How to extract and access data from JSON with PHP?
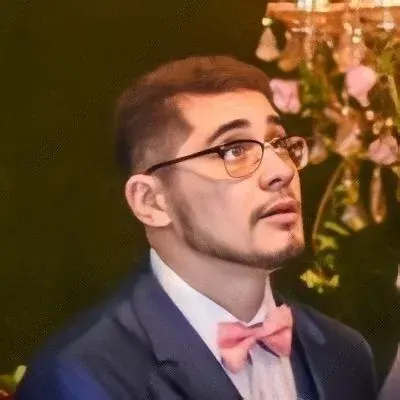
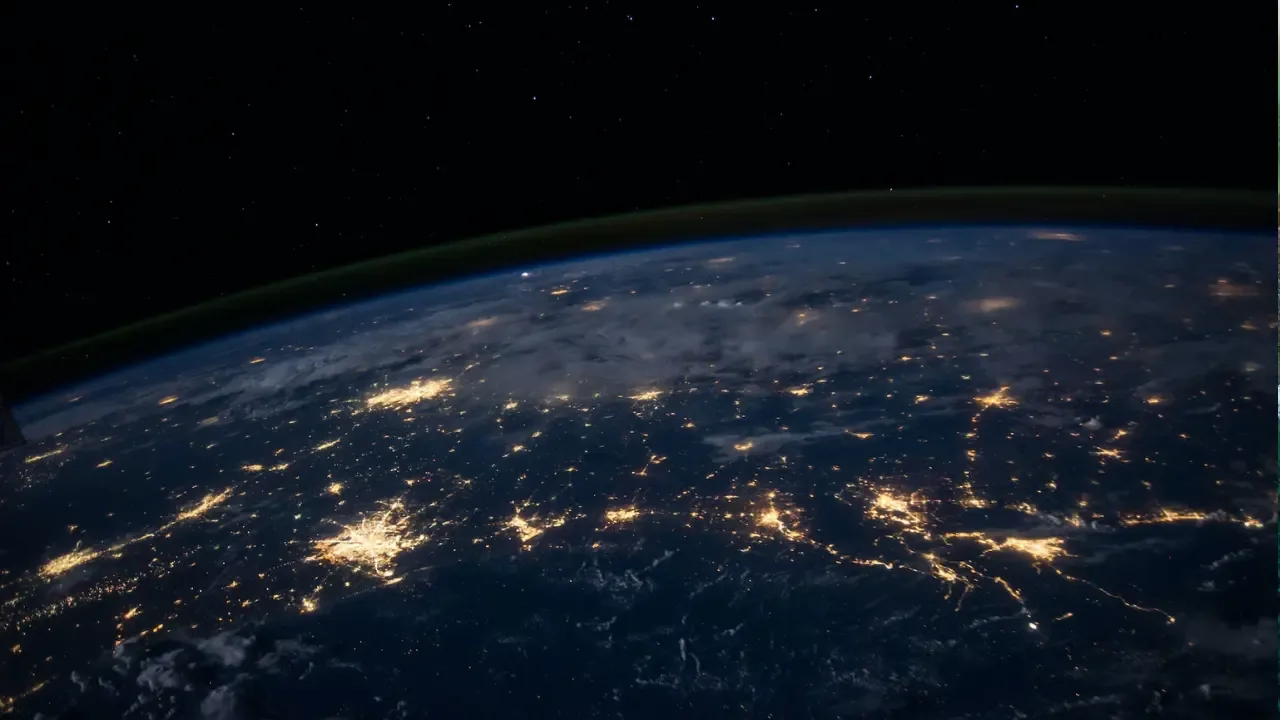
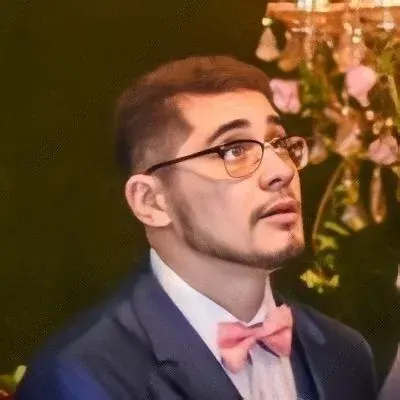
How to Extract and Access Data from JSON with PHP?
š Hey there, tech enthusiasts! Are you struggling to decode and access data from JSON using PHP? š¤ Don't worry, you've come to the right place! In this guide, we'll tackle this common issue head-on and provide you with easy solutions to help you make the most out of your JSON data. š
The JSON Example šÆ
Let's start by taking a look at an example JSON that we'll be working with throughout this guide:
{
"type": "donut",
"name": "Cake",
"toppings": [
{ "id": "5002", "type": "Glazed" },
{ "id": "5006", "type": "Chocolate with Sprinkles" },
{ "id": "5004", "type": "Maple" }
]
}
Decoding JSON in PHP š§©
Before we can access the data, we need to decode the JSON using PHP's json_decode()
function. This function takes the JSON string as input and returns a PHP object or an associative array, depending on our needs.
$jsonString = '{
"type": "donut",
"name": "Cake",
"toppings": [
{ "id": "5002", "type": "Glazed" },
{ "id": "5006", "type": "Chocolate with Sprinkles" },
{ "id": "5004", "type": "Maple" }
]
}';
$jsonData = json_decode($jsonString);
š” Pro Tip: If you want to decode the JSON into an associative array, pass true
as the second parameter to json_decode()
. For example: json_decode($jsonString, true);
Accessing JSON Data šļø
Now that we have our decoded JSON data, we can easily access its elements using the arrow (->
) or square brackets ([]
) notation. Let's dive into a few examples to demonstrate how it works. šµļøāāļø
Accessing Top-Level Elements
To access the top-level elements like "type"
and "name"
, we can use the arrow notation:
$type = $jsonData->type; // Output: "donut"
$name = $jsonData->name; // Output: "Cake"
Accessing Nested Elements
For accessing nested elements like "toppings"
, we can chain the arrow or square brackets notation:
$firstToppingId = $jsonData->toppings[0]->id; // Output: "5002"
$secondToppingType = $jsonData->toppings[1]->type; // Output: "Chocolate with Sprinkles"
Easy, right? š You can access any level of nested elements in a similar way.
š” Pro Tip:
If you're working with an associative array instead of an object, you can access the elements using the square brackets notation:
$jsonData = json_decode($jsonString, true);
$type = $jsonData['type']; // Output: "donut"
$name = $jsonData['name']; // Output: "Cake"
$firstToppingId = $jsonData['toppings'][0]['id']; // Output: "5002"
$secondToppingType = $jsonData['toppings'][1]['type']; // Output: "Chocolate with Sprinkles"
Ready to Dive In? šāāļø
You're now equipped with the knowledge to extract and access data from JSON using PHP! We hope this guide has been helpful and you feel confident in working with JSON in your PHP projects. If you still have any questions or face any issues, feel free to reach out to us. We'd be more than happy to assist you! šŖ
Don't forget to stay connected with us for more exciting tech guides and tutorials. Until next time, happy coding! ššØāš»