How to create a laravel hashed password
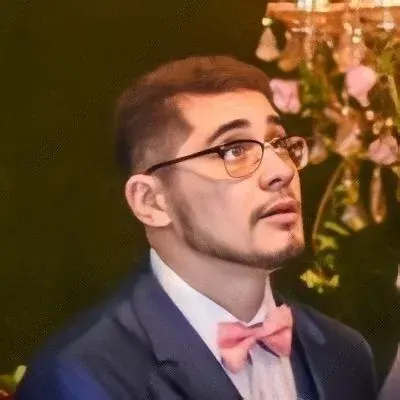
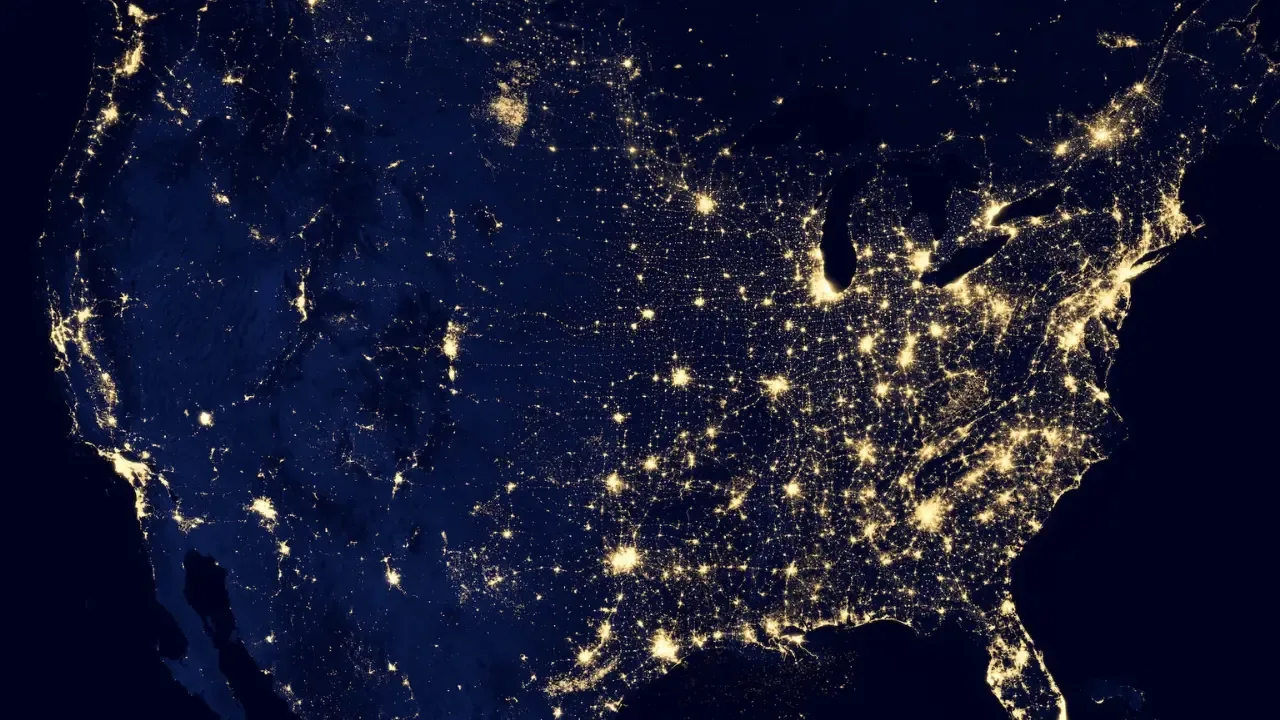
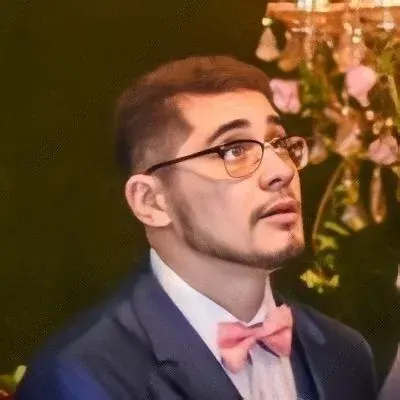
How to Create a Laravel Hashed Password Like a Pro! 😎
Have you ever wondered how to securely hash passwords in your Laravel application? Look no further! In this guide, I'll show you how to create a hashed password using Laravel's hash helper. 🔒
The Problem: Can't Find the Laravel Hash Helper?
So you want to use the Laravel hash helper, but you can't seem to find it? Don't worry, you're not alone! 🤷♀️
The Laravel hash helper is actually a built-in function that you can access anywhere in your Laravel application. It's not a separate package or a hidden gem. You get it out-of-the-box! 😁
The Solution: Using the Laravel Hash Helper
To create a hashed password, follow these simple steps:
Step 1: Import the Hash Facade at the top of your file:
use Illuminate\Support\Facades\Hash;
Step 2: Call the make
method of the Hash facade and pass in the plain text password:
$hashedPassword = Hash::make('your_plain_text_password');
That's it! Laravel will automatically hash the password for you. 🚀
But wait, there's more! You mentioned that you want to manually insert the hashed password into the database. Fear not! Laravel has got your back. Just store the $hashedPassword
variable in your database and you're good to go! 💪
Let's Put It Into Action:
Suppose you have a registration form in your Laravel application and you want to hash the user's password before storing it in the database. Here's an example of how you can achieve that:
use Illuminate\Support\Facades\Hash;
use App\Models\User;
public function register(Request $request)
{
// Validate the request...
// Hash the password
$hashedPassword = Hash::make($request->input('password'));
// Create a new user
$user = new User;
$user->name = $request->input('name');
$user->email = $request->input('email');
$user->password = $hashedPassword;
$user->save();
// Redirect to the home page or do something else
}
Call-to-Action: Share Your Thoughts and Spread the Knowledge! 💬
Now that you know how to create a Laravel hashed password, it's time to put your knowledge into action! I encourage you to try it out in your own projects and see how it enhances your application's security. And don't forget to share your thoughts and experiences in the comments below! Let's grow together as a community of Laravel enthusiasts! 🌱
Happy coding! 💻🔒