How to alias a table in Laravel Eloquent queries (or using Query Builder)?
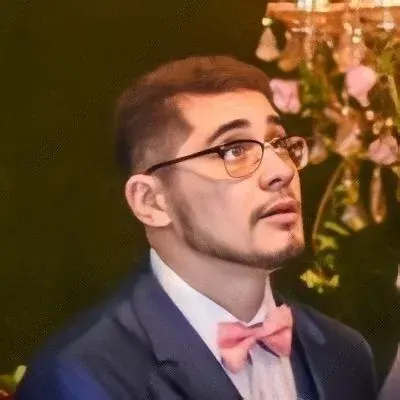
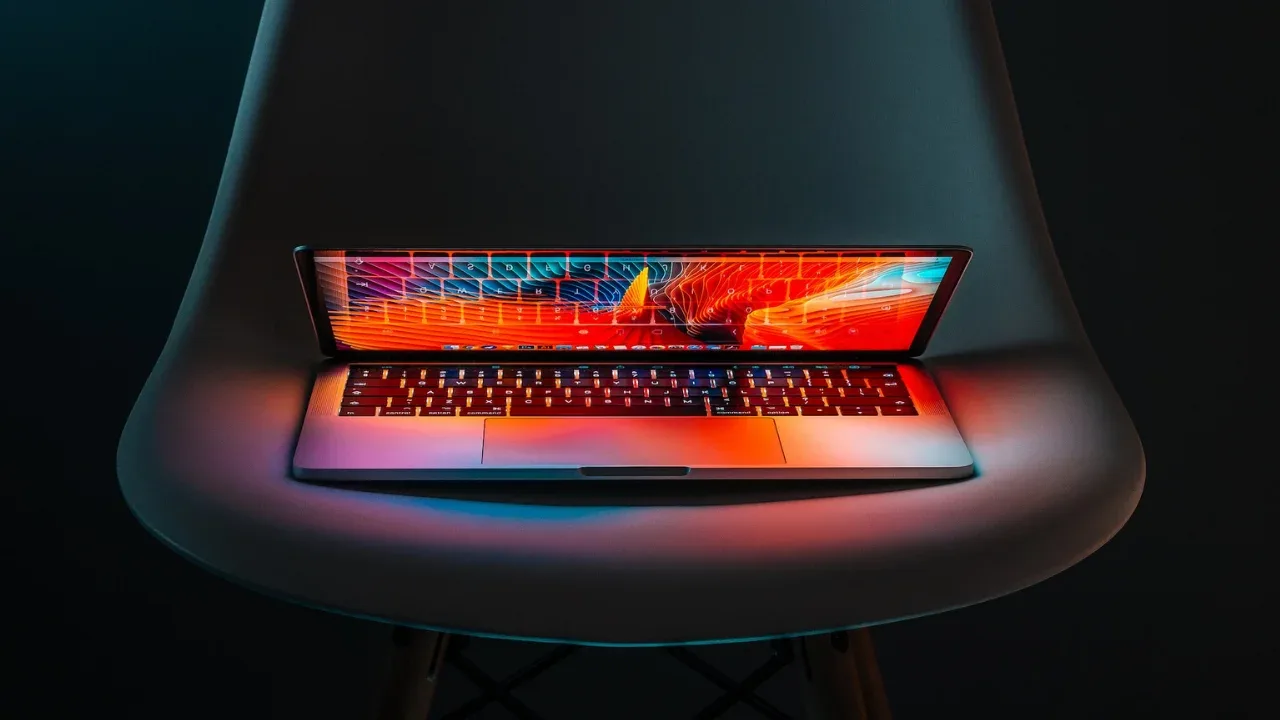
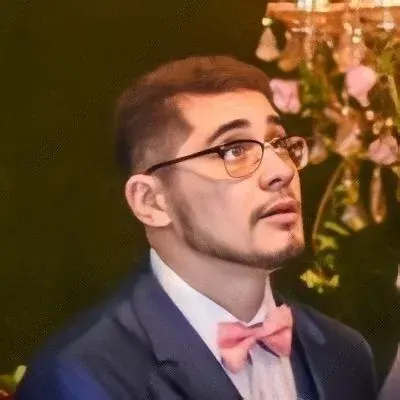
How to alias a table in Laravel Eloquent queries (or using Query Builder)?
Are you tired of typing out long table names in your Laravel Eloquent queries? Do you find your code becoming less readable because of it? Well, fret no more! In this blog post, we'll explore how you can alias a table in Laravel Eloquent queries using the Query Builder.
The Problem
Let's start by understanding the problem. In Laravel, when using the Query Builder, you may come across situations where you have a table with a really long name. Typing out this long name repeatedly in your queries can be time-consuming and make your code harder to read and maintain.
For example, consider the following query:
$users = DB::table('really_long_table_name')
->select('really_long_table_name.id')
->get();
Here, the table name 'really_long_table_name'
is used multiple times in the query, which can be a hassle. Wouldn't it be great if we could alias this table and use a shorter name instead?
The Solution
Fortunately, Laravel provides a simple solution for aliasing tables in Eloquent queries using the as
method. You can chain this method to specify an alias for the table.
Let's modify our previous example to use table aliasing:
$users = DB::table('really_long_table_name as short_name')
->select('short_name.id')
->get();
In this modified query, we've aliased the table 'really_long_table_name'
as 'short_name'
. Now, instead of typing out the long table name, we can simply use the alias 'short_name'
in our query.
Not only does this make your code more readable, but it also reduces the amount of typing required. 🚀
Using Aliases in Column Aliases
Table aliases can also be used in column aliases. This can be handy when you want to include the alias in the result array.
For example, let's modify our previous query to include a column alias:
$users = DB::table('really_long_table_name as short_name')
->select('short_name.id as user_id')
->get();
In this modified query, we've aliased the column 'id'
as 'user_id'
and included the table alias 'short_name'
as well. This way, the resulting array will have a 'user_id'
key to access the user's ID value.
Conclusion
Alias your tables in Laravel Eloquent queries using the Query Builder to make your code more readable and reduce typing. With the as
method, you can easily assign a shorter alias to your tables, saving you time and making your code cleaner.
So, the next time you find yourself typing out a long table name repeatedly, remember the power of table aliasing in Laravel. Give it a try and see how it improves the readability and maintainability of your code.
Do you have any other tips or tricks for optimizing Laravel queries? Share them with us in the comments below! 😊