How to do this in Laravel, subquery where in
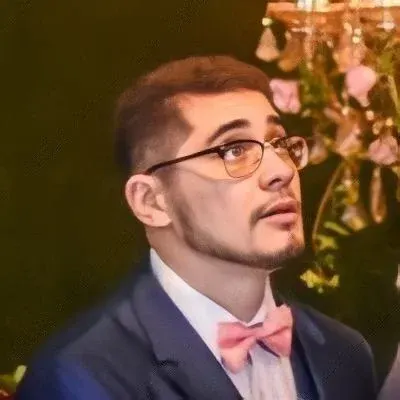
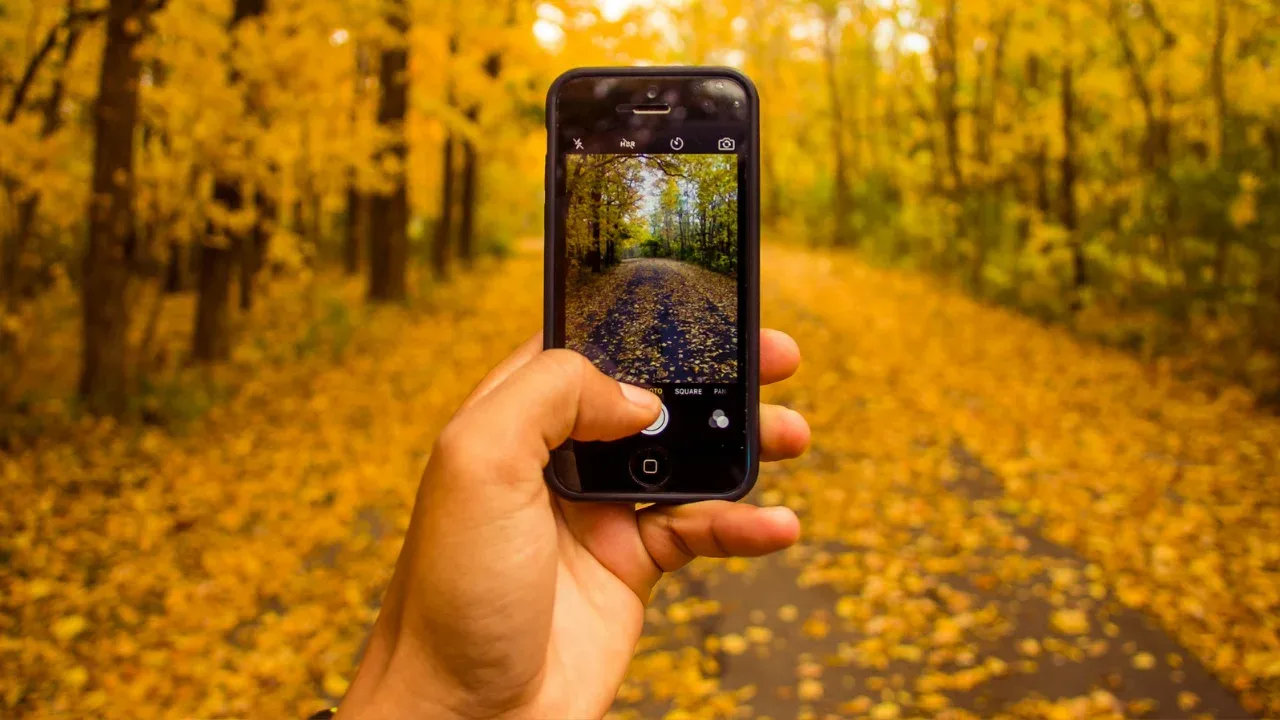
🔥 Title: Mastering Laravel: Harnessing the Power of Subquery WHERE IN
🔥
Hey there, fellow Laravel enthusiast! Need help tackling a complex subquery issue in Laravel? Look no further! In this guide, we'll explore how to write efficient queries using the subquery WHERE IN
technique. We'll address common problems and provide easy, performance-focused solutions that will leave you feeling like a Laravel wizard. Let's dive in! 💪💻
The Challenge: Crafting a Query in Laravel with a Subquery WHERE IN
✨
A user on the Laravel forum had a query they wanted to execute in Laravel, but they required a specific format to optimize performance. The original SQL query looked like this:
SELECT
`p`.`id`,
`p`.`name`,
`p`.`img`,
`p`.`safe_name`,
`p`.`sku`,
`p`.`productstatusid`
FROM `products` p
WHERE `p`.`id` IN (
SELECT
`product_id`
FROM `product_category`
WHERE `category_id` IN ('223', '15')
)
AND `p`.`active` = 1
The Solution: Leveraging Laravel's Eloquent ORM to Tackle the Subquery WHERE IN
Challenge 🚀
To achieve the desired outcome, we can leverage the power of Laravel's Eloquent ORM. With a few tweaks, we'll not only get our desired results but also optimize our performance. 😎🚀
Here's the Laravel code to achieve the same result as the original SQL query:
use App\Models\Product;
use App\Models\ProductCategory;
$desiredCategories = ['223', '15'];
$products = Product::whereIn('id', function ($query) use ($desiredCategories) {
$query->select('product_id')
->from('product_category')
->whereIn('category_id', $desiredCategories);
})
->where('active', 1)
->get(['id', 'name', 'img', 'safe_name', 'sku', 'productstatusid']);
By utilizing the whereIn
method within the Eloquent ORM, we can perform subquery operations directly within our Laravel code. This not only simplifies our codebase but also optimizes performance by reducing the number of database queries. 🏋️
The Optimized Solution: Embracing Joins for Improved Performance ⚡
While Laravel's Eloquent ORM provides a sleek solution with the subquery WHERE IN
technique, you mentioned that you could also use a join for improved performance. Let me show you how to achieve this optimization.
