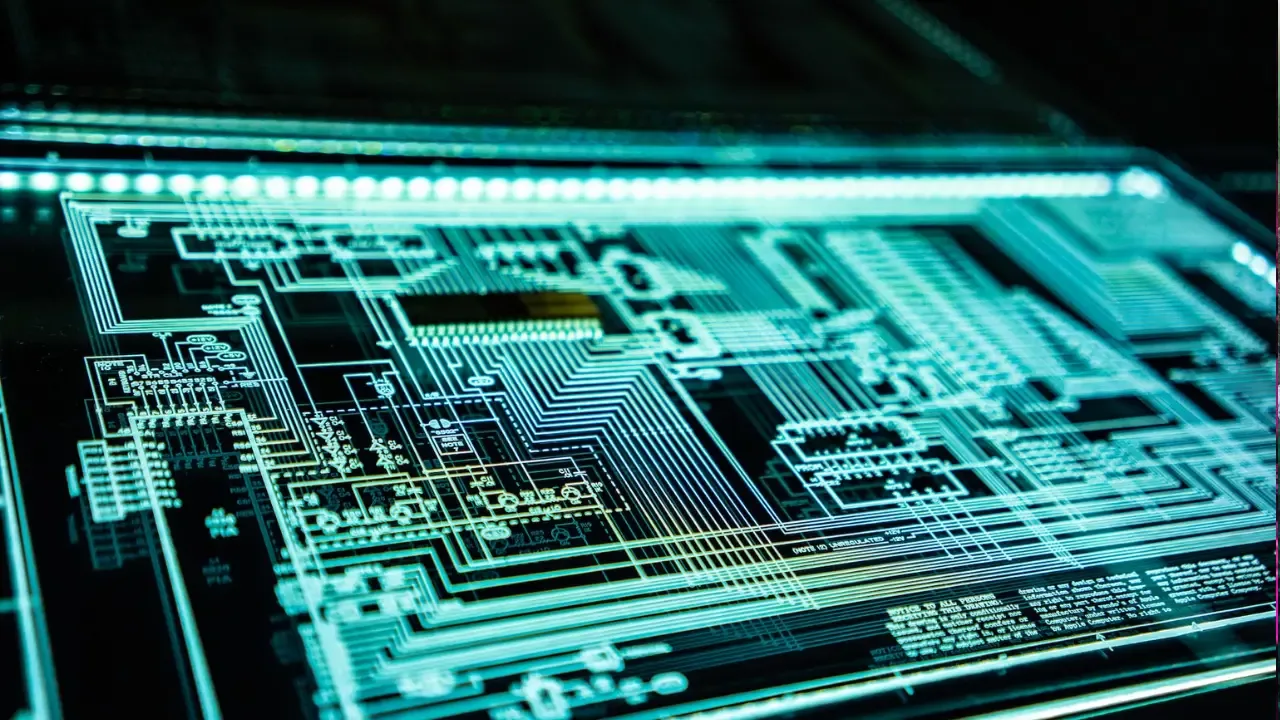
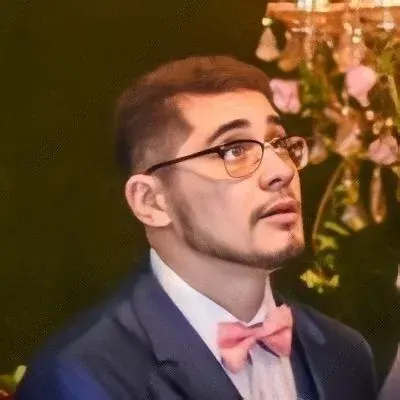
šµ Generating XML File Dynamically Using PHP šµ
š¤ Are you tired of manually creating XML files? šµ Do you need a solution to generate dynamic XML files on the fly using PHP? š¶ We've got you covered! In this guide, we'll walk you through step by step how to generate XML files dynamically using PHP. š
šÆ The Problem:
A user asked how to generate the XML file shown above dynamically using PHP. This can be a common requirement when working with XML-based APIs or when you need to create dynamic content for your applications.
š” The Solution:
To dynamically generate the XML file, we can use PHP's built-in SimpleXML extension. This extension provides a simple and intuitive way to create XML documents.
First, let's set up our PHP script. Create a new PHP file (e.g.,
generate_xml.php
) and open it in your favorite code editor.Now, let's start the XML creation process. In PHP, we can create a new SimpleXMLElement object to represent our XML document. We can also specify the XML version and encoding using the
addAttribute()
method:// Create the root XML element $xml = new SimpleXMLElement('<?xml version="1.0" encoding="UTF-8"?><xml></xml>');
Next, we need to add the
<track>
elements and their contents. We can achieve this by looping through the data and adding the elements dynamically. For example, let's assume we have an array called$tracks
containing information about each track:// Loop through the tracks array foreach ($tracks as $track) { // Create a new track element $trackElement = $xml->addChild('track'); // Add the path element to the track $trackElement->addChild('path', $track['path']); // Add the title element to the track $trackElement->addChild('title', $track['title']); }
Finally, let's output the XML content. We can use the
asXML()
method to convert the XML object into a string and then send it to the browser using the appropriate headers:// Set the Content-Type header header('Content-Type: text/xml'); // Output the XML content echo $xml->asXML();
That's it! š With these steps, you can dynamically generate the XML file using PHP. Remember to customize the code to fit your specific requirements.
š Conclusion:
In this guide, we've walked through the process of generating XML files dynamically using PHP. We used the SimpleXML extension to create the XML document, added the necessary elements, and outputted the result. Now, you have the power to generate dynamic XML files with ease!
Next time you need to generate XML files dynamically using PHP, remember these steps and enjoy the simplicity and flexibility of creating XML on the fly.
ā Have any questions or encountered any issues? Let us know in the comments below. š We're here to help!
Keep generating dynamic XML files like a rockstar! š¤
Ready to rock your code? Head over to our blog to discover more exciting tutorials and guides. š
Stay tuned for more tips, tricks, and code snippets! š
Happy coding! š