How to convert string to boolean php
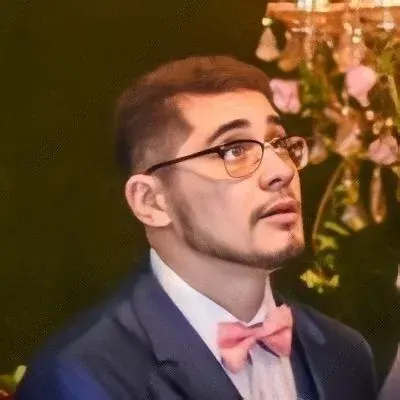
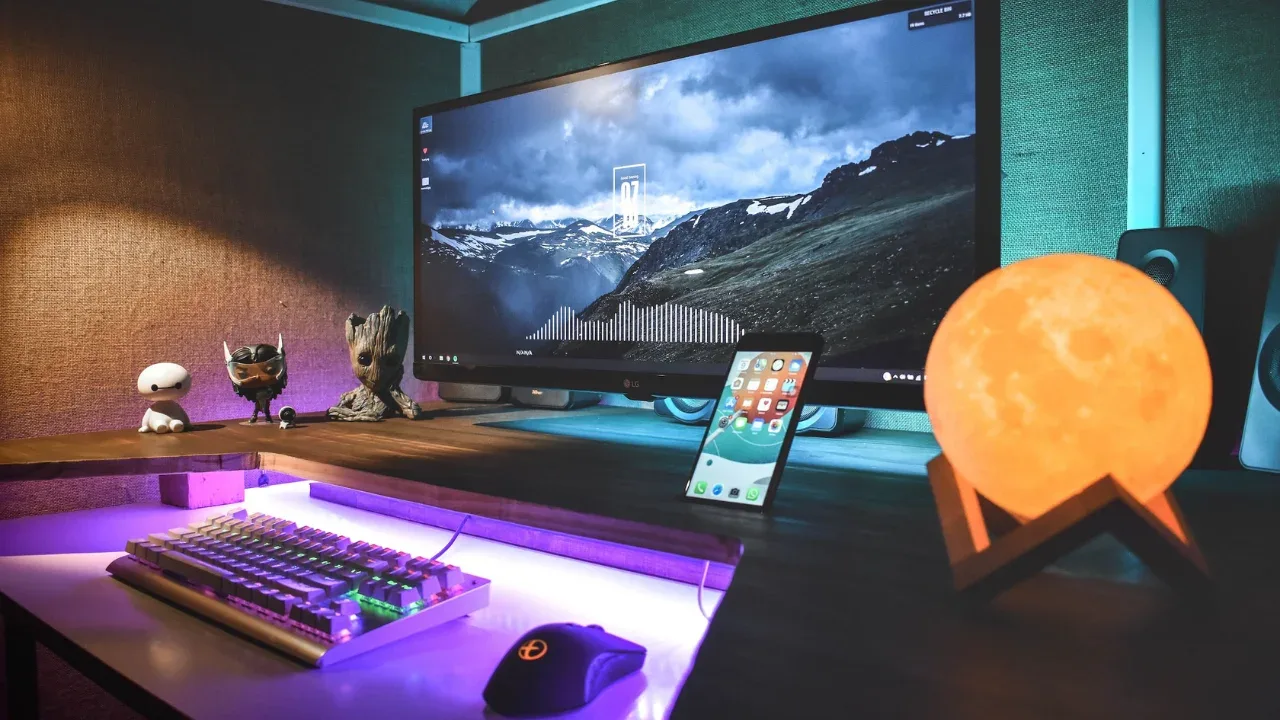
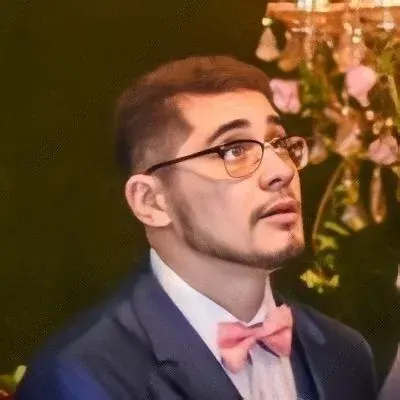
How to Convert String to Boolean in PHP: A foolproof guide 🚀
If you've ever found yourself scratching your head trying to convert a string to a boolean in PHP, you're not alone! This seemingly simple task can sometimes lead to unexpected results and frustration. But fear not! In this blog post, we'll dive into the common issues and provide you with easy solutions to get the correct boolean value. Let's get started! 💪
The Problem: String to Boolean Conversion
Consider the following code snippet:
$string = 'false';
$test_mode_mail = settype($string, 'boolean');
var_dump($test_mode_mail);
if($test_mode_mail) echo 'test mode is on.';
The expected output should be boolean false
, but instead, we get boolean true
. Why does this happen? Let's break it down.
Understanding the Issue
In PHP, the settype()
function attempts to convert a variable to a specified type. However, when converting a string to a boolean, PHP follows a particular set of rules that can lead to unexpected results.
By default, any non-empty string in PHP is evaluated as true
. So, when you settype the string 'false'
to a boolean type, it evaluates to true
because it is not an empty string.
Solution 1: Using the filter_var() Function
A reliable way to convert a string to a boolean in PHP is by using the filter_var()
function. Here's an example:
$string = 'false';
$test_mode_mail = filter_var($string, FILTER_VALIDATE_BOOLEAN);
var_dump($test_mode_mail);
if($test_mode_mail) echo 'test mode is on.';
This time, the output will be boolean false
. The FILTER_VALIDATE_BOOLEAN
filter accepts various string representations of boolean values, such as 'true'
, '1'
, 'on'
, and more. If the provided string matches any of these, it will be converted to true
. Otherwise, it will be converted to false
.
Solution 2: Manual Conversion
If you prefer a more straightforward approach, you can manually convert the string to a boolean using a conditional statement. Here's an example:
$string = 'false';
$test_mode_mail = ($string === 'true');
var_dump($test_mode_mail);
if($test_mode_mail) echo 'test mode is on.';
In this case, the output will also be boolean false
. By comparing the string with the value 'true'
, we get the desired boolean result. If the string is equal to 'true'
, the variable will be set to true
. Otherwise, it will be set to false
.
Your Turn to Try!
Now that you know how to convert a string to a boolean in PHP, it's time to test it out in your code! Feel free to experiment with different strings or implement these solutions in your own projects. Don't forget to share your experience and any questions you have in the comments below!
Conclusion
Converting a string to a boolean in PHP doesn't have to be confusing or frustrating. By understanding the default string evaluation rules and using the filter_var()
function or manual comparison, you can ensure that your boolean conversion works as expected.
Remember, if you encounter any issues or have any questions, feel free to reach out to our community or drop a comment below. Happy coding! 😄👩💻👨💻
🔗 Visit our website for more PHP tips and tricks.
📢 Have you ever struggled with converting a string to a boolean in PHP? Share your experiences in the comments below and help your fellow developers!