How to convert an array to object in PHP?
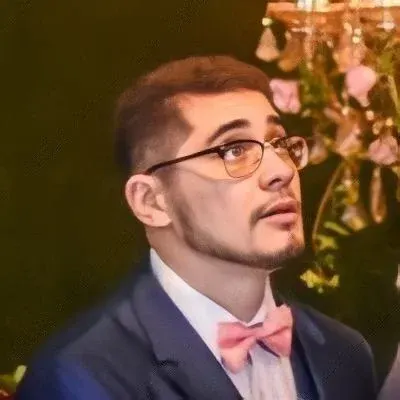
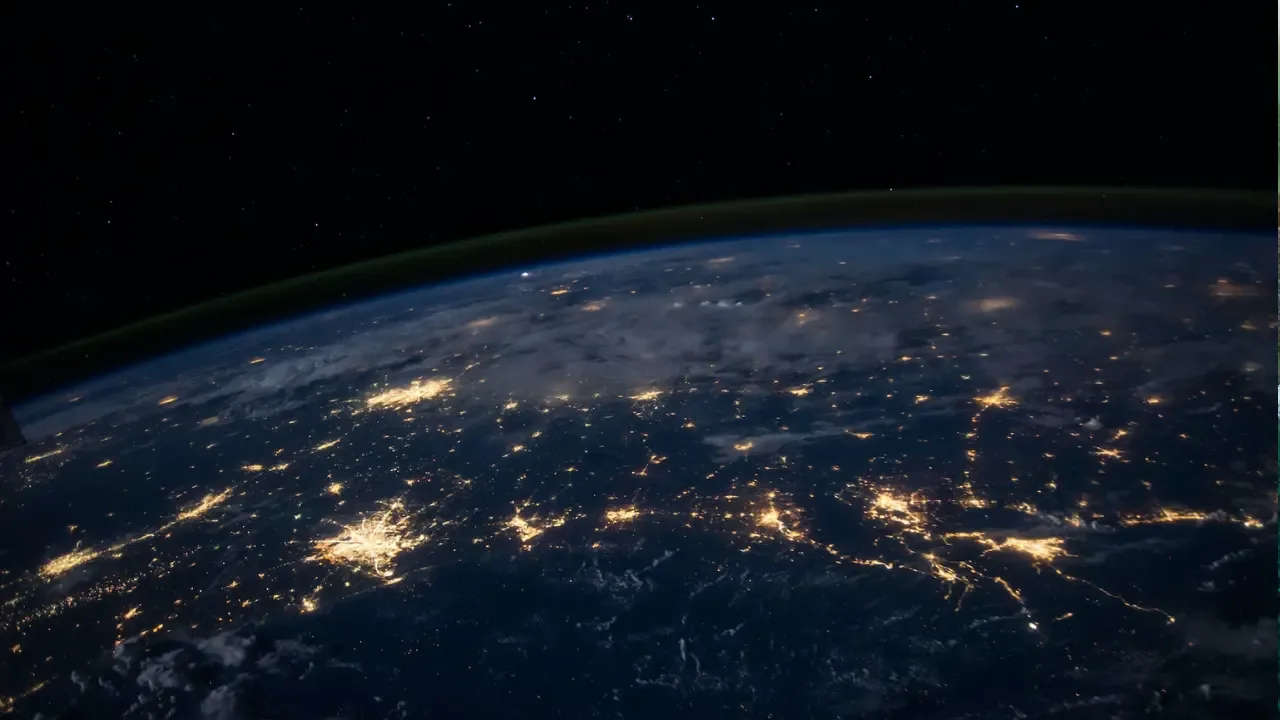
How to Convert an Array to Object in PHP? 😎🔀
Have you ever encountered the challenge of needing to convert an array to an object in PHP? 🤔 It may seem like a daunting task at first, but fear not! In this blog post, we will walk you through the process step by step, so you can convert arrays to objects like a pro. 🚀
The Challenge 🤷♂️
Let's take a look at a common issue faced by many PHP developers. Imagine having an array like this:
[
128 => [
'status' => 'Figure A. Facebook\'s horizontal scrollbars showing up on a 1024x768 screen resolution.'
],
129 => [
'status' => 'The other day at work, I had some spare time'
]
]
Now, the question is: How can we convert this array to an object? 🤔
The Easy Solution 💡
Luckily, PHP provides a simple and elegant solution for this problem using the stdClass
class. All we need to do is iterate through the array and cast each element as an object. Let's dive into the code! 💻
$array = [
128 => [
'status' => "Figure A. Facebook's horizontal scrollbars showing up on a 1024x768 screen resolution."
