How does PHP "foreach" actually work?
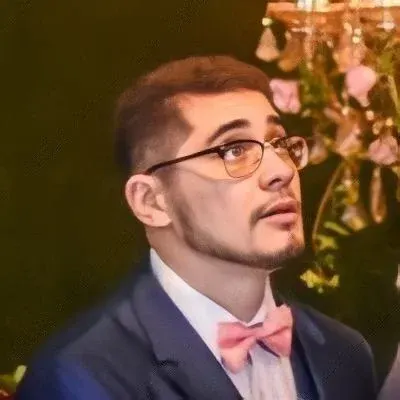
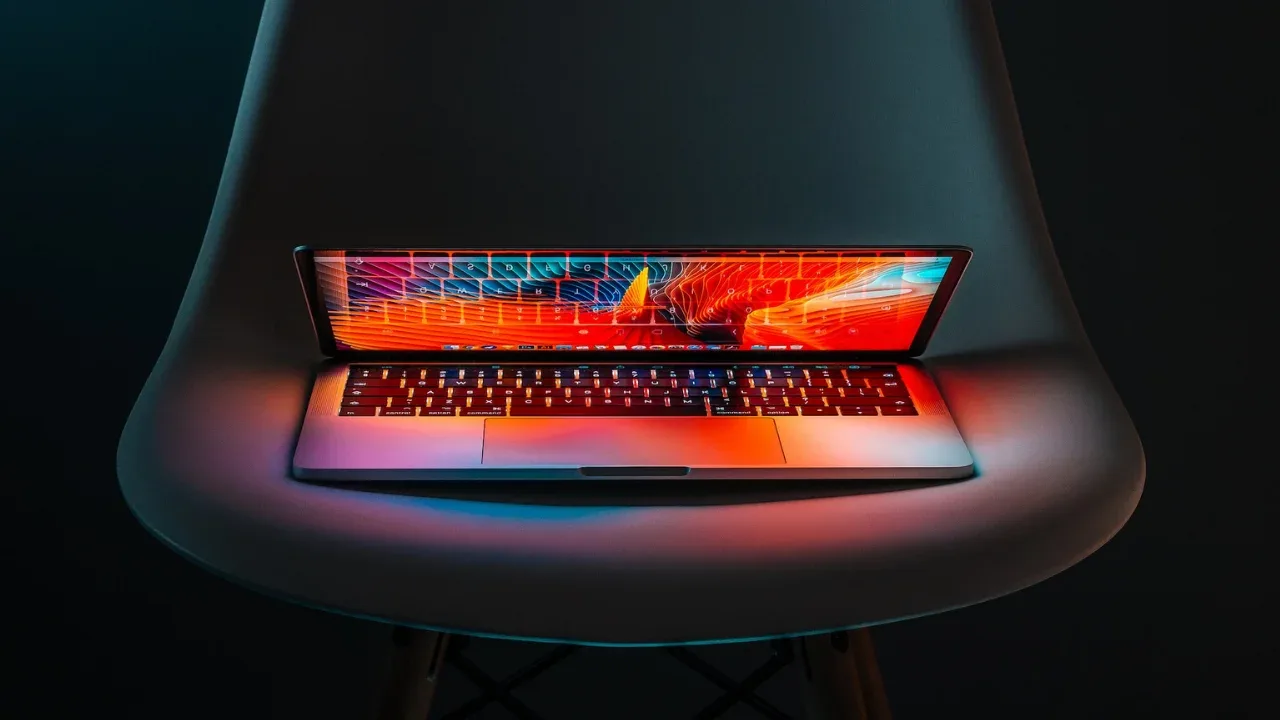
How does PHP 'foreach' actually work? 🤔
<p>So you think you know how to use the 'foreach' loop in PHP, huh? Well, buckle up because we're about to dive into the inner workings of this powerful loop. 🚀</p>
<p>Before we get started, let's quickly recap what 'foreach' does. It's a loop specifically designed for iterating over arrays and objects. It allows you to effortlessly loop through each element and perform actions or operations on them. But how exactly does it work behind the scenes? Let's find out! 🧐</p>
The 'foreach' Loop and the Copy Conundrum 😕
<p>We often hear that 'foreach' works with a <em>copy</em> of the array, which makes sense to prevent infinite loops when modifying the array during iteration. But recent discussions and experiments have led to some interesting discoveries that challenge this assumption. Let's take a closer look at a few test cases. 💡</p>
Test Case 1: Modifying the Array within the Loop 💥
<pre><code>foreach ($array as $item) { echo "$item\n"; $array[] = $item; } print_r($array); /* Output in loop: 1 2 3 4 5 $array after loop: 1 2 3 4 5 1 2 3 4 5 */ </code></pre>
<p>In this test case, we can clearly see that we are not working directly with the original array. If we were, the loop would go on forever since we're adding new items to the array. This confirms our assumption that 'foreach' operates on a <em>copy</em> of the array. 👍</p>
Test Case 2: Modifying Array Values within the Loop 🔄
<pre><code>foreach ($array as $key => $item) { $array[$key + 1] = $item + 2; echo "$item\n"; } print_r($array); /* Output in loop: 1 2 3 4 5 $array after loop: 1 3 4 5 6 7 */ </code></pre>
<p>Here, we continue to modify values within the loop. The result confirms our initial assumption that we are indeed working with a <em>copy</em> of the array. The changes made to the array are only reflected after the loop completes. 🔄</p>
The Array Pointer Puzzle 🧩
<p>Now things get a bit trickier. According to the PHP manual, when 'foreach' starts executing, the internal array pointer is reset to the first element. But wait, how can this be true if we're not working directly with the source array? Let's explore further. 🕵️♀️</p>
Test Case 3: Moving the Array Pointer 📌
<pre><code>// Move the array pointer on one to make sure it doesn't affect the loop var_dump(each($array)); foreach ($array as $item) { echo "$item\n"; } var_dump(each($array)); /* Output array(4) { [1]=> int(1) ["value"]=> int(1) [0]=> int(0) ["key"]=> int(0) } 1 2 3 4 5 bool(false) */ </code></pre>