use App\Models\Product;
use App\Models\ProductCategory;
$desiredCategories = ['223', '15'];
$products = Product::join('product_category', 'product_category.product_id', '=', 'products.id')
->whereIn('product_category.category_id', $desiredCategories)
->where('products.active', 1)
->get(['products.id', 'products.name', 'products.img', 'products.safe_name', 'products.sku', 'products.productstatusid']);
By using a join operation, we reduce the number of queries executed against the database, resulting in better performance. However, this solution might be less flexible depending on your specific requirements.
Take It to the Next Level: Learn, Experiment, and Thrive 🔝
Now that you've mastered the art of subquery WHERE IN
in Laravel, it's time to put your newfound knowledge to work. Experiment with different scenarios, explore additional Laravel query techniques, and watch your skills flourish! 💡💪
💬 We Want to Hear From You!
Have you encountered a similar challenge or faced other Laravel hurdles? Share your experiences and insights in the comments below. Our vibrant community thrives on knowledge sharing and mutual growth. Let's connect! 🤝🌐
Remember, great developers never stop learning and pushing boundaries. Embrace the Laravel ecosystem, experiment fearlessly, and unlock the true potential within you! Happy coding! 🚀💻
═══════════════════════════════════════════════════════
📚 Incorporate your CTA here:
If you found this guide helpful and want to learn more about Laravel's powerful features, stay connected with us! Subscribe to our newsletter for regular Laravel tips, tricks, and updates. Be part of our thriving community and accelerate your Laravel journey! 💌🎉
✨ Subscribe Now! ✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
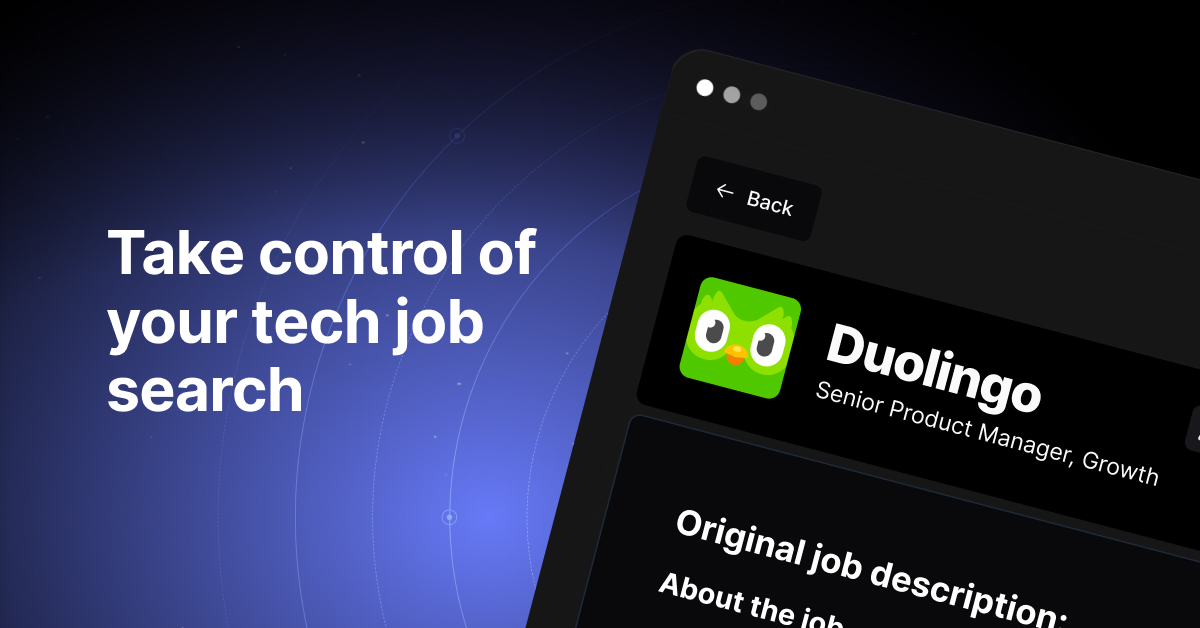