],
129 => [
'status' => 'The other day at work, I had some spare time'
]
];
$object = (object) $array;
And just like that, our array is magically transformed into an object! 🎩✨
Why Use stdClass
? 🤔
You might be wondering why we cast the array to stdClass
specifically. Well, stdClass
is a built-in class in PHP that serves as a generic empty object. It allows us to dynamically add properties to it, which makes it perfect for our array-to-object conversion needs. 🏗
A Real-World Example 🌍
To further illustrate the process, let's consider a real-world example. Imagine we are dealing with JSON data returned from an API (e.g., Facebook statuses), which we've converted to an array using json_decode()
. To work with the data more effectively, we want to convert it to an object. Here's how we can do it: 🔍
$jsonData = '[{"status":"Figure A. Facebook\'s horizontal scrollbars showing up on a 1024x768 screen resolution."},{"status":"The other day at work, I had some spare time"}]';
$array = json_decode($jsonData, true);
$object = (object) $array;
// Now, we can access the properties as if they were object attributes
echo $object->{0}->status; // Output: "Figure A. Facebook's horizontal scrollbars showing up on a 1024x768 screen resolution."
echo $object->{1}->status; // Output: "The other day at work, I had some spare time"
Your Turn! 🎉
Now that you have learned the art of converting arrays to objects in PHP, it's time to put your skills into action! 🚀✨
Take a moment to experiment with the code examples we provided, and see how arrays can be transformed into objects effortlessly. 💡💻
If you have any questions or cool ideas on how to utilize this technique, make sure to share them in the comments below. We'd love to hear from you! 💬😊
Remember: mastering array-to-object conversion opens up a world of possibilities and simplifies your PHP development journey. Don't be afraid to unleash your creativity! 🌟
Now, go forth and start converting those arrays to objects! Happy coding! 👩💻👨💻
References:
Note: The examples and code snippets provided in this blog post are for demonstration purposes only. They may not cover all edge cases, and it's always advised to validate and adapt the code to your specific needs.
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
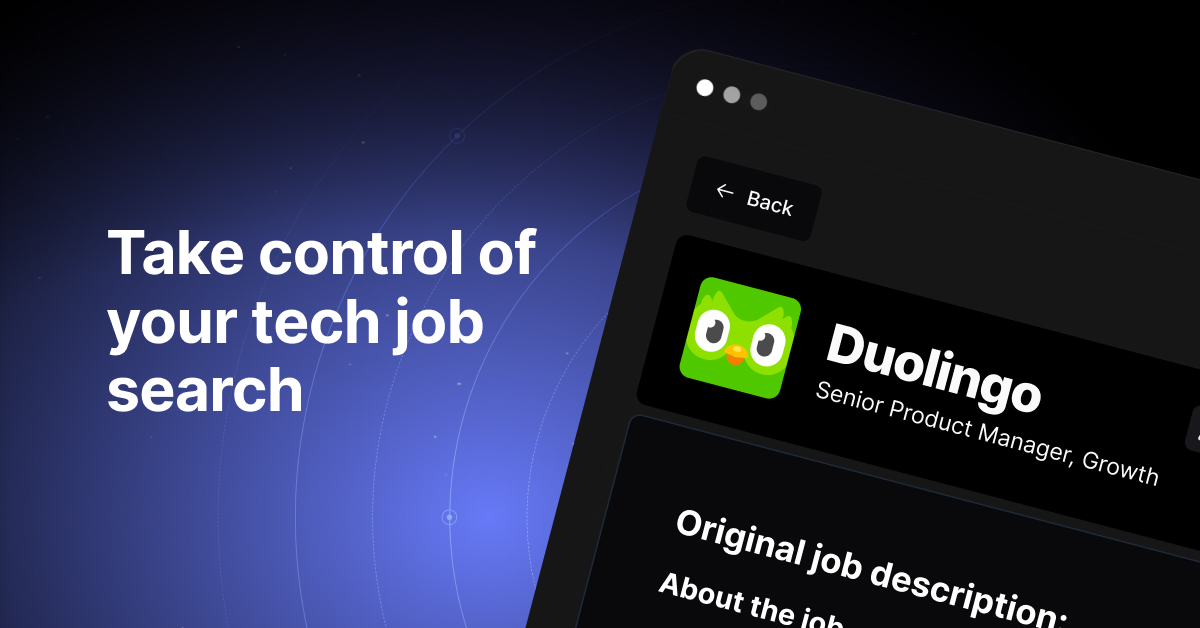