<p>By moving the array pointer using the 'each' function before the loop starts, we can see that the pointer position affects the loop. It seems that even though we're not directly accessing the source array, the array pointer is linked to the source array. 📌</p>
The Unexpected Behavior 🤔
<p>The PHP manual warns that modifying the array pointer within a 'foreach' loop might lead to unexpected behavior. So, what happens if we do just that? Let's find out! 🧐</p>
Test Case 4: Modifying the Array Pointer with 'each' 🔄
<pre><code>foreach ($array as $key => $item) { echo "$item\n"; each($array); } /* Output: 1 2 3 4 5 */ </code></pre>
<p>Surprisingly, modifying the array pointer using the 'each' function within the loop doesn't seem to have any significant impact. The loop continues as expected, supporting our hypothesis that 'foreach' consistently works with a copy of the array. 🔄</p>
Test Case 5: Resetting the Array Pointer with 'reset' 🔁
<pre><code>foreach ($array as $key => $item) { echo "$item\n"; reset($array); } /* Output: 1 2 3 4 5 */ </code></pre>
<p>Similarly, resetting the array pointer with the 'reset' function doesn't introduce any unexpected behavior. The loop proceeds smoothly and outputs the expected results. 🔁</p>
<hr>
<p><strong>The Conclusion, Please? 📚</strong></p>
<p>So, after all our experiments and discussions, what have we learned about how 'foreach' actually works? Here's the scoop: 👇</p>
<ul> <li>'foreach' does work with a <em>copy</em> of the array, ensuring that modifications to the array don't affect the iteration process.</li> <li>However, the array pointer used by 'foreach' seems to be tied to the source array, which can explain some of the unexpected behaviors we observed.</li> <li>While modifying the array pointer within a 'foreach' loop doesn't have a significant impact, it's generally advised to avoid such actions to prevent any potential surprises.</li> </ul>
<p>That's it! You now have a better understanding of how 'foreach' works under the hood. Go ahead and iterate over your arrays with confidence! 🙌</p>
<p>If you have any more questions or insights about 'foreach' or any other PHP topics, don't hesitate to share them in the comments below. Let's keep the conversation going! 💬</p>
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
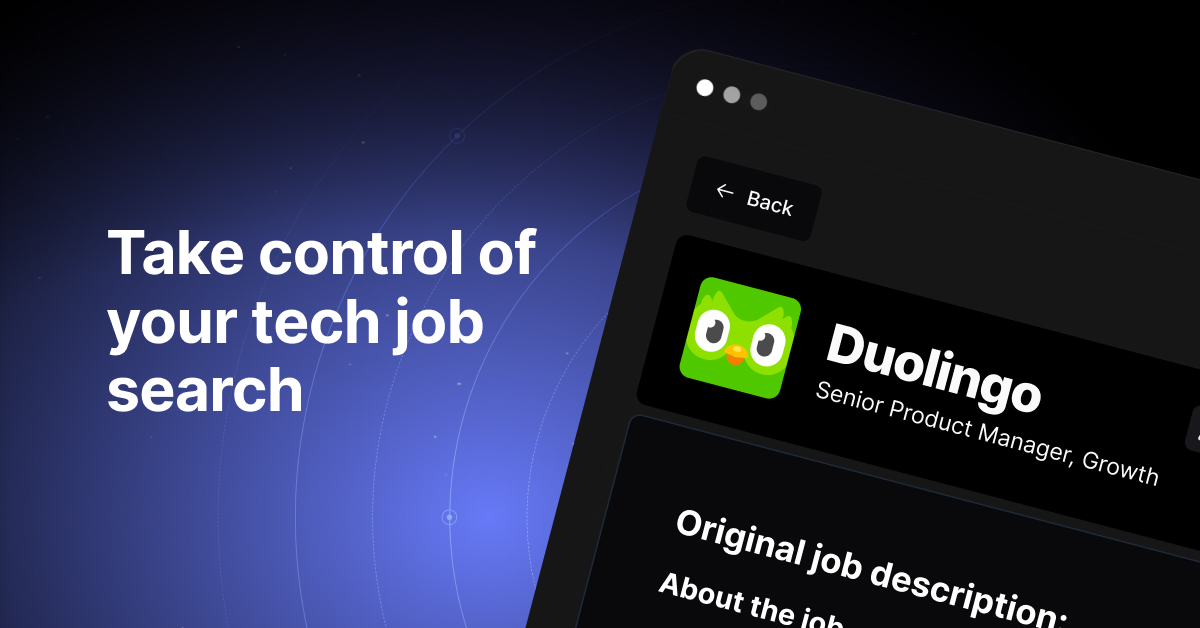